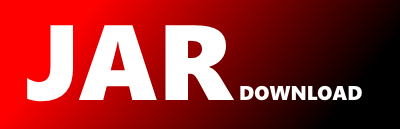
it.ozimov.cirneco.hamcrest.date.IsDateInMonth Maven / Gradle / Ivy
package it.ozimov.cirneco.hamcrest.date;
import com.google.common.base.Optional;
import it.ozimov.cirneco.hamcrest.date.utils.CalendarUtils;
import org.hamcrest.Description;
import org.hamcrest.Matcher;
import org.hamcrest.TypeSafeMatcher;
import java.util.Calendar;
import java.util.Date;
import static com.google.common.base.Preconditions.checkArgument;
/**
* Is {@linkplain Date} in a given id?
*
* @since version 0.1 for JDK7
*/
public class IsDateInMonth extends TypeSafeMatcher {
private final Month month;
/**
*
*/
public IsDateInMonth(final int month) {
//Months in {@linkplain Calendar} are zero-based
final Optional monthOptional = Month.fromId(month);
checkArgument(monthOptional.isPresent(),
String.format("The id %d is not a valid value (admitted values are [1,2,...,12])",
month));
this.month = monthOptional.get();
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id January.
*/
public static Matcher january() {
return new IsDateInMonth(1);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id February.
*/
public static Matcher february() {
return new IsDateInMonth(2);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id March.
*/
public static Matcher march() {
return new IsDateInMonth(3);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id April.
*/
public static Matcher april() {
return new IsDateInMonth(4);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id May.
*/
public static Matcher may() {
return new IsDateInMonth(5);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id June.
*/
public static Matcher june() {
return new IsDateInMonth(6);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id July.
*/
public static Matcher july() {
return new IsDateInMonth(7);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id August.
*/
public static Matcher august() {
return new IsDateInMonth(8);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id September.
*/
public static Matcher september() {
return new IsDateInMonth(9);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id October.
*/
public static Matcher october() {
return new IsDateInMonth(10);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id November.
*/
public static Matcher november() {
return new IsDateInMonth(11);
}
/**
* Creates a matcher that matches when the examined {@linkplain Date}
* represents the id December.
*/
public static Matcher december() {
return new IsDateInMonth(12);
}
@Override
protected boolean matchesSafely(final Date date) {
final int monthFromDate = CalendarUtils.month(date);
switch (month) {
case JANUARY:
return monthFromDate == Month.JANUARY.id;
case FEBRUARY:
return monthFromDate == Month.FEBRUARY.id;
case MARCH:
return monthFromDate == Month.MARCH.id;
case APRIL:
return monthFromDate == Month.APRIL.id;
case MAY:
return monthFromDate == Month.MAY.id;
case JUNE:
return monthFromDate == Month.JUNE.id;
case JULY:
return monthFromDate == Month.JULY.id;
case AUGUST:
return monthFromDate == Month.AUGUST.id;
case SEPTEMBER:
return monthFromDate == Month.SEPTEMBER.id;
case OCTOBER:
return monthFromDate == Month.OCTOBER.id;
case NOVEMBER:
return monthFromDate == Month.NOVEMBER.id;
case DECEMBER:
return monthFromDate == Month.DECEMBER.id;
default:
return false;
}
}
@Override
protected void describeMismatchSafely(final Date date, final Description mismatchDescription) {
mismatchDescription.appendValue(date)
.appendText(" has not id ")
.appendValue(month);
}
@Override
public void describeTo(final Description description) {
description
.appendText("a date with id ")
.appendValue(month);
}
/**
* Months in {@linkplain Calendar} are zero-based
*/
private enum Month {
JANUARY(Calendar.JANUARY),
FEBRUARY(Calendar.FEBRUARY),
MARCH(Calendar.MARCH),
APRIL(Calendar.APRIL),
MAY(Calendar.MAY),
JUNE(Calendar.JUNE),
JULY(Calendar.JULY),
AUGUST(Calendar.AUGUST),
SEPTEMBER(Calendar.SEPTEMBER),
OCTOBER(Calendar.OCTOBER),
NOVEMBER(Calendar.NOVEMBER),
DECEMBER(Calendar.DECEMBER);
final int id;
Month(final int id) {
this.id = id + 1;
}
static Optional fromId(final int weekDay) {
for (Month month : values()) {
if (month.id == weekDay) {
return Optional.of(month);
}
}
return Optional.absent();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy