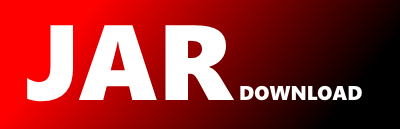
it.tidalwave.ui.android.widget.AsAdapter Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - Android - open source birding
* Copyright (C) 2009-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluebill.tidalwave.it/mobile
* SCM: https://java.net/hg/bluebill-mobile~android-src
*
**********************************************************************************************************************/
package it.tidalwave.ui.android.widget;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import javax.annotation.Nonnegative;
import java.util.List;
import it.tidalwave.util.As;
import it.tidalwave.util.thread.annotation.ThreadConfined;
import android.content.Context;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.Filterable;
import android.widget.TextView;
import it.tidalwave.ui.android.view.AdapterViewHelper;
import it.tidalwave.ui.android.view.AdapterViewHelper.Updater;
import static it.tidalwave.util.thread.ThreadType.*;
import static it.tidalwave.role.ui.android.TextViewRenderable.TextViewRenderable;
/***********************************************************************************************************************
*
* An implementation of {@link BaseAdapter} for rendering lists containing instances of {@link As}.
*
* FIXME: must be merged to PresentationModelAdapter.
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public abstract class AsAdapter extends BaseAdapter implements Filterable
{
private final int layoutId;
private final int componentViewId;
private final AdapterViewHelper adapterViewHelper;
@Nonnull
private final List extends As> objects;
@CheckForNull
private AsWidgetFilter filter;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@ThreadConfined(type=UI)
public AsAdapter (final int layoutId,
final int componentViewId,
final @Nonnull Context context,
final @Nonnull List extends As> objects)
{
this.layoutId = layoutId;
this.componentViewId = componentViewId;
this.adapterViewHelper = new AdapterViewHelper(context);
this.objects = objects;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnegative @ThreadConfined(type=UI)
public int getCount()
{
return getFilter().getCount();
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull @ThreadConfined(type=UI)
public As getItem (final @Nonnegative int index)
{
return getFilter().getItem(index);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @ThreadConfined(type=UI)
public long getItemId (final @Nonnegative int id)
{
return id;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
* Lazy creation of the Filter is important because this object can be created in a background thread: the Filter
* doesn't work when it is created in background.
*
******************************************************************************************************************/
@Override @Nonnull @ThreadConfined(type=UI)
public synchronized AsWidgetFilter getFilter()
{
if (filter == null)
{
this.filter = createFilter(objects);
}
return filter;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull @ThreadConfined(type=UI)
public View getView (final @Nonnegative int index,
final @CheckForNull View view,
final @Nonnull ViewGroup viewGroup)
{
return adapterViewHelper.prepareView(layoutId, componentViewId, view, viewGroup, new Updater()
{
public void update (final @Nonnull TextView textView)
{
getItem(index).as(TextViewRenderable).renderTo(textView);
}
});
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull @ThreadConfined(type=UI)
protected abstract AsWidgetFilter createFilter (@Nonnull List extends As> objects);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy