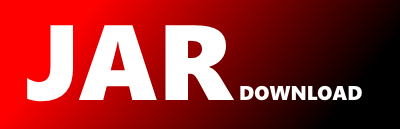
it.mice.voila.runtime.util.DateTimeUtils Maven / Gradle / Ivy
package it.mice.voila.runtime.util;
import java.sql.Timestamp;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateTimeUtils {
private static SimpleDateFormat italianDateFormat = new SimpleDateFormat("dd/MM/yyyy");
private static SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
private static SimpleDateFormat dateTimeFormat = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss");
private static SimpleDateFormat standardDateTimeFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.S");
private static SimpleDateFormat standardDateTimeFormatNoMillisecond = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
private static SimpleDateFormat americanDateTimeFormat = new SimpleDateFormat("MM/dd/yyyy HH:mm:ss");
public static Timestamp getCurrentDateAsTimestamp() {
return getDateStringAsTimestamp(getCurrentDateAsString());
}
public static Timestamp getCurrentDateTimeAsTimestamp() {
return getDateTimeStringAsTimestamp(getCurrentDateTimeAsString());
}
public static Timestamp getDateStringAsTimestamp(String date) {
Date theDate;
try {
theDate = new SimpleDateFormat(dateFormat.toPattern()).parse(date);
} catch (ParseException e) {
throw new RuntimeException(e);
}
return getTimestampFromLong((theDate.getTime()));
}
public static Timestamp getDateTimeStringAsTimestamp(String date) {
Date theDate;
try {
theDate = new SimpleDateFormat(dateTimeFormat.toPattern()).parse(date);
} catch (ParseException e) {
throw new RuntimeException(e);
}
return getTimestampFromLong((theDate.getTime()));
}
public static Timestamp getTimestampFromLong(long time) {
return new java.sql.Timestamp(time);
}
public static Date getCurrentDate() {
return new Date();
}
public static String getCurrentDateAsString() {
return new SimpleDateFormat(dateFormat.toPattern()).format(getCurrentDate());
}
public static String getCurrentDateTimeAsString() {
return new SimpleDateFormat(dateTimeFormat.toPattern()).format(getCurrentDate());
}
public static Date parseStandardDate(String date) {
try {
return new SimpleDateFormat(standardDateTimeFormat.toPattern()).parse(date);
} catch (ParseException e) {
return parseStandardDateWithoutMillisecond(date);
}
}
public static Date parseStandardDateWithoutMillisecond(String date) {
try {
return new SimpleDateFormat(standardDateTimeFormatNoMillisecond.toPattern()).parse(date);
} catch (ParseException e) {
throw new RuntimeException("Unable to parse given date string: " + date);
}
}
public static String formatStandardDate(Date date) {
try {
return new SimpleDateFormat(standardDateTimeFormat.toPattern()).format(date);
} catch (Exception e) {
throw new RuntimeException("Unable to format given date string: " + date);
}
}
public static String formatStandardDateWithoutMillisecond(Date date) {
try {
return new SimpleDateFormat(standardDateTimeFormatNoMillisecond.toPattern()).format(date);
} catch (Exception e) {
throw new RuntimeException("Unable to format given date string: " + date);
}
}
public static String formatItalianDate(Date date) {
try {
return new SimpleDateFormat(italianDateFormat.toPattern()).format(date);
} catch (Exception e) {
throw new RuntimeException("Unable to format given date string: " + date);
}
}
public static Date parseItalianDate(String date) {
try {
return new SimpleDateFormat(italianDateFormat.toPattern()).parse(date);
} catch (ParseException e) {
throw new RuntimeException("Unable to parse given date string: " + date);
}
}
public static Date parseAmericanDate(String date) {
try {
return new SimpleDateFormat(americanDateTimeFormat.toPattern()).parse(date);
} catch (ParseException e) {
date += " 00:00:00";
try {
return new SimpleDateFormat(americanDateTimeFormat.toPattern()).parse(date);
} catch (ParseException e2) {
throw new RuntimeException("Unable to parse given date string: " + date);
}
}
}
public static String formatAmericanDate(Date date) {
try {
return new SimpleDateFormat(americanDateTimeFormat.toPattern()).format(date);
} catch (Exception e) {
throw new RuntimeException("Unable to format given date string: " + date);
}
}
public static Date buildDateTime(Date date, String time) {
String workdate = new SimpleDateFormat(dateFormat.toPattern()).format(date);
workdate += " " + time + ":00.0";
try {
return new SimpleDateFormat(standardDateTimeFormat.toPattern()).parse(workdate);
} catch (ParseException e) {
throw new RuntimeException("Unable to parse given date string: " + workdate);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy