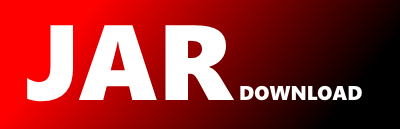
it.ozimov.cirneco.hamcrest.CirnecoMatchersJ7 Maven / Gradle / Ivy
package it.ozimov.cirneco.hamcrest;
import com.google.common.base.Equivalence;
import com.google.common.base.Optional;
import com.google.common.collect.Multimap;
import com.google.common.collect.Multiset;
import it.ozimov.cirneco.hamcrest.base.IsBetween;
import it.ozimov.cirneco.hamcrest.base.IsBetweenInclusive;
import it.ozimov.cirneco.hamcrest.base.IsBetweenLowerBoundInclusive;
import it.ozimov.cirneco.hamcrest.base.IsBetweenUpperBoundInclusive;
import it.ozimov.cirneco.hamcrest.base.IsEmptyGuavaOptional;
import it.ozimov.cirneco.hamcrest.base.IsEquivalent;
import it.ozimov.cirneco.hamcrest.base.IsSame;
import it.ozimov.cirneco.hamcrest.date.IsDate;
import it.ozimov.cirneco.hamcrest.date.IsDateInDay;
import it.ozimov.cirneco.hamcrest.date.IsDateInLeapYear;
import it.ozimov.cirneco.hamcrest.date.IsDateInMonth;
import it.ozimov.cirneco.hamcrest.date.IsDateInWeekOfYear;
import it.ozimov.cirneco.hamcrest.date.IsDateWithTime;
import it.ozimov.cirneco.hamcrest.date.utils.ClockPeriod;
import it.ozimov.cirneco.hamcrest.iterable.IsEmptyIterable;
import it.ozimov.cirneco.hamcrest.iterable.IsIterableWithDistinctElements;
import it.ozimov.cirneco.hamcrest.iterable.IsIterableWithSize;
import it.ozimov.cirneco.hamcrest.iterable.IsMultisetElementWithCount;
import it.ozimov.cirneco.hamcrest.iterable.IsSortedIterable;
import it.ozimov.cirneco.hamcrest.iterable.IsSortedIterableWithComparator;
import it.ozimov.cirneco.hamcrest.map.IsMapWithSameKeySet;
import it.ozimov.cirneco.hamcrest.map.IsMultimapKeyWithCollectionSize;
import it.ozimov.cirneco.hamcrest.map.IsMultimapWithKeySet;
import it.ozimov.cirneco.hamcrest.map.IsMultimapWithKeySetSize;
import it.ozimov.cirneco.hamcrest.number.IsInfinity;
import it.ozimov.cirneco.hamcrest.number.IsNegative;
import it.ozimov.cirneco.hamcrest.number.IsNegativeInfinity;
import it.ozimov.cirneco.hamcrest.number.IsNotANumber;
import it.ozimov.cirneco.hamcrest.number.IsPositive;
import it.ozimov.cirneco.hamcrest.number.IsPositiveInfinity;
import it.ozimov.cirneco.hamcrest.web.IsEmail;
import org.hamcrest.Matcher;
import org.hamcrest.Matchers;
import java.util.Collection;
import java.util.Comparator;
import java.util.Date;
import java.util.Map;
import java.util.Set;
/**
* The {@code CirnecoMatchersJ7} class groups all the matchers
* introduced by Cirneco's Hamcrest extension for Java 7.
* Suggested use would be to import all the static methods of this class in a unit tes.
*
* @since version 0.1 for JDK7
*/
public class CirnecoMatchersJ7 {
//BASE
/**
* Creates a matcher for {@code T}s that matches when the compareTo()
method returns
* a value between from
and to
, both excluded.
*
* For example:
*
assertThat(10, between(10, 11))
* will return false
.
*/
public static > Matcher between(final T from, final T to) {
return IsBetween.between(from, to);
}
/**
* Creates a matcher for {@code T}s that matches when the compareTo()
method returns
* a value between from
and to
, both included.
*
* For example:
*
assertThat(10, betweenInclusive(10, 11))
* will return true
.
*/
public static > Matcher betweenInclusive(final T from, final T to) {
return IsBetweenInclusive.betweenInclusive(from, to);
}
/**
* Creates a matcher for {@code T}s that matches when the compareTo()
method returns
* a value between from
and to
, both included.
*
* For example:
*
assertThat(10, betweenLowerBoundInclusive(10, 11))
* will return true
.
* while:
* assertThat(11, betweenLowerBoundInclusive(10, 11))
* will return false
.
*/
public static > Matcher betweenLowerBoundInclusive(final T from, final T to) {
return IsBetweenLowerBoundInclusive.betweenLowerBoundInclusive(from, to);
}
/**
* Creates a matcher for {@code T}s that matches when the compareTo()
method returns
* a value between from
and to
, both included.
*
* For example:
*
assertThat(11, betweenUpperBoundInclusive(10, 11))
* will return true
.
* while:
* assertThat(10, betweenUpperBoundInclusive(10, 11))
* will return false
.
*/
public static > Matcher betweenUpperBoundInclusive(final T from, final T to) {
return IsBetweenUpperBoundInclusive.betweenUpperBoundInclusive(from, to);
}
/**
* Creates a matcher that matches when the examined {@linkplain Optional}
* contains no object.
*/
public static Matcher emptyGuavaOptional() {
return IsEmptyGuavaOptional.emptyOptional();
}
/**
* Creates a matcher that matches when the examined object of type T
* is equivalent to the specified comparison
object according to
* the provided {@linkplain Equivalence}.
*
* Observe that the {@linkplain Equivalence} can deal with nulls.
*/
public static Matcher equivalentTo(final T expected, final Equivalence equivalence) {
return IsEquivalent.equivalentTo(expected, equivalence);
}
/**
* Creates a matcher that matches only when the examined {@linkplain Object} is the same instance as
* the provided target
{@linkplain Object}.
*/
public static Matcher sameInstance(final Object target) {
return IsSame.sameInstance(target);
}
/**
* Creates a matcher of a {@link Comparable} object that matches when the examined object is
* after the given value
, as reported by the compareTo
method of the
* examined object.
*
* E.g.:
*
* Date past;
* Date now;
* assertThat(now, after(past));
*
*
*
* The matcher renames the Hamcrest matcher obtained with {@linkplain org.hamcrest.Matchers#greaterThan(Comparable)}.
*/
public static > Matcher after(final T value) {
return Matchers.greaterThan(value);
}
/**
* Creates a matcher of {@link Comparable} object that matches when the examined object is
* after or equal with respect to object value
, as reported by the compareTo
method
* of the examined object.
*
* E.g.:
*
* Date past;
* Date now;
* assertThat(now, afterOrEqual(now));
* assertThat(now, afterOrEqual(past));
*
*
*
* The matcher renames the Hamcrest matcher obtained with {@linkplain org.hamcrest.Matchers#greaterThanOrEqualTo(Comparable)}.
*/
public static > Matcher afterOrEqual(final T value) {
return Matchers.greaterThanOrEqualTo(value);
}
/**
* Creates a matcher of {@link Comparable} object that matches when the examined object is
* before the given value
, as reported by the compareTo
method of the
* examined object.
*
* E.g.:
*
* Date past;
* Date now;
* assertThat(past, before(now));
*
*
*
* The matcher renames the Hamcrest matcher obtained with {@linkplain org.hamcrest.Matchers#lessThan(Comparable)}.
*/
public static > Matcher before(final T value) {
return Matchers.lessThan(value);
}
/**
* Creates a matcher of {@link Comparable} object that matches when the examined object is
* before or equal with respect to object value
, as reported by the compareTo
method
* of the examined object.
*
* E.g.:
*
* Date past;
* Date now;
* assertThat(now, beforeOrEqual(now));
* assertThat(past, beforeOrEqual(now));
*
*
*
* The matcher renames the Hamcrest matcher obtained with {@linkplain org.hamcrest.Matchers#lessThanOrEqualTo(Comparable)}.
*/
public static > Matcher beforeOrEqual(final T value) {
return Matchers.lessThanOrEqualTo(value);
}
//DATE
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given year
.
*/
public static Matcher hasYear(final int year) {
return IsDate.hasYear(year);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given id
.
*/
public static Matcher hasMonth(final Integer month) {
return IsDate.hasMonth(month);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given day
.
*/
public static Matcher hasDay(final Integer day) {
return IsDate.hasDay(day);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values year
and id
.
*/
public static Matcher hasYearAndMonth(final int year, final int month) {
return IsDate.hasYearAndMonth(year, month);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values year
, id
and
* day
.
*/
public static Matcher hasYearMonthAndDay(final Integer year,
final Integer month, final Integer day) {
return IsDate.hasYearMonthAndDay(year, month, day);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given hour
in a 24 hours clock period.
*/
public static Matcher hasHour(final int hour) {
return IsDateWithTime.hasHour(hour);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values hour
and ClockPeriod
(e.g. AM).
*/
public static Matcher hasHour(final int hour, final ClockPeriod clockPeriod) {
return IsDateWithTime.hasHour(hour, clockPeriod);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given minute
.
*/
public static Matcher hasMinute(final Integer minute) {
return IsDateWithTime.hasMinute(minute);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given sec
.
*/
public static Matcher hasSecond(final Integer second) {
return IsDateWithTime.hasSecond(second);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given millis
.
*/
public static Matcher hasMillisecond(final Integer millisecond) {
return IsDateWithTime.hasMillisecond(millisecond);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values hour
in a 24 hours clock period and minute
.
*/
public static Matcher hasHourAndMin(final int hour, final int minute) {
return IsDateWithTime.hasHourAndMin(hour, minute);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values hour
, ClockPeriod
(e.g. AM) and minute
.
*/
public static Matcher hasHourAndMin(final int hour, final ClockPeriod clockPeriod, final int minute) {
return IsDateWithTime.hasHourAndMin(hour, clockPeriod, minute);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values hour
in a 24 hours clock period, minute
and
* sec
.
*/
public static Matcher hasHourMinAndSec(final Integer hour,
final Integer minute, final Integer second) {
return IsDateWithTime.hasHourMinAndSec(hour, minute, second);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values hour
, ClockPeriod
(e.g. AM), minute
and
* sec
.
*/
public static Matcher hasHourMinAndSec(final Integer hour, final ClockPeriod clockPeriod,
final Integer minute, final Integer second) {
return IsDateWithTime.hasHourMinAndSec(hour, clockPeriod, minute, second);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values hour
in a 24 hours clock period, minute
,
* sec
and millis
.
*/
public static Matcher hasHourMinSecAndMillis(final Integer hour,
final Integer minute, final Integer second, final Integer millisecond) {
return IsDateWithTime.hasHourMinSecAndMillis(hour, minute, second, millisecond);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* has the given values hour
, ClockPeriod
(e.g. AM), minute
,
* sec
and millis
.
*/
public static Matcher hasHourMinSecAndMillis(final Integer hour, final ClockPeriod clockPeriod,
final Integer minute, final Integer second, final Integer millisecond) {
return IsDateWithTime.hasHourMinSecAndMillis(hour, clockPeriod, minute, second, millisecond);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* is in the given weekOfYear of the year.
*/
public static Matcher inWeekOfYear(final int week) {
return IsDateInWeekOfYear.inWeekOfYear(week);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents a leap year.
*/
public static Matcher leapYear() {
return IsDateInLeapYear.leapYear();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id January.
*/
public static Matcher january() {
return IsDateInMonth.january();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id February.
*/
public static Matcher february() {
return IsDateInMonth.february();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id March.
*/
public static Matcher march() {
return IsDateInMonth.march();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id April.
*/
public static Matcher april() {
return IsDateInMonth.april();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id May.
*/
public static Matcher may() {
return IsDateInMonth.may();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id June.
*/
public static Matcher june() {
return IsDateInMonth.june();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id July.
*/
public static Matcher july() {
return IsDateInMonth.july();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id August.
*/
public static Matcher august() {
return IsDateInMonth.august();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id September.
*/
public static Matcher september() {
return IsDateInMonth.september();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id October.
*/
public static Matcher october() {
return IsDateInMonth.october();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id November.
*/
public static Matcher november() {
return IsDateInMonth.november();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id December.
*/
public static Matcher december() {
return IsDateInMonth.december();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents a Sunday.
*/
public static Matcher sunday() {
return IsDateInDay.sunday();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents a Monday.
*/
public static Matcher monday() {
return IsDateInDay.monday();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents a Tuesday.
*/
public static Matcher tuesday() {
return IsDateInDay.tuesday();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents a Wednesday.
*/
public static Matcher wednesday() {
return IsDateInDay.wednesday();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents a Thursday.
*/
public static Matcher thursday() {
return IsDateInDay.thursday();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents a Friday.
*/
public static Matcher friday() {
return IsDateInDay.friday();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents a Saturday.
*/
public static Matcher saturday() {
return IsDateInDay.saturday();
}
//ITERABLE
/**
* Creates a matcher for {@link Iterable}s that matches when the
* examined {@link Iterable} has no items.
*
* For example:
*
assertThat(new ArrayList<>(), empty())
* returns true
.
*/
public static Matcher> empty() {
return IsEmptyIterable.empty();
}
/**
* Creates a matcher for {@link Iterable}s that matches when the
* examined {@link Iterable} has only distinct elements.
*
* For example:
*
assertThat(new ArrayList<>(), empty())
* returns true
.
*/
public static Matcher> hasDistinctElements() {
return IsIterableWithDistinctElements.hasDistinctElements();
}
/**
* Creates a matcher for {@link Iterable}s that matches when the
* examined {@link Iterable} yields an item count equal to 1
.
*
* For example:
*
assertThat(Arrays.asList("foo", "bar"), hasSizeOne())
* returns false
.
*/
public static Matcher> hasSizeOne() {
return IsIterableWithSize.hasSizeOne();
}
/**
* Creates a matcher for {@link Iterable}s that matches when the
* examined {@link Iterable} yields an item count equal to 2
.
*
* For example:
*
assertThat(Arrays.asList("foo", "bar"), hasSizeTwo())
* returns true
.
*/
public static Matcher> hasSizeTwo() {
return IsIterableWithSize.hasSizeTwo();
}
/**
* Creates a matcher for {@link Iterable}s that matches when the
* examined {@link Iterable} yields an item count equal to 3
.
*
* For example:
*
assertThat(Arrays.asList("foo", "bar"), hasSizeThree())
* returns false
.
*/
public static Matcher> hasSizeThree() {
return IsIterableWithSize.hasSizeThree();
}
/**
* Creates a matcher for {@link Iterable}s that matches when the
* examined {@link Iterable} yields an item count equal to 4
.
*
* For example:
*
assertThat(Arrays.asList("foo", "bar"), hasSizeFour())
* returns false
.
*/
public static Matcher> hasSizeFour() {
return IsIterableWithSize.hasSizeFour();
}
/**
* Creates a matcher for {@link Iterable}s that matches when the
* examined {@link Iterable} yields an item count equal to 5
.
*
* For example:
*
assertThat(Arrays.asList("foo", "bar"), hasSizeFive())
* returns false
.
*/
public static Matcher> hasSizeFive() {
return IsIterableWithSize.hasSizeFive();
}
/**
* Creates a matcher for {@link Iterable}s that matches when the
* examined {@link Iterable} yields an item count equal to size
.
*
* For example:
*
assertThat(Arrays.asList("foo", "bar"), hasSize(2))
* returns true
.
*/
public static Matcher> hasSize(final int size) {
return IsIterableWithSize.hasSize(size);
}
/**
* Creates a matcher for {@linkplain Multiset} matching when the examined object E
* has size
occurrences.
*/
public static Matcher> elementWithCount(final E element, final int size) {
return IsMultisetElementWithCount.elementWithCount(element, size);
}
/**
* Creates a matcher for {@link Iterable}s matching when the examined {@linkplain Iterable} has
* the elements of type K
that extends {@linkplain Comparable} and the elements
* are sorted according to the natural ordering.
*/
public static Matcher> sorted() {
return IsSortedIterable.sorted();
}
/**
* Creates a matcher for {@link Iterable}s matching when the examined {@linkplain Iterable} has
* the elements of type K
that extends {@linkplain Comparable} and the elements
* are sorted according to the inverse of natural ordering.
*/
public static Matcher> sortedReversed() {
return IsSortedIterable.sortedReversed();
}
/**
* Creates a matcher for {@link Iterable}s matching when the examined {@linkplain Iterable} has
* the elements sorted according to the given {@linkplain Comparator}.
*/
public static Matcher> sorted(final Comparator comparator) {
return IsSortedIterableWithComparator.sorted(comparator);
}
/**
* Creates a matcher for {@link Iterable}s matching when the examined {@linkplain Iterable} has
* the elements sorted according to the given {@linkplain Comparator}, but in a reverse order.
*/
public static Matcher> sortedReversed(final Comparator comparator) {
return IsSortedIterableWithComparator.sortedReversed(comparator);
}
//MAP
/**
* Creates a matcher for {@link java.util.Map}s matching when the examined {@link java.util.Map} has exactly
* the same key set of the given map.
* For example:
* assertThat(myMap, hasSameKeySet(anotherMap))
*/
public static Matcher