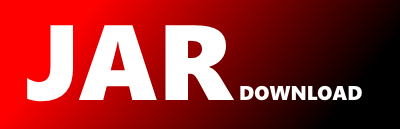
brave.propagation.AutoValue_TraceContext Maven / Gradle / Ivy
package brave.propagation;
import brave.internal.Nullable;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_TraceContext extends TraceContext {
private final boolean debug;
private final long traceIdHigh;
private final long traceId;
private final Long parentId;
private final Boolean sampled;
private final long spanId;
private final boolean shared;
private AutoValue_TraceContext(
boolean debug,
long traceIdHigh,
long traceId,
@Nullable Long parentId,
@Nullable Boolean sampled,
long spanId,
boolean shared) {
this.debug = debug;
this.traceIdHigh = traceIdHigh;
this.traceId = traceId;
this.parentId = parentId;
this.sampled = sampled;
this.spanId = spanId;
this.shared = shared;
}
@Override
public boolean debug() {
return debug;
}
@Override
public long traceIdHigh() {
return traceIdHigh;
}
@Override
public long traceId() {
return traceId;
}
@Nullable
@Override
public Long parentId() {
return parentId;
}
@Nullable
@Override
public Boolean sampled() {
return sampled;
}
@Override
public long spanId() {
return spanId;
}
@Override
public boolean shared() {
return shared;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof TraceContext) {
TraceContext that = (TraceContext) o;
return (this.debug == that.debug())
&& (this.traceIdHigh == that.traceIdHigh())
&& (this.traceId == that.traceId())
&& ((this.parentId == null) ? (that.parentId() == null) : this.parentId.equals(that.parentId()))
&& ((this.sampled == null) ? (that.sampled() == null) : this.sampled.equals(that.sampled()))
&& (this.spanId == that.spanId())
&& (this.shared == that.shared());
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.debug ? 1231 : 1237;
h *= 1000003;
h ^= (this.traceIdHigh >>> 32) ^ this.traceIdHigh;
h *= 1000003;
h ^= (this.traceId >>> 32) ^ this.traceId;
h *= 1000003;
h ^= (parentId == null) ? 0 : this.parentId.hashCode();
h *= 1000003;
h ^= (sampled == null) ? 0 : this.sampled.hashCode();
h *= 1000003;
h ^= (this.spanId >>> 32) ^ this.spanId;
h *= 1000003;
h ^= this.shared ? 1231 : 1237;
return h;
}
@Override
public TraceContext.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends TraceContext.Builder {
private Boolean debug;
private Long traceIdHigh;
private Long traceId;
private Long parentId;
private Boolean sampled;
private Long spanId;
private Boolean shared;
Builder() {
}
private Builder(TraceContext source) {
this.debug = source.debug();
this.traceIdHigh = source.traceIdHigh();
this.traceId = source.traceId();
this.parentId = source.parentId();
this.sampled = source.sampled();
this.spanId = source.spanId();
this.shared = source.shared();
}
@Override
public TraceContext.Builder debug(boolean debug) {
this.debug = debug;
return this;
}
@Override
public boolean debug() {
if (debug == null) {
throw new IllegalStateException("Property \"debug\" has not been set");
}
return debug;
}
@Override
public TraceContext.Builder traceIdHigh(long traceIdHigh) {
this.traceIdHigh = traceIdHigh;
return this;
}
@Override
public TraceContext.Builder traceId(long traceId) {
this.traceId = traceId;
return this;
}
@Override
public TraceContext.Builder parentId(@Nullable Long parentId) {
this.parentId = parentId;
return this;
}
@Override
public TraceContext.Builder sampled(@Nullable Boolean sampled) {
this.sampled = sampled;
return this;
}
@Override
@Nullable public Boolean sampled() {
return sampled;
}
@Override
public TraceContext.Builder spanId(long spanId) {
this.spanId = spanId;
return this;
}
@Override
public TraceContext.Builder shared(boolean shared) {
this.shared = shared;
return this;
}
@Override
public TraceContext build() {
String missing = "";
if (this.debug == null) {
missing += " debug";
}
if (this.traceIdHigh == null) {
missing += " traceIdHigh";
}
if (this.traceId == null) {
missing += " traceId";
}
if (this.spanId == null) {
missing += " spanId";
}
if (this.shared == null) {
missing += " shared";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_TraceContext(
this.debug,
this.traceIdHigh,
this.traceId,
this.parentId,
this.sampled,
this.spanId,
this.shared);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy