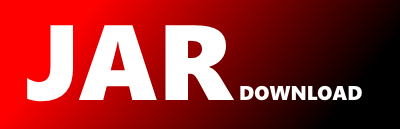
it.cnr.iit.jscontact.tools.dto.Address Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Consiglio Nazionale delle Ricerche
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package it.cnr.iit.jscontact.tools.dto;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import it.cnr.iit.jscontact.tools.constraints.BooleanMapConstraint;
import it.cnr.iit.jscontact.tools.dto.annotations.JSContactCollection;
import it.cnr.iit.jscontact.tools.dto.deserializers.AddressContextsDeserializer;
import it.cnr.iit.jscontact.tools.dto.interfaces.HasLabel;
import it.cnr.iit.jscontact.tools.dto.interfaces.IdMapValue;
import it.cnr.iit.jscontact.tools.dto.serializers.AddressContextsSerializer;
import it.cnr.iit.jscontact.tools.dto.utils.DelimiterUtils;
import lombok.*;
import lombok.experimental.SuperBuilder;
import org.apache.commons.lang3.ArrayUtils;
import org.apache.commons.lang3.StringUtils;
import javax.validation.constraints.Max;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Pattern;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import java.util.StringJoiner;
/**
* Class mapping the Address type as defined in section 2.5.1 of [draft-ietf-calext-jscontact].
*
* @see draft-ietf-calext-jscontact
* @author Mario Loffredo
*/
@JsonPropertyOrder({"@type","fullAddress","street","locality","region","country",
"postcode","countryCode","coordinates","timeZone",
"contexts","pref","label"})
@JsonInclude(JsonInclude.Include.NON_NULL)
@SuperBuilder
@Data
@AllArgsConstructor
@NoArgsConstructor
@EqualsAndHashCode(of={"hash"}, callSuper = false)
public class Address extends AbstractJSContactType implements HasLabel, IdMapValue, Serializable {
@NotNull
@Pattern(regexp = "Address", message="invalid @type value in Address")
@JsonProperty("@type")
@Builder.Default
String _type = "Address";
String fullAddress;
@JSContactCollection(addMethod = "addComponent")
StreetComponent[] street;
String locality;
String region;
String country;
String postcode;
@Pattern(regexp="[a-zA-Z]{2}", message = "invalid countryCode in Address")
String countryCode;
@Pattern(regexp="geo:([\\-0-9.]+),([\\-0-9.]+)(?:,([\\-0-9.]+))?(?:\\?(.*))?$", message = "invalid coordinates in Address")
String coordinates;
String timeZone;
@JsonSerialize(using = AddressContextsSerializer.class)
@JsonDeserialize(using = AddressContextsDeserializer.class)
@BooleanMapConstraint(message = "invalid Map contexts in Address - Only Boolean.TRUE allowed")
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@Singular(ignoreNullCollections = true)
Map contexts;
@Min(value=1, message = "invalid pref in Address - value must be greater or equal than 1")
@Max(value=100, message = "invalid pref in Address - value must be less or equal than 100")
Integer pref;
String label;
@JsonIgnore
String altid;
@JsonIgnore
String language;
@JsonIgnore
String group;
@JsonIgnore
String hash;
private boolean asContext(AddressContext context) { return contexts != null && contexts.containsKey(context); }
/**
* Tests if this address is a work address.
*
* @return true if the context map includes the "work" context, false otherwise
*/
public boolean asWork() { return asContext(AddressContext.work()); }
/**
* Tests if this address is a private address.
*
* @return true if the context map includes the "private" context, false otherwise
*/
public boolean asPrivate() { return asContext(AddressContext.private_()); }
/**
* Tests if this address is a billing address.
*
* @return true if the context map includes the "billing" context, false otherwise
*/
public boolean asBilling() {
return asContext(AddressContext.billing());
}
/**
* Tests if this address is a delivery address.
*
* @return true if the context map includes the "delivery" context, false otherwise
*/
public boolean asDelivery() {
return asContext(AddressContext.delivery());
}
/**
* Tests if this address is used in a custom context.
*
* @param extValue the custom context in text format
* @return true if the context map includes the given custom context, false otherwise
*/
public boolean asExtContext(String extValue) {
return asContext(AddressContext.ext(extValue));
}
/**
* Tests if the context of this address is undefined.
*
* @return true if the context map is empty, false otherwise
*/
public boolean hasNoContext() { return contexts == null || contexts.size() == 0; }
private String getStreetDetail(StreetComponentEnum detail) {
if (street == null)
return null;
for (StreetComponent pair : street) {
if (!pair.isExt() && pair.getType().getRfcValue() == detail)
return pair.getValue();
}
return null;
}
/**
* Returns the P.O. box of this object.
*
* @return the value of StreetComponent item in the "street" array tagged as POST_OFFICE_BOX
*/
@JsonIgnore
public String getPostOfficeBox() {
return getStreetDetail(StreetComponentEnum.POST_OFFICE_BOX);
}
/**
* Returns the street details of this object.
*
* @return a text obtained by concatenating the values of StreetComponent items in the "street" array tagged as NAME, NUMBER or DIRECTION. The items are separated by the value of the item tagged as SEPARATOR if it isn't empty, space otherwise
*/
@JsonIgnore
public String getStreetDetails() {
String separator = getStreetDetail(StreetComponentEnum.SEPARATOR);
StringJoiner joiner = new StringJoiner( (separator != null) ? separator : DelimiterUtils.SPACE_DELIMITER);
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.NAME))) joiner.add(getStreetDetail(StreetComponentEnum.NAME));
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.NUMBER))) joiner.add(getStreetDetail(StreetComponentEnum.NUMBER));
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.DIRECTION))) joiner.add(getStreetDetail(StreetComponentEnum.DIRECTION));
String streetDetails = joiner.toString();
return StringUtils.defaultIfEmpty(streetDetails, null);
}
/**
* Returns the street extensions of this object.
*
* @return a text obtained by concatenating the values of the StreetComponent items in the "street" array tagged as BUILDING, FLOOR, APARTMENT, ROOM, EXTENSION or UNKNOWN. The items are separated by the item tagged as SEPARATOR if it isn't empty, space otherwise
*/
@JsonIgnore
public String getStreetExtensions() {
String separator = getStreetDetail(StreetComponentEnum.SEPARATOR);
StringJoiner joiner = new StringJoiner( (separator != null) ? separator : DelimiterUtils.SPACE_DELIMITER);
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.BUILDING))) joiner.add("Building: " + getStreetDetail(StreetComponentEnum.BUILDING));
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.FLOOR))) joiner.add("Floor: " + getStreetDetail(StreetComponentEnum.FLOOR));
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.APARTMENT))) joiner.add("Apartment: " + getStreetDetail(StreetComponentEnum.APARTMENT));
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.ROOM))) joiner.add("Room: " + getStreetDetail(StreetComponentEnum.ROOM));
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.EXTENSION))) joiner.add(getStreetDetail(StreetComponentEnum.EXTENSION));
if (StringUtils.isNotEmpty(getStreetDetail(StreetComponentEnum.UNKNOWN))) joiner.add(getStreetDetail(StreetComponentEnum.EXTENSION));
String streetExtensions = joiner.toString();
return StringUtils.defaultIfEmpty(streetExtensions, null);
}
/**
* Adds a street component to this object.
*
* @param sc the street component
* @param components the street components
* @return the street components in input plus the sc component
*/
public static StreetComponent[] addComponent(StreetComponent[] components, StreetComponent sc) {
return ArrayUtils.add(components, sc);
}
/**
* Adds a street component to this object.
*
* @param sc the street component
*/
public void addComponent(StreetComponent sc) {
street = ArrayUtils.add(street, sc);
}
/**
* Adds a context to this object.
*
* @param context the context
*/
public void addContext(AddressContext context) {
Map clone = new HashMap<>(getContexts());
clone.put(context,Boolean.TRUE);
setContexts(clone);
}
/**
* This method will be used to get the extended contexts in the "contexts" property.
*
* @return the extended contexts in the "contexts" property
*/
@JsonIgnore
public AddressContext[] getExtContexts() {
if (getContexts() == null)
return null;
AddressContext[] extended = null;
for(AddressContext context : getContexts().keySet()){
if (context.isExtValue())
extended = ArrayUtils.add(extended, context);
}
return extended;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy