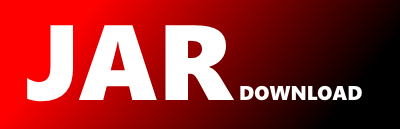
com.twitter.zipkin.thriftscala.Span.scala Maven / Gradle / Ivy
/**
* Generated by Scrooge
* version: 4.6.0
* rev: eb57ae5452b3a7a1fe18ba3ebf6bcc0721393d8c
* built at: 20160310-164326
*/
package com.twitter.zipkin.thriftscala
import com.twitter.scrooge.{
LazyTProtocol,
TFieldBlob, ThriftException, ThriftStruct, ThriftStructCodec3, ThriftStructFieldInfo,
ThriftStructMetaData, ThriftUtil}
import org.apache.thrift.protocol._
import org.apache.thrift.transport.{TMemoryBuffer, TTransport}
import java.nio.ByteBuffer
import java.util.Arrays
import scala.collection.immutable.{Map => immutable$Map}
import scala.collection.mutable.Builder
import scala.collection.mutable.{
ArrayBuffer => mutable$ArrayBuffer, Buffer => mutable$Buffer,
HashMap => mutable$HashMap, HashSet => mutable$HashSet}
import scala.collection.{Map, Set}
/**
* A trace is a series of spans (often RPC calls) which form a latency tree.
*
* Spans are usually created by instrumentation in RPC clients or servers, but
* can also represent in-process activity. Annotations in spans are similar to
* log statements, and are sometimes created directly by application developers
* to indicate events of interest, such as a cache miss.
*
* The root span is where parent_id = Nil; it usually has the longest duration
* in the trace.
*
* Span identifiers are packed into i64s, but should be treated opaquely.
* String encoding is fixed-width lower-hex, to avoid signed interpretation.
*/
object Span extends ThriftStructCodec3[Span] {
private val NoPassthroughFields = immutable$Map.empty[Short, TFieldBlob]
val Struct = new TStruct("Span")
val TraceIdField = new TField("trace_id", TType.I64, 1)
val TraceIdFieldManifest = implicitly[Manifest[Long]]
val NameField = new TField("name", TType.STRING, 3)
val NameFieldManifest = implicitly[Manifest[String]]
val IdField = new TField("id", TType.I64, 4)
val IdFieldManifest = implicitly[Manifest[Long]]
val ParentIdField = new TField("parent_id", TType.I64, 5)
val ParentIdFieldManifest = implicitly[Manifest[Long]]
val AnnotationsField = new TField("annotations", TType.LIST, 6)
val AnnotationsFieldManifest = implicitly[Manifest[Seq[com.twitter.zipkin.thriftscala.Annotation]]]
val BinaryAnnotationsField = new TField("binary_annotations", TType.LIST, 8)
val BinaryAnnotationsFieldManifest = implicitly[Manifest[Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation]]]
val DebugField = new TField("debug", TType.BOOL, 9)
val DebugFieldManifest = implicitly[Manifest[Boolean]]
val TimestampField = new TField("timestamp", TType.I64, 10)
val TimestampFieldManifest = implicitly[Manifest[Long]]
val DurationField = new TField("duration", TType.I64, 11)
val DurationFieldManifest = implicitly[Manifest[Long]]
/**
* Field information in declaration order.
*/
lazy val fieldInfos: scala.List[ThriftStructFieldInfo] = scala.List[ThriftStructFieldInfo](
new ThriftStructFieldInfo(
TraceIdField,
false,
false,
TraceIdFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
),
new ThriftStructFieldInfo(
NameField,
false,
false,
NameFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
),
new ThriftStructFieldInfo(
IdField,
false,
false,
IdFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
),
new ThriftStructFieldInfo(
ParentIdField,
true,
false,
ParentIdFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
),
new ThriftStructFieldInfo(
AnnotationsField,
false,
false,
AnnotationsFieldManifest,
_root_.scala.None,
_root_.scala.Some(implicitly[Manifest[com.twitter.zipkin.thriftscala.Annotation]]),
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
),
new ThriftStructFieldInfo(
BinaryAnnotationsField,
false,
false,
BinaryAnnotationsFieldManifest,
_root_.scala.None,
_root_.scala.Some(implicitly[Manifest[com.twitter.zipkin.thriftscala.BinaryAnnotation]]),
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
),
new ThriftStructFieldInfo(
DebugField,
false,
false,
DebugFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
),
new ThriftStructFieldInfo(
TimestampField,
true,
false,
TimestampFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
),
new ThriftStructFieldInfo(
DurationField,
true,
false,
DurationFieldManifest,
_root_.scala.None,
_root_.scala.None,
immutable$Map.empty[String, String],
immutable$Map.empty[String, String]
)
)
lazy val structAnnotations: immutable$Map[String, String] =
immutable$Map.empty[String, String]
/**
* Checks that all required fields are non-null.
*/
def validate(_item: Span): Unit = {
}
def withoutPassthroughFields(original: Span): Span =
new Immutable(
traceId =
{
val field = original.traceId
field
},
name =
{
val field = original.name
field
},
id =
{
val field = original.id
field
},
parentId =
{
val field = original.parentId
field.map { field =>
field
}
},
annotations =
{
val field = original.annotations
field.map { field =>
com.twitter.zipkin.thriftscala.Annotation.withoutPassthroughFields(field)
}
},
binaryAnnotations =
{
val field = original.binaryAnnotations
field.map { field =>
com.twitter.zipkin.thriftscala.BinaryAnnotation.withoutPassthroughFields(field)
}
},
debug =
{
val field = original.debug
field
},
timestamp =
{
val field = original.timestamp
field.map { field =>
field
}
},
duration =
{
val field = original.duration
field.map { field =>
field
}
}
)
override def encode(_item: Span, _oproto: TProtocol): Unit = {
_item.write(_oproto)
}
private[this] def lazyDecode(_iprot: LazyTProtocol): Span = {
var traceId: Long = 0L
var nameOffset: Int = -1
var id: Long = 0L
var parent_idOffset: Int = -1
var annotations: Seq[com.twitter.zipkin.thriftscala.Annotation] = Seq[com.twitter.zipkin.thriftscala.Annotation]()
var binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation]()
var debug: Boolean = false
var timestampOffset: Int = -1
var durationOffset: Int = -1
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
val _start_offset = _iprot.offset
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 1 =>
_field.`type` match {
case TType.I64 =>
traceId = readTraceIdValue(_iprot)
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'traceId' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 3 =>
_field.`type` match {
case TType.STRING =>
nameOffset = _iprot.offsetSkipString
case _actualType =>
val _expectedType = TType.STRING
throw new TProtocolException(
"Received wrong type for field 'name' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 4 =>
_field.`type` match {
case TType.I64 =>
id = readIdValue(_iprot)
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'id' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 5 =>
_field.`type` match {
case TType.I64 =>
parent_idOffset = _iprot.offsetSkipI64
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'parentId' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 6 =>
_field.`type` match {
case TType.LIST =>
annotations = readAnnotationsValue(_iprot)
case _actualType =>
val _expectedType = TType.LIST
throw new TProtocolException(
"Received wrong type for field 'annotations' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 8 =>
_field.`type` match {
case TType.LIST =>
binaryAnnotations = readBinaryAnnotationsValue(_iprot)
case _actualType =>
val _expectedType = TType.LIST
throw new TProtocolException(
"Received wrong type for field 'binaryAnnotations' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 9 =>
_field.`type` match {
case TType.BOOL =>
debug = readDebugValue(_iprot)
case _actualType =>
val _expectedType = TType.BOOL
throw new TProtocolException(
"Received wrong type for field 'debug' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 10 =>
_field.`type` match {
case TType.I64 =>
timestampOffset = _iprot.offsetSkipI64
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'timestamp' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 11 =>
_field.`type` match {
case TType.I64 =>
durationOffset = _iprot.offsetSkipI64
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'duration' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new LazyImmutable(
_iprot,
_iprot.buffer,
_start_offset,
_iprot.offset,
traceId,
nameOffset,
id,
parent_idOffset,
annotations,
binaryAnnotations,
debug,
timestampOffset,
durationOffset,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
override def decode(_iprot: TProtocol): Span =
_iprot match {
case i: LazyTProtocol => lazyDecode(i)
case i => eagerDecode(i)
}
private[this] def eagerDecode(_iprot: TProtocol): Span = {
var traceId: Long = 0L
var name: String = null
var id: Long = 0L
var parentId: _root_.scala.Option[Long] = _root_.scala.None
var annotations: Seq[com.twitter.zipkin.thriftscala.Annotation] = Seq[com.twitter.zipkin.thriftscala.Annotation]()
var binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation]()
var debug: Boolean = false
var timestamp: _root_.scala.Option[Long] = _root_.scala.None
var duration: _root_.scala.Option[Long] = _root_.scala.None
var _passthroughFields: Builder[(Short, TFieldBlob), immutable$Map[Short, TFieldBlob]] = null
var _done = false
_iprot.readStructBegin()
while (!_done) {
val _field = _iprot.readFieldBegin()
if (_field.`type` == TType.STOP) {
_done = true
} else {
_field.id match {
case 1 =>
_field.`type` match {
case TType.I64 =>
traceId = readTraceIdValue(_iprot)
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'traceId' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 3 =>
_field.`type` match {
case TType.STRING =>
name = readNameValue(_iprot)
case _actualType =>
val _expectedType = TType.STRING
throw new TProtocolException(
"Received wrong type for field 'name' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 4 =>
_field.`type` match {
case TType.I64 =>
id = readIdValue(_iprot)
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'id' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 5 =>
_field.`type` match {
case TType.I64 =>
parentId = _root_.scala.Some(readParentIdValue(_iprot))
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'parentId' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 6 =>
_field.`type` match {
case TType.LIST =>
annotations = readAnnotationsValue(_iprot)
case _actualType =>
val _expectedType = TType.LIST
throw new TProtocolException(
"Received wrong type for field 'annotations' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 8 =>
_field.`type` match {
case TType.LIST =>
binaryAnnotations = readBinaryAnnotationsValue(_iprot)
case _actualType =>
val _expectedType = TType.LIST
throw new TProtocolException(
"Received wrong type for field 'binaryAnnotations' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 9 =>
_field.`type` match {
case TType.BOOL =>
debug = readDebugValue(_iprot)
case _actualType =>
val _expectedType = TType.BOOL
throw new TProtocolException(
"Received wrong type for field 'debug' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 10 =>
_field.`type` match {
case TType.I64 =>
timestamp = _root_.scala.Some(readTimestampValue(_iprot))
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'timestamp' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case 11 =>
_field.`type` match {
case TType.I64 =>
duration = _root_.scala.Some(readDurationValue(_iprot))
case _actualType =>
val _expectedType = TType.I64
throw new TProtocolException(
"Received wrong type for field 'duration' (expected=%s, actual=%s).".format(
ttypeToString(_expectedType),
ttypeToString(_actualType)
)
)
}
case _ =>
if (_passthroughFields == null)
_passthroughFields = immutable$Map.newBuilder[Short, TFieldBlob]
_passthroughFields += (_field.id -> TFieldBlob.read(_field, _iprot))
}
_iprot.readFieldEnd()
}
}
_iprot.readStructEnd()
new Immutable(
traceId,
name,
id,
parentId,
annotations,
binaryAnnotations,
debug,
timestamp,
duration,
if (_passthroughFields == null)
NoPassthroughFields
else
_passthroughFields.result()
)
}
def apply(
traceId: Long,
name: String,
id: Long,
parentId: _root_.scala.Option[Long] = _root_.scala.None,
annotations: Seq[com.twitter.zipkin.thriftscala.Annotation] = Seq[com.twitter.zipkin.thriftscala.Annotation](),
binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation](),
debug: Boolean = false,
timestamp: _root_.scala.Option[Long] = _root_.scala.None,
duration: _root_.scala.Option[Long] = _root_.scala.None
): Span =
new Immutable(
traceId,
name,
id,
parentId,
annotations,
binaryAnnotations,
debug,
timestamp,
duration
)
def unapply(_item: Span): _root_.scala.Option[scala.Product9[Long, String, Long, Option[Long], Seq[com.twitter.zipkin.thriftscala.Annotation], Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation], Boolean, Option[Long], Option[Long]]] = _root_.scala.Some(_item)
@inline private def readTraceIdValue(_iprot: TProtocol): Long = {
_iprot.readI64()
}
@inline private def writeTraceIdField(traceId_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(TraceIdField)
writeTraceIdValue(traceId_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeTraceIdValue(traceId_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeI64(traceId_item)
}
@inline private def readNameValue(_iprot: TProtocol): String = {
_iprot.readString()
}
@inline private def writeNameField(name_item: String, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(NameField)
writeNameValue(name_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeNameValue(name_item: String, _oprot: TProtocol): Unit = {
_oprot.writeString(name_item)
}
@inline private def readIdValue(_iprot: TProtocol): Long = {
_iprot.readI64()
}
@inline private def writeIdField(id_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(IdField)
writeIdValue(id_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeIdValue(id_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeI64(id_item)
}
@inline private def readParentIdValue(_iprot: TProtocol): Long = {
_iprot.readI64()
}
@inline private def writeParentIdField(parentId_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(ParentIdField)
writeParentIdValue(parentId_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeParentIdValue(parentId_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeI64(parentId_item)
}
@inline private def readAnnotationsValue(_iprot: TProtocol): Seq[com.twitter.zipkin.thriftscala.Annotation] = {
val _list = _iprot.readListBegin()
if (_list.size == 0) {
_iprot.readListEnd()
Nil
} else {
val _rv = new mutable$ArrayBuffer[com.twitter.zipkin.thriftscala.Annotation](_list.size)
var _i = 0
while (_i < _list.size) {
_rv += {
com.twitter.zipkin.thriftscala.Annotation.decode(_iprot)
}
_i += 1
}
_iprot.readListEnd()
_rv
}
}
@inline private def writeAnnotationsField(annotations_item: Seq[com.twitter.zipkin.thriftscala.Annotation], _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(AnnotationsField)
writeAnnotationsValue(annotations_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeAnnotationsValue(annotations_item: Seq[com.twitter.zipkin.thriftscala.Annotation], _oprot: TProtocol): Unit = {
_oprot.writeListBegin(new TList(TType.STRUCT, annotations_item.size))
annotations_item match {
case _: IndexedSeq[_] =>
var _i = 0
val _size = annotations_item.size
while (_i < _size) {
val annotations_item_element = annotations_item(_i)
annotations_item_element.write(_oprot)
_i += 1
}
case _ =>
annotations_item.foreach { annotations_item_element =>
annotations_item_element.write(_oprot)
}
}
_oprot.writeListEnd()
}
@inline private def readBinaryAnnotationsValue(_iprot: TProtocol): Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = {
val _list = _iprot.readListBegin()
if (_list.size == 0) {
_iprot.readListEnd()
Nil
} else {
val _rv = new mutable$ArrayBuffer[com.twitter.zipkin.thriftscala.BinaryAnnotation](_list.size)
var _i = 0
while (_i < _list.size) {
_rv += {
com.twitter.zipkin.thriftscala.BinaryAnnotation.decode(_iprot)
}
_i += 1
}
_iprot.readListEnd()
_rv
}
}
@inline private def writeBinaryAnnotationsField(binaryAnnotations_item: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation], _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(BinaryAnnotationsField)
writeBinaryAnnotationsValue(binaryAnnotations_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeBinaryAnnotationsValue(binaryAnnotations_item: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation], _oprot: TProtocol): Unit = {
_oprot.writeListBegin(new TList(TType.STRUCT, binaryAnnotations_item.size))
binaryAnnotations_item match {
case _: IndexedSeq[_] =>
var _i = 0
val _size = binaryAnnotations_item.size
while (_i < _size) {
val binaryAnnotations_item_element = binaryAnnotations_item(_i)
binaryAnnotations_item_element.write(_oprot)
_i += 1
}
case _ =>
binaryAnnotations_item.foreach { binaryAnnotations_item_element =>
binaryAnnotations_item_element.write(_oprot)
}
}
_oprot.writeListEnd()
}
@inline private def readDebugValue(_iprot: TProtocol): Boolean = {
_iprot.readBool()
}
@inline private def writeDebugField(debug_item: Boolean, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(DebugField)
writeDebugValue(debug_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeDebugValue(debug_item: Boolean, _oprot: TProtocol): Unit = {
_oprot.writeBool(debug_item)
}
@inline private def readTimestampValue(_iprot: TProtocol): Long = {
_iprot.readI64()
}
@inline private def writeTimestampField(timestamp_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(TimestampField)
writeTimestampValue(timestamp_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeTimestampValue(timestamp_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeI64(timestamp_item)
}
@inline private def readDurationValue(_iprot: TProtocol): Long = {
_iprot.readI64()
}
@inline private def writeDurationField(duration_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeFieldBegin(DurationField)
writeDurationValue(duration_item, _oprot)
_oprot.writeFieldEnd()
}
@inline private def writeDurationValue(duration_item: Long, _oprot: TProtocol): Unit = {
_oprot.writeI64(duration_item)
}
object Immutable extends ThriftStructCodec3[Span] {
override def encode(_item: Span, _oproto: TProtocol): Unit = { _item.write(_oproto) }
override def decode(_iprot: TProtocol): Span = Span.decode(_iprot)
override lazy val metaData: ThriftStructMetaData[Span] = Span.metaData
}
/**
* The default read-only implementation of Span. You typically should not need to
* directly reference this class; instead, use the Span.apply method to construct
* new instances.
*/
class Immutable(
val traceId: Long,
val name: String,
val id: Long,
val parentId: _root_.scala.Option[Long],
val annotations: Seq[com.twitter.zipkin.thriftscala.Annotation],
val binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation],
val debug: Boolean,
val timestamp: _root_.scala.Option[Long],
val duration: _root_.scala.Option[Long],
override val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends Span {
def this(
traceId: Long,
name: String,
id: Long,
parentId: _root_.scala.Option[Long] = _root_.scala.None,
annotations: Seq[com.twitter.zipkin.thriftscala.Annotation] = Seq[com.twitter.zipkin.thriftscala.Annotation](),
binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation](),
debug: Boolean = false,
timestamp: _root_.scala.Option[Long] = _root_.scala.None,
duration: _root_.scala.Option[Long] = _root_.scala.None
) = this(
traceId,
name,
id,
parentId,
annotations,
binaryAnnotations,
debug,
timestamp,
duration,
Map.empty
)
}
/**
* This is another Immutable, this however keeps strings as lazy values that are lazily decoded from the backing
* array byte on read.
*/
private[this] class LazyImmutable(
_proto: LazyTProtocol,
_buf: Array[Byte],
_start_offset: Int,
_end_offset: Int,
val traceId: Long,
nameOffset: Int,
val id: Long,
parent_idOffset: Int,
val annotations: Seq[com.twitter.zipkin.thriftscala.Annotation],
val binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation],
val debug: Boolean,
timestampOffset: Int,
durationOffset: Int,
override val _passthroughFields: immutable$Map[Short, TFieldBlob])
extends Span {
override def write(_oprot: TProtocol): Unit = {
_oprot match {
case i: LazyTProtocol => i.writeRaw(_buf, _start_offset, _end_offset - _start_offset)
case _ => super.write(_oprot)
}
}
lazy val name: String =
if (nameOffset == -1)
null
else {
_proto.decodeString(_buf, nameOffset)
}
lazy val parentId: _root_.scala.Option[Long] =
if (parent_idOffset == -1)
None
else {
Some(_proto.decodeI64(_buf, parent_idOffset))
}
lazy val timestamp: _root_.scala.Option[Long] =
if (timestampOffset == -1)
None
else {
Some(_proto.decodeI64(_buf, timestampOffset))
}
lazy val duration: _root_.scala.Option[Long] =
if (durationOffset == -1)
None
else {
Some(_proto.decodeI64(_buf, durationOffset))
}
/**
* Override the super hash code to make it a lazy val rather than def.
*
* Calculating the hash code can be expensive, caching it where possible
* can provide significant performance wins. (Key in a hash map for instance)
* Usually not safe since the normal constructor will accept a mutable map or
* set as an arg
* Here however we control how the class is generated from serialized data.
* With the class private and the contract that we throw away our mutable references
* having the hash code lazy here is safe.
*/
override lazy val hashCode = super.hashCode
}
/**
* This Proxy trait allows you to extend the Span trait with additional state or
* behavior and implement the read-only methods from Span using an underlying
* instance.
*/
trait Proxy extends Span {
protected def _underlying_Span: Span
override def traceId: Long = _underlying_Span.traceId
override def name: String = _underlying_Span.name
override def id: Long = _underlying_Span.id
override def parentId: _root_.scala.Option[Long] = _underlying_Span.parentId
override def annotations: Seq[com.twitter.zipkin.thriftscala.Annotation] = _underlying_Span.annotations
override def binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = _underlying_Span.binaryAnnotations
override def debug: Boolean = _underlying_Span.debug
override def timestamp: _root_.scala.Option[Long] = _underlying_Span.timestamp
override def duration: _root_.scala.Option[Long] = _underlying_Span.duration
override def _passthroughFields = _underlying_Span._passthroughFields
}
}
trait Span
extends ThriftStruct
with scala.Product9[Long, String, Long, Option[Long], Seq[com.twitter.zipkin.thriftscala.Annotation], Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation], Boolean, Option[Long], Option[Long]]
with java.io.Serializable
{
import Span._
def traceId: Long
def name: String
def id: Long
def parentId: _root_.scala.Option[Long]
def annotations: Seq[com.twitter.zipkin.thriftscala.Annotation]
def binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation]
def debug: Boolean
def timestamp: _root_.scala.Option[Long]
def duration: _root_.scala.Option[Long]
def _passthroughFields: immutable$Map[Short, TFieldBlob] = immutable$Map.empty
def _1 = traceId
def _2 = name
def _3 = id
def _4 = parentId
def _5 = annotations
def _6 = binaryAnnotations
def _7 = debug
def _8 = timestamp
def _9 = duration
/**
* Gets a field value encoded as a binary blob using TCompactProtocol. If the specified field
* is present in the passthrough map, that value is returned. Otherwise, if the specified field
* is known and not optional and set to None, then the field is serialized and returned.
*/
def getFieldBlob(_fieldId: Short): _root_.scala.Option[TFieldBlob] = {
lazy val _buff = new TMemoryBuffer(32)
lazy val _oprot = new TCompactProtocol(_buff)
_passthroughFields.get(_fieldId) match {
case blob: _root_.scala.Some[TFieldBlob] => blob
case _root_.scala.None => {
val _fieldOpt: _root_.scala.Option[TField] =
_fieldId match {
case 1 =>
if (true) {
writeTraceIdValue(traceId, _oprot)
_root_.scala.Some(Span.TraceIdField)
} else {
_root_.scala.None
}
case 3 =>
if (name ne null) {
writeNameValue(name, _oprot)
_root_.scala.Some(Span.NameField)
} else {
_root_.scala.None
}
case 4 =>
if (true) {
writeIdValue(id, _oprot)
_root_.scala.Some(Span.IdField)
} else {
_root_.scala.None
}
case 5 =>
if (parentId.isDefined) {
writeParentIdValue(parentId.get, _oprot)
_root_.scala.Some(Span.ParentIdField)
} else {
_root_.scala.None
}
case 6 =>
if (annotations ne null) {
writeAnnotationsValue(annotations, _oprot)
_root_.scala.Some(Span.AnnotationsField)
} else {
_root_.scala.None
}
case 8 =>
if (binaryAnnotations ne null) {
writeBinaryAnnotationsValue(binaryAnnotations, _oprot)
_root_.scala.Some(Span.BinaryAnnotationsField)
} else {
_root_.scala.None
}
case 9 =>
if (true) {
writeDebugValue(debug, _oprot)
_root_.scala.Some(Span.DebugField)
} else {
_root_.scala.None
}
case 10 =>
if (timestamp.isDefined) {
writeTimestampValue(timestamp.get, _oprot)
_root_.scala.Some(Span.TimestampField)
} else {
_root_.scala.None
}
case 11 =>
if (duration.isDefined) {
writeDurationValue(duration.get, _oprot)
_root_.scala.Some(Span.DurationField)
} else {
_root_.scala.None
}
case _ => _root_.scala.None
}
_fieldOpt match {
case _root_.scala.Some(_field) =>
val _data = Arrays.copyOfRange(_buff.getArray, 0, _buff.length)
_root_.scala.Some(TFieldBlob(_field, _data))
case _root_.scala.None =>
_root_.scala.None
}
}
}
}
/**
* Collects TCompactProtocol-encoded field values according to `getFieldBlob` into a map.
*/
def getFieldBlobs(ids: TraversableOnce[Short]): immutable$Map[Short, TFieldBlob] =
(ids flatMap { id => getFieldBlob(id) map { id -> _ } }).toMap
/**
* Sets a field using a TCompactProtocol-encoded binary blob. If the field is a known
* field, the blob is decoded and the field is set to the decoded value. If the field
* is unknown and passthrough fields are enabled, then the blob will be stored in
* _passthroughFields.
*/
def setField(_blob: TFieldBlob): Span = {
var traceId: Long = this.traceId
var name: String = this.name
var id: Long = this.id
var parentId: _root_.scala.Option[Long] = this.parentId
var annotations: Seq[com.twitter.zipkin.thriftscala.Annotation] = this.annotations
var binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = this.binaryAnnotations
var debug: Boolean = this.debug
var timestamp: _root_.scala.Option[Long] = this.timestamp
var duration: _root_.scala.Option[Long] = this.duration
var _passthroughFields = this._passthroughFields
_blob.id match {
case 1 =>
traceId = readTraceIdValue(_blob.read)
case 3 =>
name = readNameValue(_blob.read)
case 4 =>
id = readIdValue(_blob.read)
case 5 =>
parentId = _root_.scala.Some(readParentIdValue(_blob.read))
case 6 =>
annotations = readAnnotationsValue(_blob.read)
case 8 =>
binaryAnnotations = readBinaryAnnotationsValue(_blob.read)
case 9 =>
debug = readDebugValue(_blob.read)
case 10 =>
timestamp = _root_.scala.Some(readTimestampValue(_blob.read))
case 11 =>
duration = _root_.scala.Some(readDurationValue(_blob.read))
case _ => _passthroughFields += (_blob.id -> _blob)
}
new Immutable(
traceId,
name,
id,
parentId,
annotations,
binaryAnnotations,
debug,
timestamp,
duration,
_passthroughFields
)
}
/**
* If the specified field is optional, it is set to None. Otherwise, if the field is
* known, it is reverted to its default value; if the field is unknown, it is removed
* from the passthroughFields map, if present.
*/
def unsetField(_fieldId: Short): Span = {
var traceId: Long = this.traceId
var name: String = this.name
var id: Long = this.id
var parentId: _root_.scala.Option[Long] = this.parentId
var annotations: Seq[com.twitter.zipkin.thriftscala.Annotation] = this.annotations
var binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = this.binaryAnnotations
var debug: Boolean = this.debug
var timestamp: _root_.scala.Option[Long] = this.timestamp
var duration: _root_.scala.Option[Long] = this.duration
_fieldId match {
case 1 =>
traceId = 0L
case 3 =>
name = null
case 4 =>
id = 0L
case 5 =>
parentId = _root_.scala.None
case 6 =>
annotations = Seq[com.twitter.zipkin.thriftscala.Annotation]()
case 8 =>
binaryAnnotations = Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation]()
case 9 =>
debug = false
case 10 =>
timestamp = _root_.scala.None
case 11 =>
duration = _root_.scala.None
case _ =>
}
new Immutable(
traceId,
name,
id,
parentId,
annotations,
binaryAnnotations,
debug,
timestamp,
duration,
_passthroughFields - _fieldId
)
}
/**
* If the specified field is optional, it is set to None. Otherwise, if the field is
* known, it is reverted to its default value; if the field is unknown, it is removed
* from the passthroughFields map, if present.
*/
def unsetTraceId: Span = unsetField(1)
def unsetName: Span = unsetField(3)
def unsetId: Span = unsetField(4)
def unsetParentId: Span = unsetField(5)
def unsetAnnotations: Span = unsetField(6)
def unsetBinaryAnnotations: Span = unsetField(8)
def unsetDebug: Span = unsetField(9)
def unsetTimestamp: Span = unsetField(10)
def unsetDuration: Span = unsetField(11)
override def write(_oprot: TProtocol): Unit = {
Span.validate(this)
_oprot.writeStructBegin(Struct)
writeTraceIdField(traceId, _oprot)
if (name ne null) writeNameField(name, _oprot)
writeIdField(id, _oprot)
if (parentId.isDefined) writeParentIdField(parentId.get, _oprot)
if (annotations ne null) writeAnnotationsField(annotations, _oprot)
if (binaryAnnotations ne null) writeBinaryAnnotationsField(binaryAnnotations, _oprot)
writeDebugField(debug, _oprot)
if (timestamp.isDefined) writeTimestampField(timestamp.get, _oprot)
if (duration.isDefined) writeDurationField(duration.get, _oprot)
if (_passthroughFields.nonEmpty) {
_passthroughFields.values.foreach { _.write(_oprot) }
}
_oprot.writeFieldStop()
_oprot.writeStructEnd()
}
def copy(
traceId: Long = this.traceId,
name: String = this.name,
id: Long = this.id,
parentId: _root_.scala.Option[Long] = this.parentId,
annotations: Seq[com.twitter.zipkin.thriftscala.Annotation] = this.annotations,
binaryAnnotations: Seq[com.twitter.zipkin.thriftscala.BinaryAnnotation] = this.binaryAnnotations,
debug: Boolean = this.debug,
timestamp: _root_.scala.Option[Long] = this.timestamp,
duration: _root_.scala.Option[Long] = this.duration,
_passthroughFields: immutable$Map[Short, TFieldBlob] = this._passthroughFields
): Span =
new Immutable(
traceId,
name,
id,
parentId,
annotations,
binaryAnnotations,
debug,
timestamp,
duration,
_passthroughFields
)
override def canEqual(other: Any): Boolean = other.isInstanceOf[Span]
override def equals(other: Any): Boolean =
canEqual(other) &&
_root_.scala.runtime.ScalaRunTime._equals(this, other) &&
_passthroughFields == other.asInstanceOf[Span]._passthroughFields
override def hashCode: Int = _root_.scala.runtime.ScalaRunTime._hashCode(this)
override def toString: String = _root_.scala.runtime.ScalaRunTime._toString(this)
override def productArity: Int = 9
override def productElement(n: Int): Any = n match {
case 0 => this.traceId
case 1 => this.name
case 2 => this.id
case 3 => this.parentId
case 4 => this.annotations
case 5 => this.binaryAnnotations
case 6 => this.debug
case 7 => this.timestamp
case 8 => this.duration
case _ => throw new IndexOutOfBoundsException(n.toString)
}
override def productPrefix: String = "Span"
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy