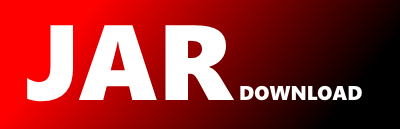
it.eng.spago.security.xmlauthorizations.XMLAuthorizationsRepository Maven / Gradle / Ivy
/**
Copyright 2004, 2007 Engineering Ingegneria Informatica S.p.A.
This file is part of Spago.
Spago is free software; you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation; either version 2.1 of the License, or
any later version.
Spago is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with Spago; if not, write to the Free Software
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
**/
package it.eng.spago.security.xmlauthorizations;
import it.eng.spago.base.Constants;
import it.eng.spago.base.SourceBean;
import it.eng.spago.configuration.ConfigSingleton;
import it.eng.spago.error.EMFErrorSeverity;
import it.eng.spago.error.EMFInternalError;
import it.eng.spago.tracing.TracerSingleton;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import sun.misc.BASE64Decoder;
/**
* DATE CONTRIBUTOR/DEVELOPER NOTE
* 13-12-2004 Butano SOurceBean restituisce ArrayList
*
**/
/**
* @author zoppello
*
* To change this generated comment edit the template variable "typecomment":
* Window>Preferences>Java>Templates.
* To enable and disable the creation of type comments go to
* Window>Preferences>Java>Code Generation.
*/
public class XMLAuthorizationsRepository {
private static XMLAuthorizationsRepository _instance = null;
private boolean _defaultAuthorization = false;
private Map _users = null;
private Map _roles = null;
private Map _functionalities = null;
private Map _resources = null;
private Map _behaviours = null;
private Map _priviledges = null;
private Map _userResources = null;
private XMLAuthorizationsRepository() {
super();
_defaultAuthorization = false;
_users = new HashMap();
_roles = new HashMap();
_functionalities = new HashMap();
_resources = new HashMap();
_behaviours = new HashMap();
_priviledges = new HashMap();
_userResources = new HashMap();
load();
} // private XMLAuthorizationsRepository()
public static XMLAuthorizationsRepository getInstance() {
if (_instance == null) {
synchronized(XMLAuthorizationsRepository.class) {
if (_instance == null) {
try {
_instance = new XMLAuthorizationsRepository();
} // try
catch (Exception ex) {
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.CRITICAL,
"XMLAuthorizationsRepository::getInstance: ", ex);
} // catch(Exception ex)
} // if (_instance == null)
} // synchronized(ConfigSingleton.class)
} // if (_instance == null)
return _instance;
} // public static ConfigSingleton getInstance()
private void load() {
SourceBean authorizations = (SourceBean)ConfigSingleton.getInstance().getAttribute("AUTHORIZATIONS");
if (authorizations == null) {
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.WARNING,
"XMLAuthorizationsRepository::load: autorizzazioni non trovate !");
return;
} // if (authorizations == null)
String defaultAuthorization = (String)authorizations.getAttribute("default");
_defaultAuthorization = ((defaultAuthorization != null) && (defaultAuthorization.equalsIgnoreCase("TRUE")));
// Load Users
List usersList = authorizations.getAttributeAsList("ENTITIES.USERS.USER");
Iterator it = usersList.iterator();
while (it.hasNext()) {
SourceBean user = (SourceBean)it.next();
String userID = (String)user.getAttribute("userID");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG,
"XMLAuthorizationsRepository::load: userID [" + userID + "]");
_users.put(userID.toUpperCase(), user);
} // while (it.hasNext())
//Load Roles
List rolesList = authorizations.getAttributeAsList("ENTITIES.ROLES.ROLE");
it = rolesList.iterator();
while (it.hasNext()) {
SourceBean role = (SourceBean)it.next();
String roleName = (String)role.getAttribute("roleName");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG,
"XMLAuthorizationsRepository::load: roleName [" + roleName + "]");
_roles.put(roleName.toUpperCase(), role);
} // while (it.hasNext())
// Load Functionalities
List functionalitiesList = authorizations.getAttributeAsList("ENTITIES.FUNCTIONALITIES.FUNCTIONALITY");
it = functionalitiesList.iterator();
while (it.hasNext()) {
SourceBean functionality = (SourceBean)it.next();
String functionalityName = (String)functionality.getAttribute("functionalityName");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG,
"XMLAuthorizationsRepository::load: functionalityName [" + functionalityName + "]");
_functionalities.put(functionalityName.toUpperCase(), functionality);
} // while (it.hasNext())
// Load Resources
List resourcesList = authorizations.getAttributeAsList("ENTITIES.RESOURCES.RESOURCE");
it = resourcesList.iterator();
while (it.hasNext()) {
SourceBean resource = (SourceBean)it.next();
String resourceName = (String)resource.getAttribute("resourceName");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG,
"XMLAuthorizationsRepository::load: resourceName [" + resourceName + "]");
_resources.put(resourceName.toUpperCase(), resource);
} // while (it.hasNext())
// Load Behaviours
List behaviourList = authorizations.getAttributeAsList("RELATIONS.BEHAVIOURS.BEHAVIOUR");
it = behaviourList.iterator();
while (it.hasNext()) {
SourceBean behaviour = (SourceBean)it.next();
String userID = (String)behaviour.getAttribute("userID");
String roleName = (String)behaviour.getAttribute("roleName");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG,
"XMLAuthorizationsRepository::load: associazione userID [" + userID + "] roleName [" + roleName + "]");
Collection roles = (Collection)_behaviours.get(userID.toUpperCase());
if (roles != null) {
if (!(roles.contains(roleName.toUpperCase())))
roles.add(roleName.toUpperCase());
} // if (roles != null)
else {
roles = new ArrayList();
roles.add(roleName.toUpperCase());
_behaviours.put(userID.toUpperCase(), roles);
} // if (roles != null) else
} // while (it.hasNext())
// Load privildeges
List priviledgeList = authorizations.getAttributeAsList("RELATIONS.PRIVILEDGES.PRIVILEDGE");
it = priviledgeList.iterator();
while (it.hasNext()) {
SourceBean privilege = (SourceBean)it.next();
String roleName = (String)privilege.getAttribute("roleName");
String functionalityName = (String)privilege.getAttribute("functionalityName");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG,
"XMLAuthorizationsRepository::load: associazione roleName [" + roleName + "] functionalityName [" +
functionalityName + "]");
Collection functionalities = (Collection)_priviledges.get(roleName.toUpperCase());
if (functionalities != null) {
if (!(functionalities.contains(functionalityName.toUpperCase())))
functionalities.add(functionalityName.toUpperCase());
} // if (functionalities != null)
else {
functionalities = new ArrayList();
functionalities.add(functionalityName.toUpperCase());
_priviledges.put(roleName.toUpperCase(), functionalities);
} // if (functionalities != null) else
} // while (it.hasNext())
// Load userResources
List userResourcesList = authorizations.getAttributeAsList("RELATIONS.USER-RESOURCES.USER-RESOURCE");
it = userResourcesList.iterator();
while (it.hasNext()) {
SourceBean userResource = (SourceBean)it.next();
String userID = (String)userResource.getAttribute("userID");
String resourceName = (String)userResource.getAttribute("resourceName");
TracerSingleton.log(Constants.NOME_MODULO, TracerSingleton.DEBUG,
"XMLAuthorizationsRepository::load: associazione userID [" + userID + "] resourceName [" + resourceName + "]");
Collection resources = (Collection)_userResources.get(userID.toUpperCase());
if (resources != null) {
if (!(resources.contains(resourceName.toUpperCase())))
resources.add(resourceName.toUpperCase());
} // if (resources != null)
else {
resources = new ArrayList();
resources.add(resourceName.toUpperCase());
_userResources.put(userID.toUpperCase(), resources);
} // if (resources != null) else
} // while (it.hasNext())
} // private void load()
public boolean getDefaultAuthorization() {
return _defaultAuthorization;
} // public boolean getDefaultAuthorization()
public void authenticateUser(String userID, String passwordToVerify) throws EMFInternalError {
if (userID == null)
throw new EMFInternalError(EMFErrorSeverity.ERROR, "Autenticazione fallita, utente [" + userID + "] non valido");
if (passwordToVerify == null)
throw new EMFInternalError(EMFErrorSeverity.ERROR, "Autenticazione fallita, password non valida");
SourceBean user = (SourceBean)_users.get(userID.toUpperCase());
if (user == null)
throw new EMFInternalError(EMFErrorSeverity.ERROR, "Autenticazione fallita, utente non censito");
String passInFileCrypted = (String)user.getAttribute("password");
byte[] passwordToVerifyByteArray = new byte[passwordToVerify.length()];
try {
passwordToVerifyByteArray = passwordToVerify.getBytes("UTF-8");
} // try
catch (UnsupportedEncodingException uee) {
throw new EMFInternalError(EMFErrorSeverity.ERROR, "Autenticazione fallita", uee);
} // catch (UnsupportedEncodingException uee)
MessageDigest algorithm = null;
try {
algorithm = MessageDigest.getInstance("SHA-1");
} // try
catch (NoSuchAlgorithmException nsae) {
throw new EMFInternalError(EMFErrorSeverity.ERROR, "Autenticazione fallita", nsae);
} // catch (NoSuchAlgorithmException nsae)
algorithm.reset();
algorithm.update(passwordToVerifyByteArray);
byte[] digestPasswordInserted = algorithm.digest();
byte[] digestRealPassword = null;
try {
digestRealPassword = new BASE64Decoder().decodeBuffer(passInFileCrypted);
} // try
catch (IOException ioe) {
throw new EMFInternalError(EMFErrorSeverity.ERROR, "Autenticazione fallita", ioe);
} // catch (IOException ioe)
if (digestPasswordInserted.length != digestRealPassword.length) {
throw new EMFInternalError(EMFErrorSeverity.ERROR, "Autenticazione fallita, password errata");
} // if (digestPasswordInserted.length != digestRealPassword.length)
for (int i = 0; i < digestPasswordInserted.length; i++)
if (digestPasswordInserted[i] != digestRealPassword[i])
throw new EMFInternalError(EMFErrorSeverity.ERROR, "Autenticazione fallita, password errata");
// Se sono arrivato qui i due digest sono uguali
// autenticazione avvenuta correttamente
} // public void authenticateUser(String userID, String passwordToVerify) throws EMFInternalError
public SourceBean getUser(String userID) {
return (SourceBean)_users.get(userID.toUpperCase());
} // public SourceBean getUser(String userID)
public Collection getUserRoles(String userID) {
Collection userRoles = (Collection)_behaviours.get(userID.toUpperCase());
if (userRoles == null)
userRoles = new ArrayList();
return userRoles;
} // public Collection getUserRoles(String userID)
public Collection getUserFunctionalities(String userID) {
Collection result = new ArrayList();
Collection userRoles = (Collection)_behaviours.get(userID.toUpperCase());
if (userRoles == null)
userRoles = new ArrayList();
Iterator userRole = userRoles.iterator();
while (userRole.hasNext()) {
Collection roleFunctionalities = (Collection)_priviledges.get(((String)userRole.next()).toUpperCase());
if (roleFunctionalities == null)
roleFunctionalities = new ArrayList();
Iterator roleFunctionality = roleFunctionalities.iterator();
while (roleFunctionality.hasNext()) {
String functionality = (String)roleFunctionality.next();
if (!(result.contains(functionality.toUpperCase())))
result.add(functionality.toUpperCase());
} // while (roleFunctionality.hasNext())
} // while (it.hasNext())
return result;
} // public Collection getUserFunctionalities(String userID)
public Collection getRoleFunctionalities(String roleName) {
Collection roleFunctionalities = (Collection)_priviledges.get(roleName.toUpperCase());
if (roleFunctionalities == null)
roleFunctionalities = new ArrayList();
return roleFunctionalities;
} // private Collection getRoleFunctionalities(String roleName)
public Collection getUserResources(String userID) {
Collection userResources = (Collection)_userResources.get(userID.toUpperCase());
if (userResources == null)
userResources = new ArrayList();
return userResources;
} // public Collection getUserResources(String userID)
} // public class XMLAuthorizationsRepository
© 2015 - 2025 Weber Informatics LLC | Privacy Policy