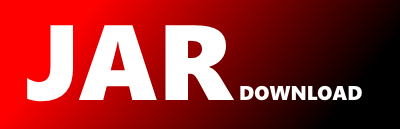
it.tidalwave.bluebill.mobile.taxonomy.factsheet.ui.main.NewTaxonMainFactSheetViewController.suppressed Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueBill Mobile - Android - open source birding
* Copyright (C) 2009-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluebill.tidalwave.it/mobile
* SCM: https://java.net/hg/bluebill-mobile~android-src
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.mobile.taxonomy.factsheet.ui.main;
import javax.annotation.Nonnull;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.SortedSet;
import java.util.TreeSet;
import it.tidalwave.util.thread.annotation.ThreadConfined;
import it.tidalwave.util.Id;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.util.logging.Logger;
import it.tidalwave.mobile.media.Media;
import it.tidalwave.bluebill.taxonomy.mobile.Taxon;
import it.tidalwave.bluebill.factsheet.Adaptation;
import it.tidalwave.bluebill.factsheet.Biblio;
import it.tidalwave.bluebill.factsheet.Habitat;
import it.tidalwave.bluebill.factsheet.FactSheet;
import it.tidalwave.bluebill.mobile.taxonomy.factsheet.spi.TaxonFactSheetViewControllerSupport;
import it.tidalwave.bluebill.mobile.taxonomy.factsheet.ui.TaxonFactSheetView;
import static it.tidalwave.util.thread.ThreadType.*;
import static it.tidalwave.role.Displayable.Displayable;
import static it.tidalwave.semantic.vocabulary.DublinCoreVocabulary.*;
import static it.tidalwave.bluebill.factsheet.bbc.vocabulary.BbcVocabulary.*;
/***********************************************************************************************************************
*
* FIXME: this is actually unused. It was originally developed to render the structured information from BBC.
* Currently is replaced by the facts/photos/videos/sounds fact sheets.
*
* TODO: reuse this later when you'll be able to better structure the Wikipedia info.
*
* @stereotype Controller
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public abstract class NewTaxonMainFactSheetViewController extends TaxonFactSheetViewControllerSupport
{
private static final String CLASS = NewTaxonMainFactSheetViewController.class.getName();
private static final Logger logger = Logger.getLogger(CLASS);
// We don't render these obvious bird adaptations
private static final List OBVIOUS_BIRD_ADAPTATIONS = Arrays.asList
(
new Id("http://www.bbc.co.uk/nature/adaptations/Oviparity#adaptation")
);
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public NewTaxonMainFactSheetViewController (final @Nonnull TaxonFactSheetView view)
{
super(view);
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@ThreadConfined(type=NOT_UI)
protected void createPresentationModels (final @Nonnull Taxon taxon)
throws NotFoundException
{
final FactSheet factSheet = null; // getFactSheet();
final Taxon genus = taxon.getParent();
final Taxon family = genus.getParent();
final Taxon order = family.getParent();
clearPresentationModels();
addPresentationModel(new TextPresentationModel("Order: " + order.getScientificName()));
addPresentationModel(new TextPresentationModel("Family: " + family.getScientificName()));
// try
// {
Collection stillImages = null;
try
{
stillImages = factSheet.getMultiple(IMAGE);
}
catch (ClassCastException e) // FIXME
{
stillImages = Collections.singletonList((Media)(Object)factSheet.get(IMAGE));
}
if (!stillImages.isEmpty())
{
final Media stillImage = stillImages.iterator().next();
// add(new PresentationModel(new URI(stillImage.getId().stringValue())));
}
// }
// catch (URISyntaxException e)
// {
// logger.throwing("", CLASS, e);
// }
addPresentationModel(new TextPresentationModel(factSheet.get(DC_DESCRIPTION)));
renderHabitats(factSheet);
renderAdaptations(factSheet);
renderBiblios(factSheet);
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private void renderHabitats (final FactSheet factSheet)
throws NotFoundException
{
final Collection habitats = factSheet.getMultiple(HABITAT);
if (!habitats.isEmpty())
{
addPresentationModel(new HeaderPresentationModel("Habitats"));
final SortedSet habitatNames = new TreeSet();
for (final Habitat habitat : habitats)
{
habitatNames.add(habitat.as(Displayable).getDisplayName());
}
for (final String habitatName : habitatNames)
{
addPresentationModel(new TextPresentationModel(habitatName));
}
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private void renderAdaptations (final FactSheet factSheet)
throws NotFoundException
{
final Collection adaptations = factSheet.getMultiple(ADAPTATION);
if (!adaptations.isEmpty())
{
addPresentationModel(new HeaderPresentationModel("Adaptations"));
final SortedSet adaptationNames = new TreeSet();
for (final Adaptation adaptation : adaptations)
{
if (!OBVIOUS_BIRD_ADAPTATIONS.contains(adaptation.getId()))
{
adaptationNames.add(adaptation.as(Displayable).getDisplayName());
}
}
for (final String adaptationName : adaptationNames)
{
addPresentationModel(new TextPresentationModel(adaptationName));
}
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private void renderBiblios (final @Nonnull FactSheet factSheet)
throws NotFoundException
{
final Collection biblios = factSheet.getMultiple(BIBLIO);
if (!biblios.isEmpty())
{
addPresentationModel(new HeaderPresentationModel("References"));
int i = 1;
for (final Biblio biblio : biblios)
{
final StringBuilder buffer = new StringBuilder();
buffer.append(String.format("[%d] ", i++)).append(biblio.getId().stringValue()).append("
");
if (biblio.containsKey(DC_TITLE))
{
buffer.append("").append(biblio.get(DC_TITLE)).append("
");
}
if (biblio.containsKey(DC_DESCRIPTION))
{
buffer.append(biblio.get(DC_DESCRIPTION)).append("
");
}
addPresentationModel(new TextPresentationModel(buffer.toString()));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy