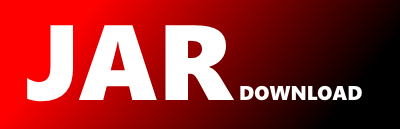
it.tidalwave.bluebill.mobile.taxonomy.TaxonCountFormat Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - open source birdwatching
* ==========================================
*
* Copyright (C) 2009, 2010 by Tidalwave s.a.s. (http://www.tidalwave.it)
* http://bluebill.tidalwave.it/mobile/
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* $Id: TaxonCountFormat.java,v 3e7ea5dbefcb 2010/05/17 15:10:54 fabrizio $
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.mobile.taxonomy;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import java.text.ChoiceFormat;
import org.openide.util.NbBundle;
import it.tidalwave.bluebill.taxonomy.Taxonomy.Type;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id: $
*
**********************************************************************************************************************/
public class TaxonCountFormat
{
protected int initializationCount = 0; // for testing
@CheckForNull
protected Locale locale;
@Nonnull
private final Type type;
private ChoiceFormat choice;
public TaxonCountFormat (final @Nonnull Type type)
{
this.type = type;
}
@Nonnull
public String format (final int n)
{
initialize();
return String.format(choice.format(n), n);
}
private synchronized void initialize()
{
if ((locale == null) || !locale.equals(Locale.getDefault()))
{
initializationCount++;
locale = Locale.getDefault();
final Map maleQuantifierMap = new HashMap();
final Map femaleQuantifierMap = new HashMap();
final Map singularMap = new HashMap();
final Map pluralMap = new HashMap();
Map cardinalityForZero = null;
maleQuantifierMap.put(0, NbBundle.getMessage(TaxonCountFormat.class, "maleNone"));
maleQuantifierMap.put(1, NbBundle.getMessage(TaxonCountFormat.class, "maleOne"));
femaleQuantifierMap.put(0, NbBundle.getMessage(TaxonCountFormat.class, "femaleNone"));
femaleQuantifierMap.put(1, NbBundle.getMessage(TaxonCountFormat.class, "femaleOne"));
singularMap.put(Type.CLASS, NbBundle.getMessage(TaxonCountFormat.class, "class"));
pluralMap. put(Type.CLASS, NbBundle.getMessage(TaxonCountFormat.class, "classes"));
singularMap.put(Type.FAMILY, NbBundle.getMessage(TaxonCountFormat.class, "family"));
pluralMap. put(Type.FAMILY, NbBundle.getMessage(TaxonCountFormat.class, "families"));
singularMap.put(Type.GENUS, NbBundle.getMessage(TaxonCountFormat.class, "genus"));
pluralMap. put(Type.GENUS, NbBundle.getMessage(TaxonCountFormat.class, "genera"));
singularMap.put(Type.ORDER, NbBundle.getMessage(TaxonCountFormat.class, "order"));
pluralMap. put(Type.ORDER, NbBundle.getMessage(TaxonCountFormat.class, "orders"));
singularMap.put(Type.SPECIES, NbBundle.getMessage(TaxonCountFormat.class, "species"));
pluralMap. put(Type.SPECIES, NbBundle.getMessage(TaxonCountFormat.class, "speciesPlural"));
singularMap.put(Type.SUBSPECIES, NbBundle.getMessage(TaxonCountFormat.class, "subspecies"));
pluralMap. put(Type.SUBSPECIES, NbBundle.getMessage(TaxonCountFormat.class, "subspeciesPlural"));
if (locale.getLanguage().equals("it"))
{
cardinalityForZero = singularMap;
}
else // english or fallback
{
cardinalityForZero = pluralMap;
}
final double[] limits = { 0, 1, 2 };
final Map quantifierMap = type.equals(Type.GENUS) || type.equals(Type.ORDER) ?
maleQuantifierMap : femaleQuantifierMap;
final String[] parts =
{
quantifierMap.get(0) + " " + cardinalityForZero.get(type),
quantifierMap.get(1) + " " + singularMap.get(type),
"%d " + pluralMap.get(type)
};
choice = new ChoiceFormat(limits, parts);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy