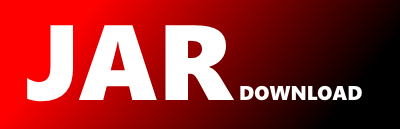
io.zeebe.test.broker.protocol.brokerapi.StubRequestHandler Maven / Gradle / Ivy
/*
* Copyright Camunda Services GmbH and/or licensed to Camunda Services GmbH under
* one or more contributor license agreements. See the NOTICE file distributed
* with this work for additional information regarding copyright ownership.
* Licensed under the Zeebe Community License 1.0. You may not use this file
* except in compliance with the Zeebe Community License 1.0.
*/
package io.zeebe.test.broker.protocol.brokerapi;
import io.zeebe.protocol.record.ExecuteCommandRequestDecoder;
import io.zeebe.protocol.record.MessageHeaderDecoder;
import io.zeebe.test.broker.protocol.MsgPackHelper;
import io.zeebe.transport.RequestHandler;
import io.zeebe.transport.ServerOutput;
import io.zeebe.transport.impl.ServerResponseImpl;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.CopyOnWriteArrayList;
import org.agrona.DirectBuffer;
import org.agrona.MutableDirectBuffer;
import org.agrona.concurrent.UnsafeBuffer;
public final class StubRequestHandler implements RequestHandler {
private final MessageHeaderDecoder headerDecoder = new MessageHeaderDecoder();
private final List> cmdRequestStubs = new ArrayList<>();
private final MsgPackHelper msgPackHelper;
// can also be used for verification
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy