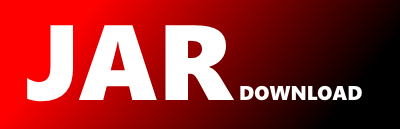
brave.http.HttpSampler Maven / Gradle / Ivy
/*
* Copyright 2013-2020 The OpenZipkin Authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package brave.http;
import brave.Span;
import brave.internal.Nullable;
import brave.sampler.SamplerFunction;
import brave.sampler.SamplerFunctions;
import static brave.http.HttpHandler.NULL_SENTINEL;
/**
* Decides whether to start a new trace based on http request properties such as path.
*
* Ex. Here's a sampler that only traces api requests
*
{@code
* httpTracingBuilder.serverSampler(new HttpSampler() {
* @Override public Boolean trySample(HttpAdapter adapter, Req request) {
* return adapter.path(request).startsWith("/api");
* }
* });
* }
*
* @see HttpRuleSampler
* @see SamplerFunction
* @deprecated Since 5.8, use {@code SamplerFunction}.
*/
@Deprecated
// abstract class as you can't lambda generic methods anyway. This lets us make helpers in the future
public abstract class HttpSampler implements SamplerFunction {
/** Ignores the request and uses the {@link brave.sampler.Sampler trace ID instead}. */
public static final HttpSampler TRACE_ID = new HttpSampler() {
@Override public Boolean trySample(HttpRequest request) {
return null;
}
@Override @Nullable public Boolean trySample(HttpAdapter adapter, Req request) {
return null;
}
@Override public String toString() {
return "DeferDecision";
}
};
/**
* Returns false to never start new traces for http requests. For example, you may wish to only
* capture traces if they originated from an inbound server request. Such a policy would filter
* out client requests made during bootstrap.
*/
public static final HttpSampler NEVER_SAMPLE = new HttpSampler() {
@Override public Boolean trySample(HttpRequest request) {
return false;
}
@Override public Boolean trySample(HttpAdapter adapter, Req request) {
return false;
}
@Override public String toString() {
return "NeverSample";
}
};
@Override @Nullable public Boolean trySample(HttpRequest request) {
if (request == null) return null;
Object unwrapped = request.unwrap();
if (unwrapped == null) unwrapped = NULL_SENTINEL; // Ensure adapter methods never see null
HttpAdapter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy