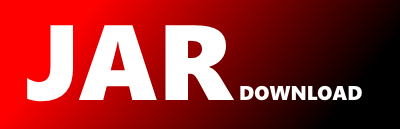
is.codion.framework.model.EntityEditEvents Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codion-framework-model Show documentation
Show all versions of codion-framework-model Show documentation
Codion Application Framework
/*
* This file is part of Codion.
*
* Codion is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Codion is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Codion. If not, see .
*
* Copyright (c) 2019 - 2024, Björn Darri Sigurðsson.
*/
package is.codion.framework.model;
import is.codion.common.event.Event;
import is.codion.common.observer.Observer;
import is.codion.framework.domain.entity.Entity;
import is.codion.framework.domain.entity.EntityType;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import static is.codion.common.event.Event.event;
import static is.codion.framework.domain.entity.Entity.groupByType;
import static java.util.Collections.synchronizedMap;
import static java.util.Objects.requireNonNull;
import static java.util.stream.Collectors.*;
/**
* A central event hub for listening for entity inserts, updates and deletes.
* {@link EntityEditModel} uses this to post its events.
* @see EntityEditModel#POST_EDIT_EVENTS
*/
public final class EntityEditEvents {
private static final EntityEditListener EDIT_LISTENER = new EntityEditListener();
private EntityEditEvents() {}
/**
* Returns an insert observer, notified each time entities of the given type are inserted.
* @param entityType the type of entity to listen for
* @return the insert observer for the given entity type
*/
public static Observer> insertObserver(EntityType entityType) {
return EDIT_LISTENER.insertObserver(requireNonNull(entityType));
}
/**
* Returns an update observer, notified each time entities of the given type are updated.
* @param entityType the type of entity to listen for
* @return the update observer for the given entity type
*/
public static Observer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy