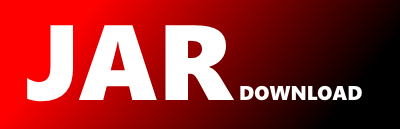
it.tidalwave.bluebill.mobile.observation.ObservationClipboard Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - open source birdwatching
* ==========================================
*
* Copyright (C) 2009, 2010 by Tidalwave s.a.s. (http://www.tidalwave.it)
* http://bluebill.tidalwave.it/mobile/
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* $Id: ObservationClipboard.java,v ee8fe6a3fbd7 2010/07/01 00:13:27 fabrizio $
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.mobile.observation;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.List;
import java.io.ObjectInputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.util.Id;
import it.tidalwave.util.logging.Logger;
import it.tidalwave.netbeans.util.Locator;
import it.tidalwave.mobile.io.FileSystem;
import it.tidalwave.mobile.io.MasterFileSystem;
import it.tidalwave.bluebill.observation.Observation;
import it.tidalwave.bluebill.observation.Observation.Builder;
import it.tidalwave.bluebill.observation.ObservationManager;
import it.tidalwave.bluebill.observation.ObservationSet;
import it.tidalwave.bluebill.taxonomy.Taxonomy;
import it.tidalwave.bluebill.taxonomy.Taxon;
import it.tidalwave.bluebill.mobile.taxonomy.TaxonomyPreferences;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id: $
*
**********************************************************************************************************************/
public class ObservationClipboard
{
private static final String CLASS = ObservationClipboard.class.getName();
private static final Logger logger = Logger.getLogger(CLASS);
/* package */ static final String NAME = "TaxonPickHistory.ser";
private static final int MAX_HISTORY_SIZE = 20;
private FileSystem fileSystem;
@Nonnegative
/* package */ int maxHistorySize = MAX_HISTORY_SIZE;
@CheckForNull
/* package */ Observation.Builder builder;
/* package */ List taxonIdHistory = new ArrayList();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public ObservationClipboard()
{
try
{
fileSystem = Locator.find(MasterFileSystem.class).getInternalFileSystem();
}
catch (Locator.NotFoundException e)
{
// ok
}
load();
if (taxonIdHistory.isEmpty())
{
taxonIdHistory.add(new Id("urn:tidalwave:taxo/concept#4d3a8321-42f3-11de-af74-002332c672e6")); // grey heron
taxonIdHistory.add(new Id("urn:tidalwave:taxo/concept#4ef1d011-42f3-11de-af74-002332c672e6")); // black-headed gull
taxonIdHistory.add(new Id("urn:tidalwave:taxo/concept#4cdcabb1-42f3-11de-af74-002332c672e6")); // flamingo
taxonIdHistory.add(new Id("urn:tidalwave:taxo/concept#4afc7a00-42f3-11de-af74-002332c672e6")); // mallard
taxonIdHistory.add(new Id("urn:tidalwave:taxo/concept#4ecf2ce0-42f3-11de-af74-002332c672e6")); // sanderling
taxonIdHistory.add(new Id("urn:tidalwave:taxo/concept#4ed0da90-42f3-11de-af74-002332c672e6")); // dunlin
taxonIdHistory.add(new Id("urn:tidalwave:taxo/concept#4d3d9060-42f3-11de-af74-002332c672e6")); // white egret
taxonIdHistory.add(new Id("urn:tidalwave:taxo/concept#4d2351a0-42f3-11de-af74-002332c672e6")); // cormorant
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public synchronized Observation.Builder getBuilder()
{
if (builder == null)
{
final ObservationSet observationSet = Locator.find(ObservationManager.class).findOrCreateObservationSetById(null);
builder = observationSet.createObservation();
}
return builder;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public void clear()
{
builder = null;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public void setBuilder (final @Nonnull Builder builder)
{
this.builder = builder;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public void addToTaxonHistory (final @Nonnull Taxon taxon)
{
final Id id = taxon.getId();
taxonIdHistory.remove(id);
taxonIdHistory.add(0, id);
while (taxonIdHistory.size() > maxHistorySize)
{
taxonIdHistory.remove(maxHistorySize);
}
save();
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public List getRecentTaxa()
{
final List taxa = new ArrayList();
final Taxonomy taxonomy = Locator.find(TaxonomyPreferences.class).getTaxonomy();
for (final Id taxonId : taxonIdHistory)
{
try
{
taxa.add(taxonomy.findTaxonById(taxonId));
}
catch (NotFoundException e)
{
logger.warning("Can't retrieve taxon for id: %s", taxonId);
}
}
return taxa;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public void clearTaxonHistory()
{
taxonIdHistory.clear();
save();
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private void load()
{
try
{
final ObjectInputStream ois = new ObjectInputStream(fileSystem.openFileInput(NAME));
taxonIdHistory = (List)ois.readObject();
ois.close();
}
catch (Exception e)
{
taxonIdHistory = new ArrayList();
logger.throwing("load()", CLASS, e);
logger.warning("Can't load history: "+ e);
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private void save()
{
try
{
final ObjectOutputStream oos = new ObjectOutputStream(fileSystem.openFileOutput(NAME));
oos.writeObject(taxonIdHistory);
oos.close();
}
catch (IOException e)
{
logger.throwing("save()", CLASS, e);
logger.severe("Can't save history: "+ e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy