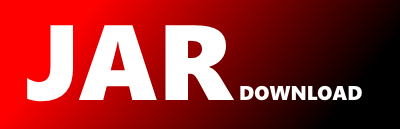
it.eng.spago.util.PortletUtilities Maven / Gradle / Ivy
/**
Copyright 2004, 2007 Engineering Ingegneria Informatica S.p.A.
This file is part of Spago.
Spago is free software; you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation; either version 2.1 of the License, or
any later version.
Spago is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with Spago; if not, write to the Free Software
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
**/
package it.eng.spago.util;
import it.eng.spago.base.Constants;
import it.eng.spago.base.PortletAccess;
import it.eng.spago.base.RequestContainer;
import it.eng.spago.base.RequestContainerPortletAccess;
import it.eng.spago.base.SourceBean;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.ResourceBundle;
import java.util.StringTokenizer;
import javax.portlet.ActionRequest;
import javax.portlet.PortletRequest;
import javax.portlet.PortletResponse;
import javax.portlet.PortletURL;
import javax.portlet.RenderRequest;
import javax.portlet.RenderResponse;
import javax.servlet.http.HttpServletRequest;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.portlet.PortletFileUpload;
/**
*
* @author Zoppello
*/
public class PortletUtilities {
/**
* Starting from the original URL and the request, creates a string representing the
* Portlet URL.
*
* @param aHttpServletRequest The request object at input
* @param originalURL The starting original URL
* @return A String representing the Portlet URL
*/
public static String createPortletURL(HttpServletRequest aHttpServletRequest, String originalURL){
RenderResponse renderResponse =(RenderResponse)aHttpServletRequest.getAttribute("javax.portlet.response");
RequestContainer requestContainer = RequestContainerPortletAccess.getRequestContainer(aHttpServletRequest);
PortletURL aPortletURL = renderResponse.createActionURL();
PortletTracer.debug(Constants.NOME_MODULO,
PortletUtilities.class.getName(),
"createPortletURL()",
"Original URL.... " + originalURL + "indexOf ? is " + originalURL.indexOf("?"));
String parameters = originalURL.substring(originalURL.indexOf("?")+1);
StringTokenizer st = new StringTokenizer(parameters, "&", false);
String parameterToken = null;
String parameterName = null;
String parameterValue = null;
while (st.hasMoreTokens()){
parameterToken = st.nextToken();
PortletTracer.debug(Constants.NOME_MODULO,
PortletUtilities.class.getName(),
"createPortletURL()","Parameter Token [" + parameterToken +"]");
parameterName = parameterToken.substring(0, parameterToken.indexOf("="));
parameterValue = parameterToken.substring(parameterToken.indexOf("=") + 1);
PortletTracer.debug(Constants.NOME_MODULO,
PortletUtilities.class.getName(),
"createPortletURL()","Parameter Name [" + parameterName +"]");
PortletTracer.debug(Constants.NOME_MODULO,
PortletUtilities.class.getName(),
"createPortletURL()","Parameter Value [" + parameterValue +"]");
aPortletURL.setParameter(parameterName, parameterValue);
}
return aPortletURL.toString();
}
/**
* Creates the particular portlet URL for a resource, given its path.
*
* @param aHttpServletRequest The request object at input
* @param resourceAbsolutePath The resource Absolute path
* @return The resource Portlet URL String
*/
public static String createPortletURLForResource(HttpServletRequest aHttpServletRequest, String resourcePath){
RenderResponse renderResponse =(RenderResponse)aHttpServletRequest.getAttribute("javax.portlet.response");
RenderRequest renderRequest =(RenderRequest)aHttpServletRequest.getAttribute("javax.portlet.request");
String resourceAbsPathFromPortletContex = null;
int idx = resourcePath.indexOf('/');
if ( idx > - 1){
PortletTracer.debug(Constants.NOME_MODULO, PortletUtilities.class.getName(), "createPortletURLForResource","URL ["+resourcePath+"] is relative !!!!" );
resourceAbsPathFromPortletContex = resourcePath.substring(idx);
PortletTracer.debug(Constants.NOME_MODULO, PortletUtilities.class.getName(), "createPortletURLForResource", "Absolute URL From Portlet Context ["+resourceAbsPathFromPortletContex+"]");
}
String urlToReturn = renderResponse.encodeURL(renderRequest.getContextPath() + resourceAbsPathFromPortletContex);
return urlToReturn;
}
/**
* Gets the PortletRequest
object.
* @return The portlet request object
*/
public static PortletRequest getPortletRequest(){
return PortletAccess.getPortletRequest();
}
/**
* Gets the PortletResponse
object.
* @return The portlet response object
*/
public static PortletResponse getPortletResponse(){
return PortletAccess.getPortletResponse();
}
/**
* Gets the service request from a Multipart Portlet Request. This method creates a
* new file upload handler, then parses the request and processes the new uploaded items.
* In this way a new uploaded file is obtained, which is put into the serviceRequest
* object.
*
* @param portletRequest The input portlet request
* @return The serviceRequest
SourceBean containing the uploaded file.
*/
public static SourceBean getServiceRequestFromMultipartPortletRequest(PortletRequest portletRequest){
SourceBean serviceRequest = null;
try{
serviceRequest = new SourceBean("SERVICEREQUEST");
DiskFileItemFactory factory = new DiskFileItemFactory();
// Create a new file upload handler
PortletFileUpload upload = new PortletFileUpload(factory);
// Parse the request
List /* FileItem */ items = upload.parseRequest((ActionRequest)portletRequest);
// Process the uploaded items
Iterator iter = items.iterator();
while (iter.hasNext()) {
FileItem item = (FileItem) iter.next();
if (item.isFormField()) {
serviceRequest.setAttribute(item.getFieldName(), item.getString());
} else {
UploadedFile uploadedFile = new UploadedFile();
uploadedFile.setFileContent(item.get());
uploadedFile.setFieldNameInForm(item.getFieldName());
uploadedFile.setSizeInBytes(item.getSize());
uploadedFile.setFileName(GeneralUtilities.getRelativeFileNames(item.getName()));
serviceRequest.setAttribute("UPLOADED_FILE", uploadedFile);
}
}
}catch(Exception e){
PortletTracer.major(Constants.NOME_MODULO, PortletUtilities.class.getName(),"getServiceRequestFromMultipartPortletRequets","Cannot parse multipart request", e);
}
return serviceRequest;
}
/**
* Gets the first uploaded file from a portlet request. This method creates a new file upload handler,
* parses the request, processes the uploaded items and then returns the first file as an
* UploadedFile
object.
* @param portletRequest The input portlet request
* @return The first uploaded file object.
*/
public static UploadedFile getFirstUploadedFile(PortletRequest portletRequest){
UploadedFile uploadedFile = null;
try{
DiskFileItemFactory factory = new DiskFileItemFactory();
// Create a new file upload handler
PortletFileUpload upload = new PortletFileUpload(factory);
// Parse the request
List /* FileItem */ items = upload.parseRequest((ActionRequest)portletRequest);
// Process the uploaded items
Iterator iter = items.iterator();
boolean endLoop = false;
while (iter.hasNext() && !endLoop) {
FileItem item = (FileItem) iter.next();
if (item.isFormField()) {
//serviceRequest.setAttribute(item.getFieldName(), item.getString());
} else {
uploadedFile = new UploadedFile();
uploadedFile.setFileContent(item.get());
uploadedFile.setFieldNameInForm(item.getFieldName());
uploadedFile.setSizeInBytes(item.getSize());
uploadedFile.setFileName(item.getName());
endLoop = true;
}
}
}catch(Exception e){
PortletTracer.major(Constants.NOME_MODULO,PortletUtilities.class.getName(),"getServiceRequestFromMultipartPortletRequets","Cannot parse multipart request", e);
}
return uploadedFile;
}
/**
* Cleans a string from spaces and tabulation characters.
*
* @param original The input string
* @return The cleaned string
*/
public static String cleanString(String original){
StringBuffer sb = new StringBuffer();
char[] arrayChar = original.toCharArray();
for (int i=0; i < arrayChar.length; i++){
if ((arrayChar[i] == '\n')
|| (arrayChar[i] == '\t')
|| (arrayChar[i] == '\r')){
}else{
sb.append(arrayChar[i]);
}
}
return sb.toString().trim();
}
/**
* Gets a localized message given its code and bundle
* information. If there isn't any message matching to these infromation, a
* warning is traced.
*
* @param code The message's code string
* @param bundle The message's bundel string
* @return A string containing the message
*/
public static String getMessage(String code, String bundle) {
// get the locale and the language of the portal
Locale portalLocale = PortletAccess.getPortalLocale();
// Locale portalLocale = (Locale)portletRequest.getPortletSession().getAttribute("LOCALE");
String portalLang = portalLocale.getLanguage();
String portalCountry = portalLocale.getCountry();
Locale locale = new Locale(portalLang, portalCountry);
ResourceBundle messages = null;
try{
messages = ResourceBundle.getBundle(bundle, locale);
}catch(Exception e){
messages = ResourceBundle.getBundle("messages");
}
if (messages == null) {
return null;
}
String message = code;
try {
message = messages.getString(code);
} catch (Exception ex) {
PortletTracer.warning(Constants.NOME_MODULO, PortletUtilities.class.getName(),
"getMessage", "code [" + code + "] not found ");
}
return message;
} // public String getMessage(String code)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy