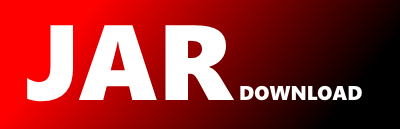
is.codion.common.rmi.server.ServerConfiguration Maven / Gradle / Ivy
/*
* This file is part of Codion.
*
* Codion is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Codion is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Codion. If not, see .
*
* Copyright (c) 2020 - 2024, Björn Darri Sigurðsson.
*/
package is.codion.common.rmi.server;
import is.codion.common.Configuration;
import is.codion.common.Text;
import is.codion.common.property.PropertyValue;
import java.rmi.registry.Registry;
import java.rmi.server.RMIClientSocketFactory;
import java.rmi.server.RMIServerSocketFactory;
import java.util.Collection;
import java.util.Optional;
import java.util.function.Supplier;
/**
* Configuration values for a {@link Server}.
* @see #builder(int)
* @see #builder(int, int)
* @see #builderFromSystemProperties()
*/
public interface ServerConfiguration {
/**
* The default idle connection timeout in milliseconds.
*/
int DEFAULT_IDLE_CONNECTION_TIMEOUT = 120_000;
/**
* The default connection maintenance interval in milliseconds.
*/
int DEFAULT_CONNECTION_MAINTENANCE_INTERVAL = 30_000;
/**
* The system property key for specifying a ssl keystore
*/
String JAVAX_NET_KEYSTORE = "javax.net.ssl.keyStore";
/**
* The system property key for specifying a ssl keystore password
*/
String JAVAX_NET_KEYSTORE_PASSWORD = "javax.net.ssl.keyStorePassword";
/**
* Localhost
*/
String LOCALHOST = "localhost";
/**
* Specifies the rmi server hostname
* Note that this is the standard Java property 'java.rmi.server.hostname
*
* - Value type: String
*
- Default value: localhost
*
*/
PropertyValue RMI_SERVER_HOSTNAME = Configuration.stringValue("java.rmi.server.hostname", LOCALHOST);
/**
* Specifies the prefix used when exporting/looking up the Codion server
*
* - Value type: String
*
- Default value: Codion Server
*
*/
PropertyValue SERVER_NAME_PREFIX = Configuration.stringValue("codion.server.namePrefix", "Codion Server");
/**
* The port on which the server is made available to clients.
* If specified on the client side, the client will only connect to a server running on this port,
* use -1 or no value if the client should connect to any available server
*
* - Value type: Integer
*
- Default value: -1
*
*/
PropertyValue SERVER_PORT = Configuration.integerValue("codion.server.port", -1);
/**
* The port on which to locate the server registry
*
* - Value type: Integer
*
- Default value: {@link Registry#REGISTRY_PORT} (1099)
*
*/
PropertyValue REGISTRY_PORT = Configuration.integerValue("codion.server.registryPort", Registry.REGISTRY_PORT);
/**
* The rmi ssl keystore to use on the classpath, this will be resolved to a temporary file and set
* as the javax.net.ssl.keyStore system property on server start
*
* - Value type: String
*
- Default value: null
*
*/
PropertyValue CLASSPATH_KEYSTORE = Configuration.stringValue("codion.server.classpathKeyStore");
/**
* The rmi ssl keystore to use
*
* - Value type: String
*
- Default value: null
*
* @see #CLASSPATH_KEYSTORE
*/
PropertyValue KEYSTORE = Configuration.stringValue(JAVAX_NET_KEYSTORE);
/**
* The rmi ssl keystore password to use
*
* - Value type: String
*
- Default value: null
*
*/
PropertyValue KEYSTORE_PASSWORD = Configuration.stringValue(JAVAX_NET_KEYSTORE_PASSWORD);
/**
* The port on which the server should export the remote admin interface
*
* - Value type: Integer
*
- Default value: 0 (admin not exported)
*
*/
PropertyValue ADMIN_PORT = Configuration.integerValue("codion.server.admin.port", 0);
/**
* Specifies a username:password combination representing the server admin user
* Example: scott:tiger
*
* - Default value: none
*
*/
PropertyValue ADMIN_USER = Configuration.stringValue("codion.server.admin.user");
/**
* Specifies whether the server should establish connections using a secure sockets layer, true (on) or false (off
*
* - Value type: Boolean
*
- Default value: true
*
*/
PropertyValue SSL_ENABLED = Configuration.booleanValue("codion.server.connection.sslEnabled", true);
/**
* Specifies the default idle client connection timeout in milliseconds.
*
* - Value type: Integer
*
- Default value: 120.000ms (2 minutes)
*
*/
PropertyValue IDLE_CONNECTION_TIMEOUT = Configuration.integerValue("codion.server.idleConnectionTimeout", DEFAULT_IDLE_CONNECTION_TIMEOUT);
/**
* A comma separated list of auxiliary server factories, providing servers to run alongside this Server
* Those must extend {@link AuxiliaryServerFactory}
*
* - Value type: String
*
- Default value: none
*
* @see AuxiliaryServer
*/
PropertyValue AUXILIARY_SERVER_FACTORY_CLASS_NAMES = Configuration.stringValue("codion.server.auxiliaryServerFactoryClassNames");
/**
* Specifies the {@link ObjectInputFilterFactory} class to use
*
* - Value type: String
*
- Default value: none
*
* @see ObjectInputFilterFactory
*/
PropertyValue OBJECT_INPUT_FILTER_FACTORY_CLASS_NAME = Configuration.stringValue("codion.server.objectInputFilterFactoryClassName");
/**
* Specifies the interval between server connection maintenance runs, in milliseconds
*
* - Value type: Integer
*
- Default value: 30_000ms (30 seconds)
*
*/
PropertyValue CONNECTION_MAINTENANCE_INTERVAL = Configuration.integerValue("codion.server.connectionMaintenanceInterval", DEFAULT_CONNECTION_MAINTENANCE_INTERVAL);
/**
* @return the server name
* @see Builder#serverName(Supplier)
*/
String serverName();
/**
* @return the server port
*/
int port();
/**
* @return the registry port to use
*/
int registryPort();
/**
* @return the port on which to make the server admin interface accessible
*/
int adminPort();
/**
* @return the class names of auxiliary server factories, providing the servers to run alongside this server
*/
Collection auxiliaryServerFactoryClassNames();
/**
* @return true if ssl is enabled
*/
boolean sslEnabled();
/**
* @return the rmi client socket factory to use, or an empty Optional if none is specified
*/
Optional rmiClientSocketFactory();
/**
* @return the rmi server socket factory to use, or an empty Optional if none is specified
*/
Optional rmiServerSocketFactory();
/**
* @return the object input filter factory class name, or an empty Optional if none is specified
*/
Optional objectInputFilterFactoryClassName();
/**
* @return the interval between server connection maintenance runs, in milliseconds.
*/
int connectionMaintenanceInterval();
/**
* @return the maximum number of concurrent connections, -1 for no limit
*/
int connectionLimit();
/**
* A Builder for ServerConfiguration
* @param the builder type
*/
interface Builder> {
/**
* @param adminPort the port on which to make the server admin interface accessible
* @return this builder instance
*/
B adminPort(int adminPort);
/**
* @param serverNameSupplier the server name supplier
* @return this builder instance
*/
B serverName(Supplier serverNameSupplier);
/**
* @param serverName the server name
* @return this builder instance
*/
B serverName(String serverName);
/**
* @param auxiliaryServerFactoryClassNames the class names of auxiliary server factories,
* providing the servers to run alongside this server
* @return this builder instance
*/
B auxiliaryServerFactoryClassNames(Collection auxiliaryServerFactoryClassNames);
/**
* When set to true this also sets the rmi client/server socket factories.
* @param sslEnabled if true then ssl is enabled
* @return this builder instance
* @see #rmiClientSocketFactory(RMIClientSocketFactory)
* @see #rmiServerSocketFactory(RMIServerSocketFactory)
*/
B sslEnabled(boolean sslEnabled);
/**
* @param rmiClientSocketFactory the rmi client socket factory to use
* @return this builder instance
*/
B rmiClientSocketFactory(RMIClientSocketFactory rmiClientSocketFactory);
/**
* @param rmiServerSocketFactory the rmi server socket factory to use
* @return this builder instance
*/
B rmiServerSocketFactory(RMIServerSocketFactory rmiServerSocketFactory);
/**
* @param objectInputFilterFactoryClassName the object input filter factory class name
* @return this builder instance
*/
B objectInputFilterFactoryClassName(String objectInputFilterFactoryClassName);
/**
* @param connectionMaintenanceInterval the interval between server connection maintenance runs, in milliseconds.
* @return this builder instance
*/
B connectionMaintenanceInterval(int connectionMaintenanceInterval);
/**
* @param connectionLimit the maximum number of concurrent connections, -1 for no limit
* @return this builder instance
*/
B connectionLimit(int connectionLimit);
/**
* @return a new ServerConfiguration instance based on this builder
*/
ServerConfiguration build();
}
/**
* @param serverPort the server port
* @param the builder type
* @return a default server configuration
*/
static > Builder builder(int serverPort) {
return (Builder) new DefaultServerConfiguration.DefaultBuilder(serverPort, Registry.REGISTRY_PORT);
}
/**
* @param serverPort the server port
* @param registryPort the registry port
* @param the builder type
* @return a default server configuration
*/
static > Builder builder(int serverPort, int registryPort) {
return (Builder) new DefaultServerConfiguration.DefaultBuilder(serverPort, registryPort);
}
/**
* Returns a Builder initialized with values from system properties.
* @param the builder type
* @return a server configuration builder initialized with values from system properties.
*/
static > Builder builderFromSystemProperties() {
return (Builder) builder(SERVER_PORT.getOrThrow(), REGISTRY_PORT.getOrThrow())
.auxiliaryServerFactoryClassNames(Text.parseCommaSeparatedValues(AUXILIARY_SERVER_FACTORY_CLASS_NAMES.get()))
.adminPort(ADMIN_PORT.get())
.sslEnabled(SSL_ENABLED.get())
.connectionMaintenanceInterval(CONNECTION_MAINTENANCE_INTERVAL.get())
.objectInputFilterFactoryClassName(OBJECT_INPUT_FILTER_FACTORY_CLASS_NAME.get());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy