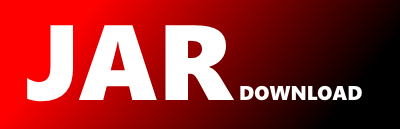
it.tidalwave.bluebill.mobile.observation.DefaultObservationUIController Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - open source birdwatching
* ==========================================
*
* Copyright (C) 2009, 2010 by Tidalwave s.a.s. (http://www.tidalwave.it)
* http://bluebill.tidalwave.it/mobile/
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* $Id: DefaultObservationUIController.java,v 9c4fd77fbb26 2010/07/06 12:23:00 fabrizio $
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.mobile.observation;
import it.tidalwave.mobile.util.ProgressListener;
import it.tidalwave.bluebill.mobile.observation.share.TextReportGenerator;
import it.tidalwave.bluebill.mobile.observation.share.KMLReportGenerator;
import javax.annotation.Nonnull;
import java.util.Calendar;
import org.openide.util.NbBundle;
import it.tidalwave.util.logging.Logger;
import it.tidalwave.netbeans.util.Locator;
import it.tidalwave.mobile.location.LocationFinder;
import it.tidalwave.bluebill.observation.Observation;
import it.tidalwave.bluebill.observation.Observation.Builder;
import it.tidalwave.bluebill.observation.ObservationItem;
import it.tidalwave.bluebill.observation.ObservationManager;
import it.tidalwave.bluebill.observation.ObservationSet;
import it.tidalwave.bluebill.observation.ObservationSetVisitor;
import it.tidalwave.bluebill.mobile.observation.share.Report;
import it.tidalwave.bluebill.mobile.share.SharingPreferences;
import it.tidalwave.bluebill.mobile.taxonomy.TaxonomyPreferences;
import static it.tidalwave.bluebill.taxonomy.Taxon.Taxon;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id: $
*
**********************************************************************************************************************/
public abstract class DefaultObservationUIController implements ObservationUIController
{
private static final String CLASS = DefaultObservationUIController.class.getName();
private static final Logger logger = Logger.getLogger(CLASS);
@Nonnull
private final TaxonomyPreferences taxonomyPreferences = Locator.find(TaxonomyPreferences.class);
@Nonnull
private final SharingPreferences sharingPreferences = Locator.find(SharingPreferences.class);
@Nonnull
private final ObservationClipboard observationClipboard = Locator.find(ObservationClipboard.class);
@Nonnull
private final ObservationManager observationManager = Locator.find(ObservationManager.class);
@Nonnull
private final ObservationSet observationSet = observationManager.findOrCreateObservationSetById(null);
@Nonnull
private final ObservationUI ui;
/*******************************************************************************************************************
*
*
*
******************************************************************************************************************/
public DefaultObservationUIController (final @Nonnull ObservationUI ui)
{
this.ui = ui;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
public void startNewObservationSequence()
{
logger.info("startNewObservationSequence()");
observationClipboard.clear();
final Builder builder = observationClipboard.getBuilder();
observationClipboard.setBuilder(builder.at(Calendar.getInstance().getTime()));
Locator.find(LocationFinder.class).start(); // search needs time, and we pre-start it
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
public void browseToFactSheet()
{
logger.info("browseToFactSheet()");
// nothing to do by default
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
public void commitNewObservation()
{
logger.info("commitNewObservation()");
final Observation.Builder builder = observationClipboard.getBuilder();
if (builder.getItemCount() > 0)
{
builder.build();
final String message = NbBundle.getMessage(DefaultObservationUIController.class, "addedNewObservation");
ui.getCommonUITasks().showTemporaryMessage(message);
ui.highlightLatestObservation();
}
observationClipboard.clear();
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
public void cancelNewObservation()
{
logger.info("cancelNewObservation()");
Locator.find(LocationFinder.class).stop(); // search needs time, and we pre-start it
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
public void deleteAllObservations()
{
logger.info("deleteAllObservations()");
final String title = NbBundle.getMessage(DefaultObservationUIController.class, "confirm");
final String message = NbBundle.getMessage(DefaultObservationUIController.class, "confirmEraseAllObservations");
ui.getCommonUITasks().alertDialog(title, message, new Runnable()
{
public void run()
{
observationSet.clear();
}
});
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
public void deleteObservationItem (final @Nonnull ObservationItem observationItem)
{
logger.info("deleteObservationItem(%s)", observationItem);
final String title = NbBundle.getMessage(DefaultObservationUIController.class, "confirm");
final String message = NbBundle.getMessage(DefaultObservationUIController.class,
"confirmDeleteObservationItem",
taxonomyPreferences.format(observationItem.getObservable().as(Taxon)));
final String itemDeleted = NbBundle.getMessage(DefaultObservationUIController.class, "itemDeleted");
ui.getCommonUITasks().alertDialog(title, message, new Runnable()
{
public void run()
{
try
{
observationItem.getObservation().change().without(observationItem).apply();
ui.getCommonUITasks().showTemporaryMessage(itemDeleted);
}
catch (Exception e)
{
throw new RuntimeException(e);
}
}
});
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
public final void shareObservations (final @Nonnull ShareOptions options)
{
logger.info("shareObservations(%s)", options);
final String subject = NbBundle.getMessage(DefaultObservationUIController.class, "observations");
final String creatingTextReport = NbBundle.getMessage(DefaultObservationUIController.class, "creatingTextReport");
final String creatingKmlReport = NbBundle.getMessage(DefaultObservationUIController.class, "creatingKMLReport");
final ProgressListener progressListener = options.getProgressListener();
final ObservationSetVisitor visitor = observationSet.as(ObservationSetVisitor.class);
progressListener.start();
progressListener.setProgressName(creatingTextReport);
final TextReportGenerator textReportGenerator = new TextReportGenerator();
visitor.visit(new VisitorListenerDelegate(textReportGenerator, progressListener));
Report textReport = new Report().withSubject(subject).
withRecipients(sharingPreferences.getDefaultRecipients()).
withBody(textReportGenerator.getMessage());
if (options.isKmlAttachment())
{
logger.info(">>>> creating KML attachment");
progressListener.setProgressName(creatingKmlReport);
final KMLReportGenerator kmlReportGenerator = new KMLReportGenerator();
visitor.visit(new VisitorListenerDelegate(kmlReportGenerator, progressListener));
final Report kmlReport = new Report().withSubject("observations.kml")
.withMimeType("application/vnd.google-earth.kml+xml")
.withBody(kmlReportGenerator.getMessage());
textReport = textReport.withAttachment(kmlReport);
}
progressListener.stop();
shareObservations(textReport);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
protected abstract void shareObservations (@Nonnull Report report);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy