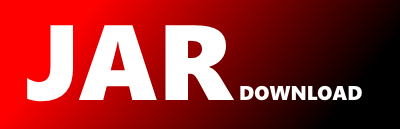
it.tidalwave.ui.android.widget.PresentationModelAdapter Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - Android - open source birding
* Copyright (C) 2009-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluebill.tidalwave.it/mobile
* SCM: https://java.net/hg/bluebill-mobile~android-src
*
**********************************************************************************************************************/
package it.tidalwave.ui.android.widget;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.List;
import javax.swing.Action;
import java.awt.event.ActionEvent;
import it.tidalwave.util.AsException;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.role.SimpleComposite;
import it.tidalwave.role.ui.ActionProvider;
import it.tidalwave.role.ui.PresentationModel;
import android.content.Context;
import android.view.ViewGroup;
import android.view.ContextMenu;
import android.view.ContextMenu.ContextMenuInfo;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.AdapterView.AdapterContextMenuInfo;
import android.widget.BaseAdapter;
import android.widget.TextView;
import lombok.Getter;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import static it.tidalwave.role.ui.ActionProvider.ActionProvider;
import static it.tidalwave.role.ui.android.ViewRenderable.ViewRenderable;
import static it.tidalwave.role.ui.android.ViewFactory.ViewFactory;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public class PresentationModelAdapter extends BaseAdapter
{
private final Logger log = LoggerFactory.getLogger(PresentationModelAdapter.class);
@Nonnull
private final Context context;
@Nonnull
private final List presentationModels;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public PresentationModelAdapter (final @Nonnull Context context,
final @Nonnull PresentationModel presentationModel)
{
log.debug("PresentationModelAdapter()");
this.context = context;
this.presentationModels = new ArrayList();
try
{
// FIXME: why SimpleComposite?
final SimpleComposite composite = presentationModel.as(SimpleComposite.class);
presentationModels.addAll(composite.findChildren().results());
}
catch (AsException e)
{
log.debug(">>>> presentationModel is not a Composite");
// ok, no Composite
}
// log.debug(">>>> created from {}, {} items", presentationModel, presentationModels.size());
log.debug("PresentationModelAdapter - created from {}@{}, {} items",
new Object[] { presentationModel.getClass().getSimpleName(),
Integer.toHexString(System.identityHashCode(presentationModel)),
presentationModels.size() });
}
/*******************************************************************************************************************
*
* An {@link AdapterView.OnItemClickListener} that must be set to each {@code ListView} bound to this adapter, in
* order to delegate the click gesture management to a preferred action provided by the {@link ActionProvider} role
* bound to a {@link PresentationModel}.
*
******************************************************************************************************************/
@Getter
private final AdapterView.OnItemClickListener onItemClickListener = new AdapterView.OnItemClickListener()
{
public void onItemClick (final @Nonnull AdapterView> adapterView,
final @Nonnull View view,
final int index,
final long id)
{
try
{
final ActionEvent actionEvent = new ActionEvent(view, ActionEvent.ACTION_PERFORMED, "actionPerformed");
getItem(index).as(ActionProvider).getDefaultAction().actionPerformed(actionEvent);
}
catch (AsException e)
{
// ok, no ActionProvider
}
catch (NotFoundException e)
{
// ok, no default Action
}
}
};
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnegative
public int getCount()
{
return presentationModels.size();
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnull
public PresentationModel getItem (final @Nonnegative int index)
{
return presentationModels.get(index);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
public long getItemId (final int id)
{
return id;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnull
public View getView (final @Nonnegative int index,
final @Nonnull View convertView,
final @Nonnull ViewGroup viewGroup)
{
// FIXME: recycle view when possible
// NameViewHelper nameViewHelper;
//
// if (view == null)
// {
// view = layoutInflater.inflate(resourceId, null);
// view.setTag(nameViewHelper = new NameViewHelper(view));
// }
// else
// {
// nameViewHelper = (NameViewHelper)view.getTag();
// }
//
// nameViewHelper.set(getItem(index));
//
// return view;
try
{
final PresentationModel presentationModel = getItem(index);
final View view = presentationModel.as(ViewFactory).createView(context, null);
presentationModel.as(ViewRenderable).renderTo(view);
return view;
}
catch (AsException e)
{
log.error("Can't find needed role: ", e);
final TextView textView = new TextView(context); // FIXME: recycle
textView.setText("Missing role: " + e.getMessage());
return textView;
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public void onCreateContextMenu (final @Nonnull ContextMenu menu,
final @Nonnull View view,
final @Nonnull ContextMenuInfo menuInfo)
{
final AdapterContextMenuInfo info = (AdapterContextMenuInfo)menuInfo;
try
{
final ActionProvider actionProvider = getItem(info.position).as(ActionProvider);
int id = 0;
for (final Action action : actionProvider.getActions())
{
final MenuItem menuItem = menu.add(0, id++, 0, (String)action.getValue(Action.NAME));
menuItem.setEnabled(action.isEnabled());
}
}
catch (AsException e)
{
// ok, no ActionProvider
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public boolean onContextItemSelected (final @Nonnull MenuItem item)
{
final AdapterContextMenuInfo info = (AdapterContextMenuInfo)item.getMenuInfo();
try
{
final ActionProvider actionProvider = getItem(info.position).as(ActionProvider);
final Action[] actions = actionProvider.getActions().toArray(new Action[0]);
final int menuIndex = item.getItemId();
actions[menuIndex].actionPerformed(new ActionEvent(this, ActionEvent.ACTION_PERFORMED, "actionPerformed"));
}
catch (AsException e)
{
return false; // no ActionProvider
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy