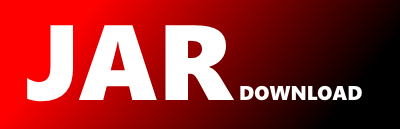
it.eng.spago.navigation.LightNavigator Maven / Gradle / Ivy
/**
Copyright 2004, 2007 Engineering Ingegneria Informatica S.p.A.
This file is part of Spago.
Spago is free software; you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation; either version 2.1 of the License, or
any later version.
Spago is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with Spago; if not, write to the Free Software
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
**/
package it.eng.spago.navigation;
import it.eng.spago.base.Constants;
import it.eng.spago.navigation.NavigationException;
import it.eng.spago.tracing.TracerSingleton;
import java.util.ArrayList;
public class LightNavigator {
// ArrayList used as stack (last-in-first-out)
private ArrayList list = new ArrayList();
/**
* Adds a MarkedRequest
element to the stack.
* @param markedRequest the MarkedRequest
object to be added to the stack
*/
public void add(MarkedRequest markedRequest) throws NavigationException {
if (markedRequest == null) {
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.CRITICAL, "LightNavigator: " +
"add: the MarkedRequest object at input is null.");
throw new NavigationException ("The MarkedRequest object at input is null.");
}
list.add(0, markedRequest);
}
/**
* Retrieves the MarkedRequest
object at the desired position in the stack and deletes
* the more recent MarkedRequest
objects present in the stack.
*
* @param i The int representing the position of the stack.
* @throws NavigationException
if the position at input is not present in the stack
*/
public MarkedRequest goBackToPosition(int i) throws NavigationException {
// Index i must be 0 < i < list.size()-1, the request at index 0 is the request for the current page
if (i < 0 || i > list.size() -1 ) {
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.CRITICAL, "LightNavigator: " +
"goBackToPosition: the position " + i +" is not present in the stack.");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG, "The requests stack is:\n" + this.toString());
throw new NavigationException ("Index of the required request is not correct.");
}
MarkedRequest markedRequest = (MarkedRequest) list.get(i);
for (int j = 0; j < i; j++) {
list.remove(0);
}
return markedRequest;
}
/**
* Deletes the more recent MarkedRequest
object in the stack and adds
* the MarkedRequest
passed at input in the first position of the stack.
*
* @param markedRequest the MarkedRequest
object that will replace the more recent MarkedRequest
in the stack
* @throws NavigationException
if teh MarkedRequest
object at input is null of if the stack is empty.
*/
public void replaceLast(MarkedRequest markedRequest) throws NavigationException {
if (markedRequest == null) {
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.CRITICAL, "LightNavigator: " +
"replaceLast: the MarkedRequest object at input is null.");
throw new NavigationException ("The MarkedRequest object at input is null.");
}
if (list.size() == 0) {
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.CRITICAL, "LightNavigator: " +
"replaceLast: the stack is empty: it is not possible to substitute the request.");
throw new NavigationException ("The stack is empty: it is not possible to substitute the request.");
}
// removes the most recent request
list.remove(0);
// adds the request at input in the first position
list.add(0, markedRequest);
}
/**
* Resets the stack.
*
*/
public void reset() {
list = new ArrayList();
}
/**
* Retrieves the more recent MarkedRequest
object in the stack with the mark passed at input
* and deletes the more recent MarkedRequest
objects present in the stack.
*
* @param mark The string mark of the desired MarkedRequest
object in the stack.
* @throws NavigationException
if there are no MarkedRequest
objects with the mark passed at input.
*/
public MarkedRequest goBackToMark(String mark) throws NavigationException{
if (mark == null) {
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.MAJOR, "LightNavigator: " +
"goBackToMark: the input mark is null.");
throw new NavigationException ("The input mark for navigation research cannot be null.");
}
MarkedRequest toReturn = null;
int i = 1;
while (i <= list.size()) {
MarkedRequest markedRequest = (MarkedRequest) list.get(i - 1);
if (mark.equalsIgnoreCase(markedRequest.getMark())) {
toReturn = markedRequest;
break;
}
i++;
}
if (toReturn == null) {
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.MAJOR, "LightNavigator: " +
"goBackToMark: Request with mark '" + mark + "' not found.");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG, "The requests stack is:\n" + this.toString());
throw new NavigationException ("Request with mark '" + mark + "' not found.");
}
for (int j = 0; j < i - 1; j++) {
list.remove(0);
}
return toReturn;
}
public String toString () {
String toReturn = "";
for (int i = 0 ; i < list.size(); i++) {
toReturn += "Position " + i + ":\n";
toReturn += list.get(i).toString();
toReturn += "\n-------------------------------------------\n";
}
return toReturn;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy