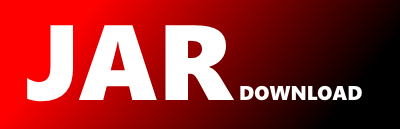
zipkin2.reporter.okhttp3.AutoValue_OkHttpSender Maven / Gradle / Ivy
package zipkin2.reporter.okhttp3;
import javax.annotation.processing.Generated;
import okhttp3.HttpUrl;
import okhttp3.OkHttpClient;
import zipkin2.codec.Encoding;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_OkHttpSender extends OkHttpSender {
private final Encoding encoding;
private final int messageMaxBytes;
private final HttpUrl endpoint;
private final OkHttpClient client;
private final int maxRequests;
private final boolean compressionEnabled;
private final RequestBodyMessageEncoder encoder;
private AutoValue_OkHttpSender(
Encoding encoding,
int messageMaxBytes,
HttpUrl endpoint,
OkHttpClient client,
int maxRequests,
boolean compressionEnabled,
RequestBodyMessageEncoder encoder) {
this.encoding = encoding;
this.messageMaxBytes = messageMaxBytes;
this.endpoint = endpoint;
this.client = client;
this.maxRequests = maxRequests;
this.compressionEnabled = compressionEnabled;
this.encoder = encoder;
}
@Override
public Encoding encoding() {
return encoding;
}
@Override
public int messageMaxBytes() {
return messageMaxBytes;
}
@Override
HttpUrl endpoint() {
return endpoint;
}
@Override
OkHttpClient client() {
return client;
}
@Override
int maxRequests() {
return maxRequests;
}
@Override
boolean compressionEnabled() {
return compressionEnabled;
}
@Override
RequestBodyMessageEncoder encoder() {
return encoder;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof OkHttpSender) {
OkHttpSender that = (OkHttpSender) o;
return (this.encoding.equals(that.encoding()))
&& (this.messageMaxBytes == that.messageMaxBytes())
&& (this.endpoint.equals(that.endpoint()))
&& (this.client.equals(that.client()))
&& (this.maxRequests == that.maxRequests())
&& (this.compressionEnabled == that.compressionEnabled())
&& (this.encoder.equals(that.encoder()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.encoding.hashCode();
h *= 1000003;
h ^= this.messageMaxBytes;
h *= 1000003;
h ^= this.endpoint.hashCode();
h *= 1000003;
h ^= this.client.hashCode();
h *= 1000003;
h ^= this.maxRequests;
h *= 1000003;
h ^= this.compressionEnabled ? 1231 : 1237;
h *= 1000003;
h ^= this.encoder.hashCode();
return h;
}
static final class Builder extends OkHttpSender.Builder {
private Encoding encoding;
private Integer messageMaxBytes;
private HttpUrl endpoint;
private OkHttpClient.Builder clientBuilder$;
private OkHttpClient client;
private Integer maxRequests;
private Boolean compressionEnabled;
private RequestBodyMessageEncoder encoder;
Builder() {
}
@Override
public OkHttpSender.Builder encoding(Encoding encoding) {
if (encoding == null) {
throw new NullPointerException("Null encoding");
}
this.encoding = encoding;
return this;
}
@Override
Encoding encoding() {
if (encoding == null) {
throw new IllegalStateException("Property \"encoding\" has not been set");
}
return encoding;
}
@Override
public OkHttpSender.Builder messageMaxBytes(int messageMaxBytes) {
this.messageMaxBytes = messageMaxBytes;
return this;
}
@Override
public OkHttpSender.Builder endpoint(HttpUrl endpoint) {
if (endpoint == null) {
throw new NullPointerException("Null endpoint");
}
this.endpoint = endpoint;
return this;
}
@Override
public OkHttpClient.Builder clientBuilder() {
if (clientBuilder$ == null) {
clientBuilder$ = new OkHttpClient.Builder();
}
return clientBuilder$;
}
@Override
public OkHttpSender.Builder maxRequests(int maxRequests) {
this.maxRequests = maxRequests;
return this;
}
@Override
int maxRequests() {
if (maxRequests == null) {
throw new IllegalStateException("Property \"maxRequests\" has not been set");
}
return maxRequests;
}
@Override
public OkHttpSender.Builder compressionEnabled(boolean compressionEnabled) {
this.compressionEnabled = compressionEnabled;
return this;
}
@Override
OkHttpSender.Builder encoder(RequestBodyMessageEncoder encoder) {
if (encoder == null) {
throw new NullPointerException("Null encoder");
}
this.encoder = encoder;
return this;
}
@Override
OkHttpSender autoBuild() {
if (clientBuilder$ != null) {
this.client = clientBuilder$.build();
} else if (this.client == null) {
OkHttpClient.Builder client$builder = new OkHttpClient.Builder();
this.client = client$builder.build();
}
String missing = "";
if (this.encoding == null) {
missing += " encoding";
}
if (this.messageMaxBytes == null) {
missing += " messageMaxBytes";
}
if (this.endpoint == null) {
missing += " endpoint";
}
if (this.maxRequests == null) {
missing += " maxRequests";
}
if (this.compressionEnabled == null) {
missing += " compressionEnabled";
}
if (this.encoder == null) {
missing += " encoder";
}
if (!missing.isEmpty()) {
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_OkHttpSender(
this.encoding,
this.messageMaxBytes,
this.endpoint,
this.client,
this.maxRequests,
this.compressionEnabled,
this.encoder);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy