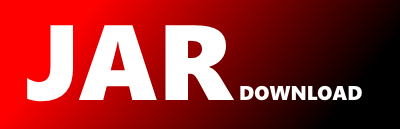
it.skrape.selects.CssSelector.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of skrapeit-html-parser Show documentation
Show all versions of skrapeit-html-parser Show documentation
A Kotlin-based testing/scraping/parsing library providing the ability to analyze and extract data from HTML (server & client-side rendered). It places particular emphasis on ease of use and a high level of readability by providing an intuitive DSL. First and foremost it aims to be a testing lib, but it can also be used to scrape websites in a convenient fashion.
package it.skrape.selects
import it.skrape.SkrapeItDsl
import org.jsoup.nodes.Document
@Suppress("LongParameterList")
@SkrapeItDsl
public class CssSelector(
public var rawCssSelector: String = "",
public var withClass: CssClassName? = null,
public var withId: String? = null,
public var withAttributeKey: String? = null,
public var withAttributeKeys: List? = null,
public var withAttribute: Pair? = null,
public var withAttributes: List>? = null,
public val doc: CssSelectable = Doc(Document(""))
) : CssSelectable() {
override val toCssSelector: String
get() = ("${doc.toCssSelector} $this").trim()
override fun applySelector(rawCssSelector: String): List =
doc.applySelector("$this $rawCssSelector".trim())
override fun toString(): String = rawCssSelector.trim() + buildString {
append(withId.toIdSelector())
append(withClass.toClassesSelector())
append(withAttributeKey.toAttributeKeySelector())
append(withAttributeKeys.toAttributesKeysSelector())
append(withAttribute.toAttributeSelector())
append(withAttributes.toAttributesSelector())
}.withoutSpaces()
private fun String?.toIdSelector() = this?.let { "#$it" }.orEmpty()
private fun CssClassName?.toClassesSelector() = this?.let { ".$it" }.orEmpty()
private fun String?.toAttributeKeySelector() = this?.let { "[$it]" }.orEmpty()
private fun List?.toAttributesKeysSelector() =
this?.joinToString(prefix = "['", separator = "']['", postfix = "']").orEmpty()
private fun Pair?.toAttributeSelector() =
this?.let { "[${it.first}='${it.second}']" }.orEmpty()
private fun List>?.toAttributesSelector() =
this?.joinToString(separator = "") { "[${it.first}='${it.second}']" }.orEmpty()
private fun String.withoutSpaces() = replace("\\s".toRegex(), "")
}
public typealias CssClassName = String
public infix fun CssClassName.and(value: String): String = "$this.$value"
public infix fun Pair.and(pair: Pair): MutableList> =
mutableListOf(this).apply { add(pair) }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy