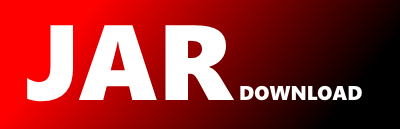
com.google.protobuf.FieldOptions Maven / Gradle / Ivy
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: google/protobuf/descriptor.proto
package com.google.protobuf;
import com.squareup.wire.EnumAdapter;
import com.squareup.wire.FieldEncoding;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoAdapter;
import com.squareup.wire.ProtoReader;
import com.squareup.wire.ProtoWriter;
import com.squareup.wire.ReverseProtoWriter;
import com.squareup.wire.Syntax;
import com.squareup.wire.WireEnum;
import com.squareup.wire.WireField;
import com.squareup.wire.internal.Internal;
import java.io.IOException;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.List;
import okio.ByteString;
public final class FieldOptions extends Message {
public static final ProtoAdapter ADAPTER = new ProtoAdapter_FieldOptions();
private static final long serialVersionUID = 0L;
public static final CType DEFAULT_CTYPE = CType.STRING;
public static final Boolean DEFAULT_PACKED = false;
public static final JSType DEFAULT_JSTYPE = JSType.JS_NORMAL;
public static final Boolean DEFAULT_LAZY = false;
public static final Boolean DEFAULT_UNVERIFIED_LAZY = false;
public static final Boolean DEFAULT_DEPRECATED = false;
public static final Boolean DEFAULT_WEAK = false;
public static final Boolean DEFAULT_DEBUG_REDACT = false;
public static final OptionRetention DEFAULT_RETENTION = OptionRetention.RETENTION_UNKNOWN;
public static final String DEFAULT_SINCE = "";
public static final String DEFAULT_UNTIL = "";
public static final Boolean DEFAULT_USE_ARRAY = false;
/**
* The ctype option instructs the C++ code generator to use a different
* representation of the field than it normally would. See the specific
* options below. This option is only implemented to support use of
* [ctype=CORD] and [ctype=STRING] (the default) on non-repeated fields of
* type "bytes" in the open source release -- sorry, we'll try to include
* other types in a future version!
*/
@WireField(
tag = 1,
adapter = "com.google.protobuf.FieldOptions$CType#ADAPTER"
)
public final CType ctype;
/**
* The packed option can be enabled for repeated primitive fields to enable
* a more efficient representation on the wire. Rather than repeatedly
* writing the tag and type for each element, the entire array is encoded as
* a single length-delimited blob. In proto3, only explicit setting it to
* false will avoid using packed encoding. This option is prohibited in
* Editions, but the `repeated_field_encoding` feature can be used to control
* the behavior.
*/
@WireField(
tag = 2,
adapter = "com.squareup.wire.ProtoAdapter#BOOL"
)
public final Boolean packed;
/**
* The jstype option determines the JavaScript type used for values of the
* field. The option is permitted only for 64 bit integral and fixed types
* (int64, uint64, sint64, fixed64, sfixed64). A field with jstype JS_STRING
* is represented as JavaScript string, which avoids loss of precision that
* can happen when a large value is converted to a floating point JavaScript.
* Specifying JS_NUMBER for the jstype causes the generated JavaScript code to
* use the JavaScript "number" type. The behavior of the default option
* JS_NORMAL is implementation dependent.
*
* This option is an enum to permit additional types to be added, e.g.
* goog.math.Integer.
*/
@WireField(
tag = 6,
adapter = "com.google.protobuf.FieldOptions$JSType#ADAPTER"
)
public final JSType jstype;
/**
* Should this field be parsed lazily? Lazy applies only to message-type
* fields. It means that when the outer message is initially parsed, the
* inner message's contents will not be parsed but instead stored in encoded
* form. The inner message will actually be parsed when it is first accessed.
*
* This is only a hint. Implementations are free to choose whether to use
* eager or lazy parsing regardless of the value of this option. However,
* setting this option true suggests that the protocol author believes that
* using lazy parsing on this field is worth the additional bookkeeping
* overhead typically needed to implement it.
*
* This option does not affect the public interface of any generated code;
* all method signatures remain the same. Furthermore, thread-safety of the
* interface is not affected by this option; const methods remain safe to
* call from multiple threads concurrently, while non-const methods continue
* to require exclusive access.
*
* Note that implementations may choose not to check required fields within
* a lazy sub-message. That is, calling IsInitialized() on the outer message
* may return true even if the inner message has missing required fields.
* This is necessary because otherwise the inner message would have to be
* parsed in order to perform the check, defeating the purpose of lazy
* parsing. An implementation which chooses not to check required fields
* must be consistent about it. That is, for any particular sub-message, the
* implementation must either *always* check its required fields, or *never*
* check its required fields, regardless of whether or not the message has
* been parsed.
*
* As of May 2022, lazy verifies the contents of the byte stream during
* parsing. An invalid byte stream will cause the overall parsing to fail.
*/
@WireField(
tag = 5,
adapter = "com.squareup.wire.ProtoAdapter#BOOL"
)
public final Boolean lazy;
/**
* unverified_lazy does no correctness checks on the byte stream. This should
* only be used where lazy with verification is prohibitive for performance
* reasons.
*/
@WireField(
tag = 15,
adapter = "com.squareup.wire.ProtoAdapter#BOOL"
)
public final Boolean unverified_lazy;
/**
* Is this field deprecated?
* Depending on the target platform, this can emit Deprecated annotations
* for accessors, or it will be completely ignored; in the very least, this
* is a formalization for deprecating fields.
*/
@WireField(
tag = 3,
adapter = "com.squareup.wire.ProtoAdapter#BOOL"
)
public final Boolean deprecated;
/**
* For Google-internal migration only. Do not use.
*/
@WireField(
tag = 10,
adapter = "com.squareup.wire.ProtoAdapter#BOOL"
)
public final Boolean weak;
/**
* Indicate that the field value should not be printed out when using debug
* formats, e.g. when the field contains sensitive credentials.
*/
@WireField(
tag = 16,
adapter = "com.squareup.wire.ProtoAdapter#BOOL"
)
public final Boolean debug_redact;
@WireField(
tag = 17,
adapter = "com.google.protobuf.FieldOptions$OptionRetention#ADAPTER"
)
public final OptionRetention retention;
@WireField(
tag = 19,
adapter = "com.google.protobuf.FieldOptions$OptionTargetType#ADAPTER",
label = WireField.Label.REPEATED
)
public final List targets;
@WireField(
tag = 20,
adapter = "com.google.protobuf.FieldOptions$EditionDefault#ADAPTER",
label = WireField.Label.REPEATED
)
public final List edition_defaults;
/**
* Any features defined in the specific edition.
*/
@WireField(
tag = 21,
adapter = "com.google.protobuf.FeatureSet#ADAPTER"
)
public final FeatureSet features;
/**
* The parser stores options it doesn't recognize here. See above.
*/
@WireField(
tag = 999,
adapter = "com.google.protobuf.UninterpretedOption#ADAPTER",
label = WireField.Label.REPEATED
)
public final List uninterpreted_option;
/**
* Annotates a field that starts to be available with a specified version.
*
* The value is the earliest version in which the annotated field is retained. It is pruned from
* all preceding versions.
* Extension source: wire/extensions.proto
*/
@WireField(
tag = 1076,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String since;
/**
* Annotates a field that ceases to be available with a specified version.
*
* The value is the version that drops the annotated field. It is not available in that version or
* any subsequent version.
* Extension source: wire/extensions.proto
*/
@WireField(
tag = 1077,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String until;
/**
* Uses an Array at runtime to hold the values, to avoid autoboxing on the JVM. Will use the appropriate
* array type, for example `repeated float` would be represented as a FloatArray.
* Extension source: wire/extensions.proto
*/
@WireField(
tag = 1185,
adapter = "com.squareup.wire.ProtoAdapter#BOOL"
)
public final Boolean use_array;
public FieldOptions(Builder builder, ByteString unknownFields) {
super(ADAPTER, unknownFields);
this.ctype = builder.ctype;
this.packed = builder.packed;
this.jstype = builder.jstype;
this.lazy = builder.lazy;
this.unverified_lazy = builder.unverified_lazy;
this.deprecated = builder.deprecated;
this.weak = builder.weak;
this.debug_redact = builder.debug_redact;
this.retention = builder.retention;
this.targets = Internal.immutableCopyOf("targets", builder.targets);
this.edition_defaults = Internal.immutableCopyOf("edition_defaults", builder.edition_defaults);
this.features = builder.features;
this.uninterpreted_option = Internal.immutableCopyOf("uninterpreted_option", builder.uninterpreted_option);
this.since = builder.since;
this.until = builder.until;
this.use_array = builder.use_array;
}
@Override
public Builder newBuilder() {
Builder builder = new Builder();
builder.ctype = ctype;
builder.packed = packed;
builder.jstype = jstype;
builder.lazy = lazy;
builder.unverified_lazy = unverified_lazy;
builder.deprecated = deprecated;
builder.weak = weak;
builder.debug_redact = debug_redact;
builder.retention = retention;
builder.targets = Internal.copyOf(targets);
builder.edition_defaults = Internal.copyOf(edition_defaults);
builder.features = features;
builder.uninterpreted_option = Internal.copyOf(uninterpreted_option);
builder.since = since;
builder.until = until;
builder.use_array = use_array;
builder.addUnknownFields(unknownFields());
return builder;
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof FieldOptions)) return false;
FieldOptions o = (FieldOptions) other;
return unknownFields().equals(o.unknownFields())
&& Internal.equals(ctype, o.ctype)
&& Internal.equals(packed, o.packed)
&& Internal.equals(jstype, o.jstype)
&& Internal.equals(lazy, o.lazy)
&& Internal.equals(unverified_lazy, o.unverified_lazy)
&& Internal.equals(deprecated, o.deprecated)
&& Internal.equals(weak, o.weak)
&& Internal.equals(debug_redact, o.debug_redact)
&& Internal.equals(retention, o.retention)
&& targets.equals(o.targets)
&& edition_defaults.equals(o.edition_defaults)
&& Internal.equals(features, o.features)
&& uninterpreted_option.equals(o.uninterpreted_option)
&& Internal.equals(since, o.since)
&& Internal.equals(until, o.until)
&& Internal.equals(use_array, o.use_array);
}
@Override
public int hashCode() {
int result = super.hashCode;
if (result == 0) {
result = unknownFields().hashCode();
result = result * 37 + (ctype != null ? ctype.hashCode() : 0);
result = result * 37 + (packed != null ? packed.hashCode() : 0);
result = result * 37 + (jstype != null ? jstype.hashCode() : 0);
result = result * 37 + (lazy != null ? lazy.hashCode() : 0);
result = result * 37 + (unverified_lazy != null ? unverified_lazy.hashCode() : 0);
result = result * 37 + (deprecated != null ? deprecated.hashCode() : 0);
result = result * 37 + (weak != null ? weak.hashCode() : 0);
result = result * 37 + (debug_redact != null ? debug_redact.hashCode() : 0);
result = result * 37 + (retention != null ? retention.hashCode() : 0);
result = result * 37 + targets.hashCode();
result = result * 37 + edition_defaults.hashCode();
result = result * 37 + (features != null ? features.hashCode() : 0);
result = result * 37 + uninterpreted_option.hashCode();
result = result * 37 + (since != null ? since.hashCode() : 0);
result = result * 37 + (until != null ? until.hashCode() : 0);
result = result * 37 + (use_array != null ? use_array.hashCode() : 0);
super.hashCode = result;
}
return result;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
if (ctype != null) builder.append(", ctype=").append(ctype);
if (packed != null) builder.append(", packed=").append(packed);
if (jstype != null) builder.append(", jstype=").append(jstype);
if (lazy != null) builder.append(", lazy=").append(lazy);
if (unverified_lazy != null) builder.append(", unverified_lazy=").append(unverified_lazy);
if (deprecated != null) builder.append(", deprecated=").append(deprecated);
if (weak != null) builder.append(", weak=").append(weak);
if (debug_redact != null) builder.append(", debug_redact=").append(debug_redact);
if (retention != null) builder.append(", retention=").append(retention);
if (!targets.isEmpty()) builder.append(", targets=").append(targets);
if (!edition_defaults.isEmpty()) builder.append(", edition_defaults=").append(edition_defaults);
if (features != null) builder.append(", features=").append(features);
if (!uninterpreted_option.isEmpty()) builder.append(", uninterpreted_option=").append(uninterpreted_option);
if (since != null) builder.append(", since=").append(Internal.sanitize(since));
if (until != null) builder.append(", until=").append(Internal.sanitize(until));
if (use_array != null) builder.append(", use_array=").append(use_array);
return builder.replace(0, 2, "FieldOptions{").append('}').toString();
}
public static final class Builder extends Message.Builder {
public CType ctype;
public Boolean packed;
public JSType jstype;
public Boolean lazy;
public Boolean unverified_lazy;
public Boolean deprecated;
public Boolean weak;
public Boolean debug_redact;
public OptionRetention retention;
public List targets;
public List edition_defaults;
public FeatureSet features;
public List uninterpreted_option;
public String since;
public String until;
public Boolean use_array;
public Builder() {
targets = Internal.newMutableList();
edition_defaults = Internal.newMutableList();
uninterpreted_option = Internal.newMutableList();
}
/**
* The ctype option instructs the C++ code generator to use a different
* representation of the field than it normally would. See the specific
* options below. This option is only implemented to support use of
* [ctype=CORD] and [ctype=STRING] (the default) on non-repeated fields of
* type "bytes" in the open source release -- sorry, we'll try to include
* other types in a future version!
*/
public Builder ctype(CType ctype) {
this.ctype = ctype;
return this;
}
/**
* The packed option can be enabled for repeated primitive fields to enable
* a more efficient representation on the wire. Rather than repeatedly
* writing the tag and type for each element, the entire array is encoded as
* a single length-delimited blob. In proto3, only explicit setting it to
* false will avoid using packed encoding. This option is prohibited in
* Editions, but the `repeated_field_encoding` feature can be used to control
* the behavior.
*/
public Builder packed(Boolean packed) {
this.packed = packed;
return this;
}
/**
* The jstype option determines the JavaScript type used for values of the
* field. The option is permitted only for 64 bit integral and fixed types
* (int64, uint64, sint64, fixed64, sfixed64). A field with jstype JS_STRING
* is represented as JavaScript string, which avoids loss of precision that
* can happen when a large value is converted to a floating point JavaScript.
* Specifying JS_NUMBER for the jstype causes the generated JavaScript code to
* use the JavaScript "number" type. The behavior of the default option
* JS_NORMAL is implementation dependent.
*
* This option is an enum to permit additional types to be added, e.g.
* goog.math.Integer.
*/
public Builder jstype(JSType jstype) {
this.jstype = jstype;
return this;
}
/**
* Should this field be parsed lazily? Lazy applies only to message-type
* fields. It means that when the outer message is initially parsed, the
* inner message's contents will not be parsed but instead stored in encoded
* form. The inner message will actually be parsed when it is first accessed.
*
* This is only a hint. Implementations are free to choose whether to use
* eager or lazy parsing regardless of the value of this option. However,
* setting this option true suggests that the protocol author believes that
* using lazy parsing on this field is worth the additional bookkeeping
* overhead typically needed to implement it.
*
* This option does not affect the public interface of any generated code;
* all method signatures remain the same. Furthermore, thread-safety of the
* interface is not affected by this option; const methods remain safe to
* call from multiple threads concurrently, while non-const methods continue
* to require exclusive access.
*
* Note that implementations may choose not to check required fields within
* a lazy sub-message. That is, calling IsInitialized() on the outer message
* may return true even if the inner message has missing required fields.
* This is necessary because otherwise the inner message would have to be
* parsed in order to perform the check, defeating the purpose of lazy
* parsing. An implementation which chooses not to check required fields
* must be consistent about it. That is, for any particular sub-message, the
* implementation must either *always* check its required fields, or *never*
* check its required fields, regardless of whether or not the message has
* been parsed.
*
* As of May 2022, lazy verifies the contents of the byte stream during
* parsing. An invalid byte stream will cause the overall parsing to fail.
*/
public Builder lazy(Boolean lazy) {
this.lazy = lazy;
return this;
}
/**
* unverified_lazy does no correctness checks on the byte stream. This should
* only be used where lazy with verification is prohibitive for performance
* reasons.
*/
public Builder unverified_lazy(Boolean unverified_lazy) {
this.unverified_lazy = unverified_lazy;
return this;
}
/**
* Is this field deprecated?
* Depending on the target platform, this can emit Deprecated annotations
* for accessors, or it will be completely ignored; in the very least, this
* is a formalization for deprecating fields.
*/
public Builder deprecated(Boolean deprecated) {
this.deprecated = deprecated;
return this;
}
/**
* For Google-internal migration only. Do not use.
*/
public Builder weak(Boolean weak) {
this.weak = weak;
return this;
}
/**
* Indicate that the field value should not be printed out when using debug
* formats, e.g. when the field contains sensitive credentials.
*/
public Builder debug_redact(Boolean debug_redact) {
this.debug_redact = debug_redact;
return this;
}
public Builder retention(OptionRetention retention) {
this.retention = retention;
return this;
}
public Builder targets(List targets) {
Internal.checkElementsNotNull(targets);
this.targets = targets;
return this;
}
public Builder edition_defaults(List edition_defaults) {
Internal.checkElementsNotNull(edition_defaults);
this.edition_defaults = edition_defaults;
return this;
}
/**
* Any features defined in the specific edition.
*/
public Builder features(FeatureSet features) {
this.features = features;
return this;
}
/**
* The parser stores options it doesn't recognize here. See above.
*/
public Builder uninterpreted_option(List uninterpreted_option) {
Internal.checkElementsNotNull(uninterpreted_option);
this.uninterpreted_option = uninterpreted_option;
return this;
}
/**
* Annotates a field that starts to be available with a specified version.
*
* The value is the earliest version in which the annotated field is retained. It is pruned from
* all preceding versions.
*/
public Builder since(String since) {
this.since = since;
return this;
}
/**
* Annotates a field that ceases to be available with a specified version.
*
* The value is the version that drops the annotated field. It is not available in that version or
* any subsequent version.
*/
public Builder until(String until) {
this.until = until;
return this;
}
/**
* Uses an Array at runtime to hold the values, to avoid autoboxing on the JVM. Will use the appropriate
* array type, for example `repeated float` would be represented as a FloatArray.
*/
public Builder use_array(Boolean use_array) {
this.use_array = use_array;
return this;
}
@Override
public FieldOptions build() {
return new FieldOptions(this, super.buildUnknownFields());
}
}
public enum CType implements WireEnum {
/**
* Default mode.
*/
STRING(0),
/**
* The option [ctype=CORD] may be applied to a non-repeated field of type
* "bytes". It indicates that in C++, the data should be stored in a Cord
* instead of a string. For very large strings, this may reduce memory
* fragmentation. It may also allow better performance when parsing from a
* Cord, or when parsing with aliasing enabled, as the parsed Cord may then
* alias the original buffer.
*/
CORD(1),
STRING_PIECE(2);
public static final ProtoAdapter ADAPTER = new ProtoAdapter_CType();
private final int value;
CType(int value) {
this.value = value;
}
/**
* Return the constant for {@code value} or null.
*/
public static CType fromValue(int value) {
switch (value) {
case 0: return STRING;
case 1: return CORD;
case 2: return STRING_PIECE;
default: return null;
}
}
@Override
public int getValue() {
return value;
}
private static final class ProtoAdapter_CType extends EnumAdapter {
ProtoAdapter_CType() {
super(CType.class, Syntax.PROTO_2, CType.STRING);
}
@Override
protected CType fromValue(int value) {
return CType.fromValue(value);
}
}
}
public enum JSType implements WireEnum {
/**
* Use the default type.
*/
JS_NORMAL(0),
/**
* Use JavaScript strings.
*/
JS_STRING(1),
/**
* Use JavaScript numbers.
*/
JS_NUMBER(2);
public static final ProtoAdapter ADAPTER = new ProtoAdapter_JSType();
private final int value;
JSType(int value) {
this.value = value;
}
/**
* Return the constant for {@code value} or null.
*/
public static JSType fromValue(int value) {
switch (value) {
case 0: return JS_NORMAL;
case 1: return JS_STRING;
case 2: return JS_NUMBER;
default: return null;
}
}
@Override
public int getValue() {
return value;
}
private static final class ProtoAdapter_JSType extends EnumAdapter {
ProtoAdapter_JSType() {
super(JSType.class, Syntax.PROTO_2, JSType.JS_NORMAL);
}
@Override
protected JSType fromValue(int value) {
return JSType.fromValue(value);
}
}
}
/**
* If set to RETENTION_SOURCE, the option will be omitted from the binary.
* Note: as of January 2023, support for this is in progress and does not yet
* have an effect (b/264593489).
*/
public enum OptionRetention implements WireEnum {
RETENTION_UNKNOWN(0),
RETENTION_RUNTIME(1),
RETENTION_SOURCE(2);
public static final ProtoAdapter ADAPTER = new ProtoAdapter_OptionRetention();
private final int value;
OptionRetention(int value) {
this.value = value;
}
/**
* Return the constant for {@code value} or null.
*/
public static OptionRetention fromValue(int value) {
switch (value) {
case 0: return RETENTION_UNKNOWN;
case 1: return RETENTION_RUNTIME;
case 2: return RETENTION_SOURCE;
default: return null;
}
}
@Override
public int getValue() {
return value;
}
private static final class ProtoAdapter_OptionRetention extends EnumAdapter {
ProtoAdapter_OptionRetention() {
super(OptionRetention.class, Syntax.PROTO_2, OptionRetention.RETENTION_UNKNOWN);
}
@Override
protected OptionRetention fromValue(int value) {
return OptionRetention.fromValue(value);
}
}
}
/**
* This indicates the types of entities that the field may apply to when used
* as an option. If it is unset, then the field may be freely used as an
* option on any kind of entity. Note: as of January 2023, support for this is
* in progress and does not yet have an effect (b/264593489).
*/
public enum OptionTargetType implements WireEnum {
TARGET_TYPE_UNKNOWN(0),
TARGET_TYPE_FILE(1),
TARGET_TYPE_EXTENSION_RANGE(2),
TARGET_TYPE_MESSAGE(3),
TARGET_TYPE_FIELD(4),
TARGET_TYPE_ONEOF(5),
TARGET_TYPE_ENUM(6),
TARGET_TYPE_ENUM_ENTRY(7),
TARGET_TYPE_SERVICE(8),
TARGET_TYPE_METHOD(9);
public static final ProtoAdapter ADAPTER = new ProtoAdapter_OptionTargetType();
private final int value;
OptionTargetType(int value) {
this.value = value;
}
/**
* Return the constant for {@code value} or null.
*/
public static OptionTargetType fromValue(int value) {
switch (value) {
case 0: return TARGET_TYPE_UNKNOWN;
case 1: return TARGET_TYPE_FILE;
case 2: return TARGET_TYPE_EXTENSION_RANGE;
case 3: return TARGET_TYPE_MESSAGE;
case 4: return TARGET_TYPE_FIELD;
case 5: return TARGET_TYPE_ONEOF;
case 6: return TARGET_TYPE_ENUM;
case 7: return TARGET_TYPE_ENUM_ENTRY;
case 8: return TARGET_TYPE_SERVICE;
case 9: return TARGET_TYPE_METHOD;
default: return null;
}
}
@Override
public int getValue() {
return value;
}
private static final class ProtoAdapter_OptionTargetType extends EnumAdapter {
ProtoAdapter_OptionTargetType() {
super(OptionTargetType.class, Syntax.PROTO_2, OptionTargetType.TARGET_TYPE_UNKNOWN);
}
@Override
protected OptionTargetType fromValue(int value) {
return OptionTargetType.fromValue(value);
}
}
}
public static final class EditionDefault extends Message {
public static final ProtoAdapter ADAPTER = new ProtoAdapter_EditionDefault();
private static final long serialVersionUID = 0L;
public static final Edition DEFAULT_EDITION = Edition.EDITION_UNKNOWN;
public static final String DEFAULT_VALUE = "";
@WireField(
tag = 3,
adapter = "com.google.protobuf.Edition#ADAPTER"
)
public final Edition edition;
/**
* Textproto value.
*/
@WireField(
tag = 2,
adapter = "com.squareup.wire.ProtoAdapter#STRING"
)
public final String value;
public EditionDefault(Edition edition, String value) {
this(edition, value, ByteString.EMPTY);
}
public EditionDefault(Edition edition, String value, ByteString unknownFields) {
super(ADAPTER, unknownFields);
this.edition = edition;
this.value = value;
}
@Override
public Builder newBuilder() {
Builder builder = new Builder();
builder.edition = edition;
builder.value = value;
builder.addUnknownFields(unknownFields());
return builder;
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof EditionDefault)) return false;
EditionDefault o = (EditionDefault) other;
return unknownFields().equals(o.unknownFields())
&& Internal.equals(edition, o.edition)
&& Internal.equals(value, o.value);
}
@Override
public int hashCode() {
int result = super.hashCode;
if (result == 0) {
result = unknownFields().hashCode();
result = result * 37 + (edition != null ? edition.hashCode() : 0);
result = result * 37 + (value != null ? value.hashCode() : 0);
super.hashCode = result;
}
return result;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
if (edition != null) builder.append(", edition=").append(edition);
if (value != null) builder.append(", value=").append(Internal.sanitize(value));
return builder.replace(0, 2, "EditionDefault{").append('}').toString();
}
public static final class Builder extends Message.Builder {
public Edition edition;
public String value;
public Builder() {
}
public Builder edition(Edition edition) {
this.edition = edition;
return this;
}
/**
* Textproto value.
*/
public Builder value(String value) {
this.value = value;
return this;
}
@Override
public EditionDefault build() {
return new EditionDefault(edition, value, super.buildUnknownFields());
}
}
private static final class ProtoAdapter_EditionDefault extends ProtoAdapter {
public ProtoAdapter_EditionDefault() {
super(FieldEncoding.LENGTH_DELIMITED, EditionDefault.class, "type.googleapis.com/google.protobuf.FieldOptions.EditionDefault", Syntax.PROTO_2, null, "google/protobuf/descriptor.proto");
}
@Override
public int encodedSize(EditionDefault value) {
int result = 0;
result += Edition.ADAPTER.encodedSizeWithTag(3, value.edition);
result += ProtoAdapter.STRING.encodedSizeWithTag(2, value.value);
result += value.unknownFields().size();
return result;
}
@Override
public void encode(ProtoWriter writer, EditionDefault value) throws IOException {
Edition.ADAPTER.encodeWithTag(writer, 3, value.edition);
ProtoAdapter.STRING.encodeWithTag(writer, 2, value.value);
writer.writeBytes(value.unknownFields());
}
@Override
public void encode(ReverseProtoWriter writer, EditionDefault value) throws IOException {
writer.writeBytes(value.unknownFields());
ProtoAdapter.STRING.encodeWithTag(writer, 2, value.value);
Edition.ADAPTER.encodeWithTag(writer, 3, value.edition);
}
@Override
public EditionDefault decode(ProtoReader reader) throws IOException {
Builder builder = new Builder();
long token = reader.beginMessage();
for (int tag; (tag = reader.nextTag()) != -1;) {
switch (tag) {
case 2: builder.value(ProtoAdapter.STRING.decode(reader)); break;
case 3: {
try {
builder.edition(Edition.ADAPTER.decode(reader));
} catch (ProtoAdapter.EnumConstantNotFoundException e) {
builder.addUnknownField(tag, FieldEncoding.VARINT, (long) e.value);
}
break;
}
default: {
reader.readUnknownField(tag);
}
}
}
builder.addUnknownFields(reader.endMessageAndGetUnknownFields(token));
return builder.build();
}
@Override
public EditionDefault redact(EditionDefault value) {
Builder builder = value.newBuilder();
builder.clearUnknownFields();
return builder.build();
}
}
}
private static final class ProtoAdapter_FieldOptions extends ProtoAdapter {
public ProtoAdapter_FieldOptions() {
super(FieldEncoding.LENGTH_DELIMITED, FieldOptions.class, "type.googleapis.com/google.protobuf.FieldOptions", Syntax.PROTO_2, null, "google/protobuf/descriptor.proto");
}
@Override
public int encodedSize(FieldOptions value) {
int result = 0;
result += CType.ADAPTER.encodedSizeWithTag(1, value.ctype);
result += ProtoAdapter.BOOL.encodedSizeWithTag(2, value.packed);
result += JSType.ADAPTER.encodedSizeWithTag(6, value.jstype);
result += ProtoAdapter.BOOL.encodedSizeWithTag(5, value.lazy);
result += ProtoAdapter.BOOL.encodedSizeWithTag(15, value.unverified_lazy);
result += ProtoAdapter.BOOL.encodedSizeWithTag(3, value.deprecated);
result += ProtoAdapter.BOOL.encodedSizeWithTag(10, value.weak);
result += ProtoAdapter.BOOL.encodedSizeWithTag(16, value.debug_redact);
result += OptionRetention.ADAPTER.encodedSizeWithTag(17, value.retention);
result += OptionTargetType.ADAPTER.asRepeated().encodedSizeWithTag(19, value.targets);
result += EditionDefault.ADAPTER.asRepeated().encodedSizeWithTag(20, value.edition_defaults);
result += FeatureSet.ADAPTER.encodedSizeWithTag(21, value.features);
result += UninterpretedOption.ADAPTER.asRepeated().encodedSizeWithTag(999, value.uninterpreted_option);
result += ProtoAdapter.STRING.encodedSizeWithTag(1076, value.since);
result += ProtoAdapter.STRING.encodedSizeWithTag(1077, value.until);
result += ProtoAdapter.BOOL.encodedSizeWithTag(1185, value.use_array);
result += value.unknownFields().size();
return result;
}
@Override
public void encode(ProtoWriter writer, FieldOptions value) throws IOException {
CType.ADAPTER.encodeWithTag(writer, 1, value.ctype);
ProtoAdapter.BOOL.encodeWithTag(writer, 2, value.packed);
JSType.ADAPTER.encodeWithTag(writer, 6, value.jstype);
ProtoAdapter.BOOL.encodeWithTag(writer, 5, value.lazy);
ProtoAdapter.BOOL.encodeWithTag(writer, 15, value.unverified_lazy);
ProtoAdapter.BOOL.encodeWithTag(writer, 3, value.deprecated);
ProtoAdapter.BOOL.encodeWithTag(writer, 10, value.weak);
ProtoAdapter.BOOL.encodeWithTag(writer, 16, value.debug_redact);
OptionRetention.ADAPTER.encodeWithTag(writer, 17, value.retention);
OptionTargetType.ADAPTER.asRepeated().encodeWithTag(writer, 19, value.targets);
EditionDefault.ADAPTER.asRepeated().encodeWithTag(writer, 20, value.edition_defaults);
FeatureSet.ADAPTER.encodeWithTag(writer, 21, value.features);
UninterpretedOption.ADAPTER.asRepeated().encodeWithTag(writer, 999, value.uninterpreted_option);
ProtoAdapter.STRING.encodeWithTag(writer, 1076, value.since);
ProtoAdapter.STRING.encodeWithTag(writer, 1077, value.until);
ProtoAdapter.BOOL.encodeWithTag(writer, 1185, value.use_array);
writer.writeBytes(value.unknownFields());
}
@Override
public void encode(ReverseProtoWriter writer, FieldOptions value) throws IOException {
writer.writeBytes(value.unknownFields());
ProtoAdapter.BOOL.encodeWithTag(writer, 1185, value.use_array);
ProtoAdapter.STRING.encodeWithTag(writer, 1077, value.until);
ProtoAdapter.STRING.encodeWithTag(writer, 1076, value.since);
UninterpretedOption.ADAPTER.asRepeated().encodeWithTag(writer, 999, value.uninterpreted_option);
FeatureSet.ADAPTER.encodeWithTag(writer, 21, value.features);
EditionDefault.ADAPTER.asRepeated().encodeWithTag(writer, 20, value.edition_defaults);
OptionTargetType.ADAPTER.asRepeated().encodeWithTag(writer, 19, value.targets);
OptionRetention.ADAPTER.encodeWithTag(writer, 17, value.retention);
ProtoAdapter.BOOL.encodeWithTag(writer, 16, value.debug_redact);
ProtoAdapter.BOOL.encodeWithTag(writer, 10, value.weak);
ProtoAdapter.BOOL.encodeWithTag(writer, 3, value.deprecated);
ProtoAdapter.BOOL.encodeWithTag(writer, 15, value.unverified_lazy);
ProtoAdapter.BOOL.encodeWithTag(writer, 5, value.lazy);
JSType.ADAPTER.encodeWithTag(writer, 6, value.jstype);
ProtoAdapter.BOOL.encodeWithTag(writer, 2, value.packed);
CType.ADAPTER.encodeWithTag(writer, 1, value.ctype);
}
@Override
public FieldOptions decode(ProtoReader reader) throws IOException {
Builder builder = new Builder();
long token = reader.beginMessage();
for (int tag; (tag = reader.nextTag()) != -1;) {
switch (tag) {
case 1: {
try {
builder.ctype(CType.ADAPTER.decode(reader));
} catch (ProtoAdapter.EnumConstantNotFoundException e) {
builder.addUnknownField(tag, FieldEncoding.VARINT, (long) e.value);
}
break;
}
case 2: builder.packed(ProtoAdapter.BOOL.decode(reader)); break;
case 3: builder.deprecated(ProtoAdapter.BOOL.decode(reader)); break;
case 5: builder.lazy(ProtoAdapter.BOOL.decode(reader)); break;
case 6: {
try {
builder.jstype(JSType.ADAPTER.decode(reader));
} catch (ProtoAdapter.EnumConstantNotFoundException e) {
builder.addUnknownField(tag, FieldEncoding.VARINT, (long) e.value);
}
break;
}
case 10: builder.weak(ProtoAdapter.BOOL.decode(reader)); break;
case 15: builder.unverified_lazy(ProtoAdapter.BOOL.decode(reader)); break;
case 16: builder.debug_redact(ProtoAdapter.BOOL.decode(reader)); break;
case 17: {
try {
builder.retention(OptionRetention.ADAPTER.decode(reader));
} catch (ProtoAdapter.EnumConstantNotFoundException e) {
builder.addUnknownField(tag, FieldEncoding.VARINT, (long) e.value);
}
break;
}
case 19: {
try {
builder.targets.add(OptionTargetType.ADAPTER.decode(reader));
} catch (ProtoAdapter.EnumConstantNotFoundException e) {
builder.addUnknownField(tag, FieldEncoding.VARINT, (long) e.value);
}
break;
}
case 20: builder.edition_defaults.add(EditionDefault.ADAPTER.decode(reader)); break;
case 21: builder.features(FeatureSet.ADAPTER.decode(reader)); break;
case 999: builder.uninterpreted_option.add(UninterpretedOption.ADAPTER.decode(reader)); break;
case 1076: builder.since(ProtoAdapter.STRING.decode(reader)); break;
case 1077: builder.until(ProtoAdapter.STRING.decode(reader)); break;
case 1185: builder.use_array(ProtoAdapter.BOOL.decode(reader)); break;
default: {
reader.readUnknownField(tag);
}
}
}
builder.addUnknownFields(reader.endMessageAndGetUnknownFields(token));
return builder.build();
}
@Override
public FieldOptions redact(FieldOptions value) {
Builder builder = value.newBuilder();
Internal.redactElements(builder.edition_defaults, EditionDefault.ADAPTER);
if (builder.features != null) builder.features = FeatureSet.ADAPTER.redact(builder.features);
Internal.redactElements(builder.uninterpreted_option, UninterpretedOption.ADAPTER);
builder.clearUnknownFields();
return builder.build();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy