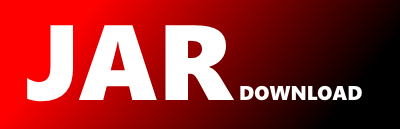
it.tidalwave.bluebill.stats.domain.jpa.JpaPingFinder Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Stats
* Copyright (C) 2011-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluebill.tidalwave.it/mobile/
* SCM: http://java.net/hg/bluebill-server~stats-src
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.stats.domain.jpa;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.persistence.Query;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import it.tidalwave.bluebill.stats.domain.JpaPing;
import it.tidalwave.bluebill.stats.domain.Ping;
import it.tidalwave.bluebill.stats.domain.PingFinder;
import it.tidalwave.util.SortCriterionDeclaration;
import it.tidalwave.util.spi.SimpleFinderSupport;
import org.springframework.transaction.annotation.Transactional;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id: JpaPingFinder.java,v 7a52f33be2b5 2011/07/15 23:33:19 fabrizio $
*
**********************************************************************************************************************/
@RequiredArgsConstructor @Slf4j
public class JpaPingFinder extends SimpleFinderSupport implements PingFinder
{
private static final SortCriterion BY_TIMESTAMP_IMPL = new SortCriterionDeclaration("timeStamp");
private static final SortCriterion BY_OS_VERSION_IMPL = new SortCriterionDeclaration("osVersion");
private static final SortCriterion BY_MODEL_IMPL = new SortCriterionDeclaration("model");
private static final SortCriterion BY_INSTALL_ID_IMPL = new SortCriterionDeclaration("installId");
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private static final Map sortCriterionImplementationMap = new HashMap()
{{
put(PingFinder.BY_TIMESTAMP, BY_TIMESTAMP_IMPL);
put(PingFinder.BY_OS_VERSION, BY_OS_VERSION_IMPL);
put(PingFinder.BY_MODEL, BY_MODEL_IMPL);
put(PingFinder.BY_INSTALL_ID, BY_INSTALL_ID_IMPL);
}};
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@RequiredArgsConstructor
static class JpaSorter
{
@Nonnull
private final SortCriterion sortCriterion;
@Nonnull
private final SortDirection sortDirection;
public String toJpaQl()
{
return "p." + sortCriterion + ((sortDirection == SortDirection.ASCENDING) ? "" : " DESC");
}
}
@Nonnull
private final JpaPingDao dao;
@Nonnull
protected final List sorters = new ArrayList();
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public PingFinder sort (final @Nonnull SortCriterion criterion,
final @Nonnull SortDirection direction)
{
final SortCriterion criterionImpl = sortCriterionImplementationMap.get(criterion);
if (criterionImpl == null)
{
throw new IllegalArgumentException("Unsupported sortCriterion: " + criterion);
}
final JpaPingFinder clone = (JpaPingFinder)clone();
clone.sorters.add(new JpaSorter(criterionImpl, direction));
return clone;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnegative
public int count()
{
final String jpaQl = "SELECT count(p) FROM JpaPing p";
log.debug(jpaQl);
return ((Number)createQuery(jpaQl).getSingleResult()).intValue();
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Transactional @Nonnull
protected List extends Ping> computeNeededResults()
{
final StringBuilder builder = new StringBuilder("FROM JpaPing p");
String leading = " ORDER BY ";
String trailing = "";
for (final JpaSorter sorter : sorters)
{
builder.append(leading).append(sorter.toJpaQl()).append(trailing);
leading = "";
trailing = ", ";
}
log.debug(">>>> jpa query: {} [{}, x{}]", new Object[] { builder, firstResult, maxResults });
final Query query = createQuery(builder.toString());
final List pings = new ArrayList();
final List jpaPings = query.getResultList();
log.debug(">>>> retrieved {} records", jpaPings.size());
for (final JpaPing jpaPing : jpaPings)
{
pings.add(jpaPing.toPing());
}
return pings;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnull
private Query createQuery (final @Nonnull String ql)
{
return dao.createQuery(ql).setFirstResult(firstResult).setMaxResults(maxResults);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy