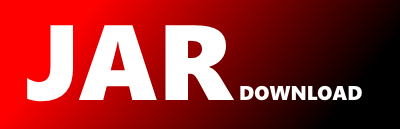
it.tidalwave.bluebill.mobile.taxonomy.TaxonObservable Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - open source birdwatching
* ==========================================
*
* Copyright (C) 2009, 2010 by Tidalwave s.a.s. (http://www.tidalwave.it)
* http://bluebill.tidalwave.it/mobile/
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* $Id: TaxonObservable.java,v 64899513037c 2010/05/29 11:09:38 fabrizio $
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.mobile.taxonomy;
import javax.annotation.Nonnull;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.List;
import java.io.Externalizable;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.io.Serializable;
import org.openide.util.Lookup;
import it.tidalwave.util.Id;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.bluebill.observation.Finder;
import it.tidalwave.bluebill.observation.Observable;
import it.tidalwave.bluebill.observation.Observation;
import it.tidalwave.bluebill.observation.ObservationItem;
import it.tidalwave.bluebill.observation.simple.AsSupport;
import it.tidalwave.bluebill.taxonomy.Taxon;
import static it.tidalwave.bluebill.taxonomy.Taxon.Taxon;
import it.tidalwave.bluebill.taxonomy.Taxonomy;
/***********************************************************************************************************************
*
* Makes sure that only the id of a Taxon is serialized, and not the whole class with the whole Taxonomy.
*
* @author Fabrizio Giudici
* @version $Id: $
*
**********************************************************************************************************************/
public class TaxonObservable extends AsSupport implements Observable, Externalizable
{
public static final Class TaxonObservable = TaxonObservable.class;
private static final long serialVersionUID = 4534653437593487L;
// FIXME: drop this when you have a real Lookup that you can mock
public static Taxonomy testTaxonomy;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public TaxonObservable() // for deserialization
{
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public TaxonObservable (final @Nonnull Taxon taxon,
final @Nonnull Object ... capabilities)
{
super(taxon);
this.capabilities.addAll(Arrays.asList(capabilities));
this.lookup = null; // force recreation - this won't fire events, though
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Finder findObservationItems()
{
throw new UnsupportedOperationException("Not supported yet.");
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Finder findObservations()
{
throw new UnsupportedOperationException("Not supported yet.");
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public void writeExternal (final @Nonnull ObjectOutput oo)
throws IOException
{
oo.writeObject(as(Taxon).getId());
oo.writeObject(getSerializables(capabilities));
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public void readExternal (final @Nonnull ObjectInput oi)
throws IOException, ClassNotFoundException
{
final Id id = (Id)oi.readObject();
try
{
Taxonomy taxonomy = null;
if (testTaxonomy != null)
{
taxonomy = testTaxonomy;
}
else
{
final Lookup lookup = Lookup.getDefault();
taxonomy = lookup.lookup(TaxonomyPreferences.class).getTaxonomy();
}
final Taxon taxon = taxonomy.findTaxonById(id);
capabilities.add(taxon);
capabilities.addAll((List)oi.readObject());
}
catch (NotFoundException e)
{
throw new IOException(e.toString());
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Override
public boolean equals (Object o)
{
if ((o == null) || !(o instanceof TaxonObservable))
{
return false;
}
return as(Taxon).equals(((TaxonObservable)o).as(Taxon));
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Override
public int hashCode()
{
return as(Taxon).hashCode();
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private static List getSerializables (final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy