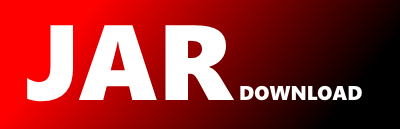
com.github.kristofa.brave.AutoValue_ClientTracer Maven / Gradle / Ivy
package com.github.kristofa.brave;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ClientTracer extends ClientTracer {
private final Recorder recorder;
private final CurrentSpan currentLocalSpan;
private final ServerSpanThreadBinder currentServerSpan;
private final ClientSpanThreadBinder currentSpan;
private final SpanFactory spanFactory;
AutoValue_ClientTracer(
Recorder recorder,
CurrentSpan currentLocalSpan,
ServerSpanThreadBinder currentServerSpan,
ClientSpanThreadBinder currentSpan,
SpanFactory spanFactory) {
if (recorder == null) {
throw new NullPointerException("Null recorder");
}
this.recorder = recorder;
if (currentLocalSpan == null) {
throw new NullPointerException("Null currentLocalSpan");
}
this.currentLocalSpan = currentLocalSpan;
if (currentServerSpan == null) {
throw new NullPointerException("Null currentServerSpan");
}
this.currentServerSpan = currentServerSpan;
if (currentSpan == null) {
throw new NullPointerException("Null currentSpan");
}
this.currentSpan = currentSpan;
if (spanFactory == null) {
throw new NullPointerException("Null spanFactory");
}
this.spanFactory = spanFactory;
}
@Override
Recorder recorder() {
return recorder;
}
@Override
CurrentSpan currentLocalSpan() {
return currentLocalSpan;
}
@Override
ServerSpanThreadBinder currentServerSpan() {
return currentServerSpan;
}
@Override
ClientSpanThreadBinder currentSpan() {
return currentSpan;
}
@Override
SpanFactory spanFactory() {
return spanFactory;
}
@Override
public String toString() {
return "ClientTracer{"
+ "recorder=" + recorder + ", "
+ "currentLocalSpan=" + currentLocalSpan + ", "
+ "currentServerSpan=" + currentServerSpan + ", "
+ "currentSpan=" + currentSpan + ", "
+ "spanFactory=" + spanFactory
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ClientTracer) {
ClientTracer that = (ClientTracer) o;
return (this.recorder.equals(that.recorder()))
&& (this.currentLocalSpan.equals(that.currentLocalSpan()))
&& (this.currentServerSpan.equals(that.currentServerSpan()))
&& (this.currentSpan.equals(that.currentSpan()))
&& (this.spanFactory.equals(that.spanFactory()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.recorder.hashCode();
h *= 1000003;
h ^= this.currentLocalSpan.hashCode();
h *= 1000003;
h ^= this.currentServerSpan.hashCode();
h *= 1000003;
h ^= this.currentSpan.hashCode();
h *= 1000003;
h ^= this.spanFactory.hashCode();
return h;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy