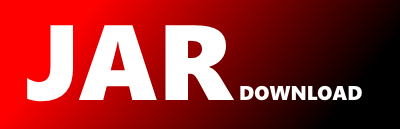
it.siopeplus.giornaledicassa.TotaliDisponibilitaLiquide Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.0
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.10.05 at 04:27:43 PM GMT
//
package it.siopeplus.giornaledicassa;
import java.io.Serializable;
import java.math.BigDecimal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString2;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy2;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="saldo_conti_correnti">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="saldo_conti_BI" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* <minInclusive value="0"/>
* </restriction>
* </simpleType>
* </element>
* <element name="totale_conti" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="vincoli_conti_correnti" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="vincoli_conti_BI" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="totale_vincoli" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="svincoli_conti_correnti" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="svincoli_conti_BI" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="totale_svincoli" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="anticipazione_accordata">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* <minInclusive value="0"/>
* </restriction>
* </simpleType>
* </element>
* <element name="anticipazione_utilizzata">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* <minInclusive value="0"/>
* </restriction>
* </simpleType>
* </element>
* <element name="totale_somme_bloccate_riservate" minOccurs="0">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* <element name="disponibilita">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}decimal">
* <totalDigits value="15"/>
* <fractionDigits value="2"/>
* </restriction>
* </simpleType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"saldoContiCorrenti",
"saldoContiBI",
"totaleConti",
"vincoliContiCorrenti",
"vincoliContiBI",
"totaleVincoli",
"svincoliContiCorrenti",
"svincoliContiBI",
"totaleSvincoli",
"anticipazioneAccordata",
"anticipazioneUtilizzata",
"totaleSommeBloccateRiservate",
"disponibilita"
})
@XmlRootElement(name = "totali_disponibilita_liquide", namespace = "")
public class TotaliDisponibilitaLiquide implements Serializable, ToString2
{
private final static long serialVersionUID = -1L;
@XmlElement(name = "saldo_conti_correnti", required = true)
protected BigDecimal saldoContiCorrenti;
@XmlElement(name = "saldo_conti_BI")
protected BigDecimal saldoContiBI;
@XmlElement(name = "totale_conti")
protected BigDecimal totaleConti;
@XmlElement(name = "vincoli_conti_correnti")
protected BigDecimal vincoliContiCorrenti;
@XmlElement(name = "vincoli_conti_BI")
protected BigDecimal vincoliContiBI;
@XmlElement(name = "totale_vincoli")
protected BigDecimal totaleVincoli;
@XmlElement(name = "svincoli_conti_correnti")
protected BigDecimal svincoliContiCorrenti;
@XmlElement(name = "svincoli_conti_BI")
protected BigDecimal svincoliContiBI;
@XmlElement(name = "totale_svincoli")
protected BigDecimal totaleSvincoli;
@XmlElement(name = "anticipazione_accordata", required = true)
protected BigDecimal anticipazioneAccordata;
@XmlElement(name = "anticipazione_utilizzata", required = true)
protected BigDecimal anticipazioneUtilizzata;
@XmlElement(name = "totale_somme_bloccate_riservate")
protected BigDecimal totaleSommeBloccateRiservate;
@XmlElement(required = true)
protected BigDecimal disponibilita;
/**
* Gets the value of the saldoContiCorrenti property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getSaldoContiCorrenti() {
return saldoContiCorrenti;
}
/**
* Sets the value of the saldoContiCorrenti property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setSaldoContiCorrenti(BigDecimal value) {
this.saldoContiCorrenti = value;
}
/**
* Gets the value of the saldoContiBI property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getSaldoContiBI() {
return saldoContiBI;
}
/**
* Sets the value of the saldoContiBI property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setSaldoContiBI(BigDecimal value) {
this.saldoContiBI = value;
}
/**
* Gets the value of the totaleConti property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTotaleConti() {
return totaleConti;
}
/**
* Sets the value of the totaleConti property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTotaleConti(BigDecimal value) {
this.totaleConti = value;
}
/**
* Gets the value of the vincoliContiCorrenti property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getVincoliContiCorrenti() {
return vincoliContiCorrenti;
}
/**
* Sets the value of the vincoliContiCorrenti property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setVincoliContiCorrenti(BigDecimal value) {
this.vincoliContiCorrenti = value;
}
/**
* Gets the value of the vincoliContiBI property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getVincoliContiBI() {
return vincoliContiBI;
}
/**
* Sets the value of the vincoliContiBI property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setVincoliContiBI(BigDecimal value) {
this.vincoliContiBI = value;
}
/**
* Gets the value of the totaleVincoli property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTotaleVincoli() {
return totaleVincoli;
}
/**
* Sets the value of the totaleVincoli property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTotaleVincoli(BigDecimal value) {
this.totaleVincoli = value;
}
/**
* Gets the value of the svincoliContiCorrenti property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getSvincoliContiCorrenti() {
return svincoliContiCorrenti;
}
/**
* Sets the value of the svincoliContiCorrenti property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setSvincoliContiCorrenti(BigDecimal value) {
this.svincoliContiCorrenti = value;
}
/**
* Gets the value of the svincoliContiBI property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getSvincoliContiBI() {
return svincoliContiBI;
}
/**
* Sets the value of the svincoliContiBI property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setSvincoliContiBI(BigDecimal value) {
this.svincoliContiBI = value;
}
/**
* Gets the value of the totaleSvincoli property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTotaleSvincoli() {
return totaleSvincoli;
}
/**
* Sets the value of the totaleSvincoli property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTotaleSvincoli(BigDecimal value) {
this.totaleSvincoli = value;
}
/**
* Gets the value of the anticipazioneAccordata property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAnticipazioneAccordata() {
return anticipazioneAccordata;
}
/**
* Sets the value of the anticipazioneAccordata property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setAnticipazioneAccordata(BigDecimal value) {
this.anticipazioneAccordata = value;
}
/**
* Gets the value of the anticipazioneUtilizzata property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAnticipazioneUtilizzata() {
return anticipazioneUtilizzata;
}
/**
* Sets the value of the anticipazioneUtilizzata property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setAnticipazioneUtilizzata(BigDecimal value) {
this.anticipazioneUtilizzata = value;
}
/**
* Gets the value of the totaleSommeBloccateRiservate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTotaleSommeBloccateRiservate() {
return totaleSommeBloccateRiservate;
}
/**
* Sets the value of the totaleSommeBloccateRiservate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTotaleSommeBloccateRiservate(BigDecimal value) {
this.totaleSommeBloccateRiservate = value;
}
/**
* Gets the value of the disponibilita property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getDisponibilita() {
return disponibilita;
}
/**
* Sets the value of the disponibilita property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setDisponibilita(BigDecimal value) {
this.disponibilita = value;
}
public String toString() {
final ToStringStrategy2 strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy2 strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy2 strategy) {
{
BigDecimal theSaldoContiCorrenti;
theSaldoContiCorrenti = this.getSaldoContiCorrenti();
strategy.appendField(locator, this, "saldoContiCorrenti", buffer, theSaldoContiCorrenti, (this.saldoContiCorrenti!= null));
}
{
BigDecimal theSaldoContiBI;
theSaldoContiBI = this.getSaldoContiBI();
strategy.appendField(locator, this, "saldoContiBI", buffer, theSaldoContiBI, (this.saldoContiBI!= null));
}
{
BigDecimal theTotaleConti;
theTotaleConti = this.getTotaleConti();
strategy.appendField(locator, this, "totaleConti", buffer, theTotaleConti, (this.totaleConti!= null));
}
{
BigDecimal theVincoliContiCorrenti;
theVincoliContiCorrenti = this.getVincoliContiCorrenti();
strategy.appendField(locator, this, "vincoliContiCorrenti", buffer, theVincoliContiCorrenti, (this.vincoliContiCorrenti!= null));
}
{
BigDecimal theVincoliContiBI;
theVincoliContiBI = this.getVincoliContiBI();
strategy.appendField(locator, this, "vincoliContiBI", buffer, theVincoliContiBI, (this.vincoliContiBI!= null));
}
{
BigDecimal theTotaleVincoli;
theTotaleVincoli = this.getTotaleVincoli();
strategy.appendField(locator, this, "totaleVincoli", buffer, theTotaleVincoli, (this.totaleVincoli!= null));
}
{
BigDecimal theSvincoliContiCorrenti;
theSvincoliContiCorrenti = this.getSvincoliContiCorrenti();
strategy.appendField(locator, this, "svincoliContiCorrenti", buffer, theSvincoliContiCorrenti, (this.svincoliContiCorrenti!= null));
}
{
BigDecimal theSvincoliContiBI;
theSvincoliContiBI = this.getSvincoliContiBI();
strategy.appendField(locator, this, "svincoliContiBI", buffer, theSvincoliContiBI, (this.svincoliContiBI!= null));
}
{
BigDecimal theTotaleSvincoli;
theTotaleSvincoli = this.getTotaleSvincoli();
strategy.appendField(locator, this, "totaleSvincoli", buffer, theTotaleSvincoli, (this.totaleSvincoli!= null));
}
{
BigDecimal theAnticipazioneAccordata;
theAnticipazioneAccordata = this.getAnticipazioneAccordata();
strategy.appendField(locator, this, "anticipazioneAccordata", buffer, theAnticipazioneAccordata, (this.anticipazioneAccordata!= null));
}
{
BigDecimal theAnticipazioneUtilizzata;
theAnticipazioneUtilizzata = this.getAnticipazioneUtilizzata();
strategy.appendField(locator, this, "anticipazioneUtilizzata", buffer, theAnticipazioneUtilizzata, (this.anticipazioneUtilizzata!= null));
}
{
BigDecimal theTotaleSommeBloccateRiservate;
theTotaleSommeBloccateRiservate = this.getTotaleSommeBloccateRiservate();
strategy.appendField(locator, this, "totaleSommeBloccateRiservate", buffer, theTotaleSommeBloccateRiservate, (this.totaleSommeBloccateRiservate!= null));
}
{
BigDecimal theDisponibilita;
theDisponibilita = this.getDisponibilita();
strategy.appendField(locator, this, "disponibilita", buffer, theDisponibilita, (this.disponibilita!= null));
}
return buffer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy