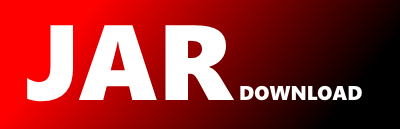
it.tidalwave.bluebill.factsheet.bbc.BbcFactSheetProvider Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - Android - open source birding
* Copyright (C) 2009-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluebill.tidalwave.it/mobile
* SCM: https://kenai.com/hg/bluebill~android-src
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.factsheet.bbc;
import javax.annotation.Nonnull;
import java.util.Locale;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.io.InputStream;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.openrdf.model.Graph;
import org.openrdf.rio.RDFHandlerException;
import org.openrdf.rio.RDFParseException;
import it.tidalwave.util.Id;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.mobile.media.Media;
import it.tidalwave.mobile.util.Downloadable;
import it.tidalwave.semantic.GenericEntity;
import it.tidalwave.semantic.io.GraphDeserializer;
import it.tidalwave.semantic.io.GraphUnmarshaller;
import it.tidalwave.semantic.io.MimeTypes;
import it.tidalwave.semantic.io.impl.DefaultGraphDeserializer;
import it.tidalwave.semantic.io.impl.GraphUnmarshallerImpl;
import it.tidalwave.bluebill.factsheet.FactSheet;
import it.tidalwave.bluebill.factsheet.bbc.vocabulary.BbcVocabulary;
import it.tidalwave.bluebill.factsheet.bbc.io.WoUnmarshallerFactory;
import it.tidalwave.bluebill.taxonomy.mobile.Taxon;
import static it.tidalwave.semantic.GenericEntity.*;
import static it.tidalwave.mobile.util.Downloadable.Downloadable;
import static it.tidalwave.bluebill.factsheet.bbc.vocabulary.BbcVocabulary.*;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public class BbcFactSheetProvider
{
private static final Logger log = LoggerFactory.getLogger(BbcFactSheetProvider.class);
public static final GenericEntity BBC = namedEntity(new Id(BBC_URI), BBC_DISPLAY_NAME);
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public FactSheet createFactSheet (final @Nonnull Taxon taxon)
throws IOException, NotFoundException
{
log.info("createFactSheet({})", taxon);
try
{
final URI uri = getUriFor(taxon);
log.info(">>>> resource uri: {}", uri);
final Media media = new Media().with(Media.ID, new Id(uri.toASCIIString()));
// final Media media = new Media().with(Media.RES_URL, uri);
final Downloadable downloadable = media.as(Downloadable);
downloadable.download();
downloadable.waitUntilDownloadingCompleted();
return loadGraph(downloadable);
}
catch (InterruptedException e)
{
throw new IOException(e.toString()); // Java 5 / Android compatibility
}
catch (URISyntaxException e)
{
throw new IOException(e.toString()); // Java 5 / Android compatibility
}
catch (RDFHandlerException e)
{
throw new IOException(e.toString()); // Java 5 / Android compatibility
}
catch (RDFParseException e)
{
throw new IOException(e.toString()); // Java 5 / Android compatibility
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
protected FactSheet loadGraph (final @Nonnull Downloadable downloadable)
throws IOException, RDFParseException, RDFHandlerException
{
final File file = downloadable.getFile();
log.info(">>>> resource cached to {}", file);
final InputStream is = new BufferedInputStream(new FileInputStream(file), 16 * 1024);
final Graph graph = loadGraph(is);
final FactSheet factSheet = loadFactSheet(graph);
is.close(); // FIXME: finally
return factSheet;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
protected URI getUriFor (final @Nonnull Taxon taxon)
throws NotFoundException, URISyntaxException
{
final String displayName = taxon.getDisplayName(Locale.UK);
final StringBuilder buffer = new StringBuilder(BBC_CONTEXT + "nature/species/");
String separator = "";
for (final String s : displayName.split(" "))
{
buffer.append(separator).append(capitalized(s));
separator = "_";
}
buffer.append(".rdf");
return new URI(buffer.toString());
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
protected Graph loadGraph (final @Nonnull InputStream is)
throws IOException, RDFHandlerException, RDFParseException
{
final GraphDeserializer deserializer = new DefaultGraphDeserializer();
deserializer.setContextUri(BBC_CONTEXT);
return deserializer.read(is, MimeTypes.MIME_RDF_XML);
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
protected FactSheet loadFactSheet (final @Nonnull Graph graph)
{
final GraphUnmarshaller unmarshaller = new GraphUnmarshallerImpl();
unmarshaller.registerStatementUnmarshallerFactory(new WoUnmarshallerFactory());
return (FactSheet)unmarshaller.unmarshal(graph, new Id(BbcVocabulary.TYPE_WO_SPECIES.stringValue()));
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
private static String capitalized (final @Nonnull String string)
{
return string.substring(0, 1).toUpperCase(Locale.UK) + string.substring(1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy