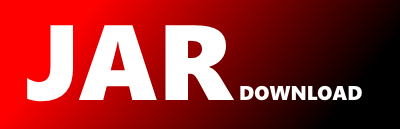
it.mice.voila.runtime.jquery.wdcalendar.CalendarEventBean Maven / Gradle / Ivy
The newest version!
package it.mice.voila.runtime.jquery.wdcalendar;
import it.mice.voila.runtime.util.DateTimeUtils;
import java.io.Serializable;
import java.util.Date;
/**
* Base calendar event bean for WdCalendar jquery widget.
*/
public class CalendarEventBean implements Serializable {
/**
* serial version UID
*/
private static final long serialVersionUID = -7114857166713008599L;
public static final int THEME_STYLE_GRAY = 22;
public static final int THEME_STYLE_RED = 1;
public static final int THEME_STYLE_PINK = 2;
public static final int THEME_STYLE_MAGENTA = 3;
public static final int THEME_STYLE_SLATE_BLUE = 4;
public static final int THEME_STYLE_BLU = 5;
public static final int THEME_STYLE_BLU2 = 6;
public static final int THEME_STYLE_SEAGREEN = 7;
public static final int THEME_STYLE_GREEN = 8;
public static final int THEME_STYLE_DARK_GREEN = 9;
public static final int THEME_STYLE_LAWN_GREEN = 10;
public static final int THEME_STYLE_OLIVE = 11;
public static final int THEME_STYLE_YELLOW = 12;
public static final int THEME_STYLE_ORANGE = 13;
public static final int THEME_STYLE_ORANGE_RED = 14;
public static final int THEME_STYLE_ROSY_BROWN = 15;
public static final int THEME_STYLE_MEDIUM_PURPLE = 16;
public static final int THEME_STYLE_SLATE_GRAY = 17;
public static final int THEME_STYLE_LIGHT_BLUE = 18;
public static final int THEME_STYLE_MEDIUM_SEAGREEN = 19;
public static final int THEME_STYLE_LIGHT_OLIVE = 20;
public static final int THEME_STYLE_PERU = 21;
/**
* Unique id for the event.
*/
private int id;
/**
* Event start date-time.
*/
private String startDate;
/**
* Event end date-time.
*/
private String endDate;
/**
* Is an all day event?
*/
private boolean allDayEvent;
/**
* Is a cross day event?
*/
private boolean crossDayEvent;
/**
* Is a recurring event?
*/
private boolean recurringEvent;
/**
* Theme style code (see static THEME_STYLE).
*/
private int themeStyle;
/**
* Is the event updatable?
*/
private boolean updatableEvent;
/**
* Location for the event.
*/
private String location;
/**
* Partecipants to the event.
*/
private String partecipant;
public CalendarEventBean(int id, Date startDate, Date endDate, boolean allDayEvent, boolean crossDayEvent, boolean recurringEvent, int themeStyle, boolean updatableEvent, String location, String partecipant) {
this.id = id;
this.startDate = DateTimeUtils.formatAmericanDate(startDate);
this.endDate = DateTimeUtils.formatAmericanDate(endDate);
this.allDayEvent = allDayEvent;
this.crossDayEvent = crossDayEvent;
this.recurringEvent = recurringEvent;
this.themeStyle = themeStyle;
this.updatableEvent = updatableEvent;
this.location = location;
this.partecipant = partecipant;
}
/**
* @return the id
*/
public int getId() {
return id;
}
/**
* @param id the id to set
*/
public void setId(int id) {
this.id = id;
}
/**
* @return the startDate
*/
public String getStartDate() {
return startDate;
}
/**
* @param startDate the startDate to set
*/
public void setStartDate(String startDate) {
this.startDate = startDate;
}
/**
* @return the endDate
*/
public String getEndDate() {
return endDate;
}
/**
* @param endDate the endDate to set
*/
public void setEndDate(String endDate) {
this.endDate = endDate;
}
/**
* @return the allDayEvent
*/
public boolean isAllDayEvent() {
return allDayEvent;
}
/**
* @param allDayEvent the allDayEvent to set
*/
public void setAllDayEvent(boolean allDayEvent) {
this.allDayEvent = allDayEvent;
}
/**
* @return the crossDayEvent
*/
public boolean isCrossDayEvent() {
return crossDayEvent;
}
/**
* @param crossDayEvent the crossDayEvent to set
*/
public void setCrossDayEvent(boolean crossDayEvent) {
this.crossDayEvent = crossDayEvent;
}
/**
* @return the recurringEvent
*/
public boolean isRecurringEvent() {
return recurringEvent;
}
/**
* @param recurringEvent the recurringEvent to set
*/
public void setRecurringEvent(boolean recurringEvent) {
this.recurringEvent = recurringEvent;
}
/**
* @return the themeStyle
*/
public int getThemeStyle() {
return themeStyle;
}
/**
* @param themeStyle the themeStyle to set
*/
public void setThemeStyle(int themeStyle) {
this.themeStyle = themeStyle;
}
/**
* @return the updatableEvent
*/
public boolean isUpdatableEvent() {
return updatableEvent;
}
/**
* @param updatableEvent the updatableEvent to set
*/
public void setUpdatableEvent(boolean updatableEvent) {
this.updatableEvent = updatableEvent;
}
/**
* @return the location
*/
public String getLocation() {
return location;
}
/**
* @param location the location to set
*/
public void setLocation(String location) {
this.location = location;
}
/**
* @return the partecipant
*/
public String getPartecipant() {
return partecipant;
}
/**
* @param partecipant the partecipant to set
*/
public void setPartecipant(String partecipant) {
this.partecipant = partecipant;
}
public String getTitle() {
return "Event no. " + getId();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy