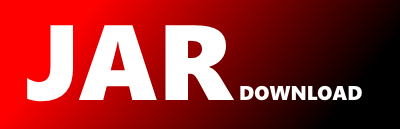
it.tidalwave.bluebill.stats.domain.JpaPing Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Stats
* Copyright (C) 2011-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluebill.tidalwave.it/mobile/
* SCM: http://java.net/hg/bluebill-server~stats-src
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.stats.domain;
import java.util.Date;
import javax.annotation.Nonnull;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import lombok.ToString;
import org.joda.time.DateTime;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@Entity @Table(name="PING")
@AllArgsConstructor @NoArgsConstructor @Getter @Setter @ToString
public class JpaPing
{
@Id @Column(length=40)
private String id;
@Temporal(TemporalType.TIMESTAMP) @Column(name="TIMESTAMP_")
private Date timeStamp;
@Column(length=15)
private String ipAddress;
@Column(length=40)
private String installId;
@Column(length=40)
private String appVersion;
@Column(length=40)
private String osVersion;
@Column(length=10)
private String javaVersion;
@Column(length=5)
private String userLanguage;
@Column(length=5)
private String userRegion;
@Column(length=40)
private String board;
@Column(length=40)
private String brand;
@Column(length=40)
private String device;
@Column(length=40)
private String model;
@Column(length=40)
private String product;
@Column(length=40)
private String versionIncremental;
@Column(length=40)
private String versionRelease;
private Integer versionSDK;
public JpaPing (final @Nonnull Ping ping, final @Nonnull String id)
{
this.id = id;
timeStamp = ping.getTimestamp().toDate();
ipAddress = ping.getIpAddress();
installId = ping.getInstallId();
appVersion = ping.getAppVersion();
osVersion = ping.getOsVersion();
javaVersion = ping.getJavaVersion();
userLanguage = ping.getUserLanguage();
userRegion = ping.getUserRegion();
board = ping.getBoard();
brand = ping.getBrand();
device = ping.getDevice();
model = ping.getModel();
product = ping.getProduct();
versionIncremental = ping.getVersionIncremental();
versionRelease = ping.getVersionRelease();
versionSDK = Integer.parseInt(ping.getVersionSDK());
}
@Nonnull
public Ping toPing()
{
return new Ping(new DateTime(timeStamp.getTime()),
ipAddress,
installId,
appVersion,
osVersion,
javaVersion,
userLanguage,
userRegion,
board,
brand,
device,
model,
product,
versionIncremental,
versionRelease,
"" + versionSDK);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy