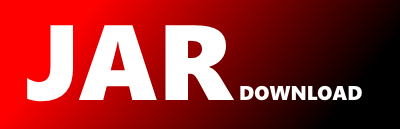
it.tidalwave.bluebill.taxonomy.elmo.impl.ElmoTaxonomyConcept Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - open source birdwatching
* ==========================================
*
* Copyright (C) 2009, 2010 by Tidalwave s.a.s. (http://www.tidalwave.it)
* http://bluebill.tidalwave.it/mobile/
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* $Id: ElmoTaxonomyConcept.java,v 9827802063b2 2010/07/17 22:18:31 fabrizio $
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.taxonomy.elmo.impl;
import it.tidalwave.bluebill.taxonomy.AnonymousTaxon;
import it.tidalwave.util.NotFoundException;
import java.util.Locale;
import java.util.Map;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.List;
import javax.xml.namespace.QName;
import org.openrdf.model.Statement;
import org.openrdf.repository.Repository;
import org.openrdf.elmo.annotations.rdf;
import org.openrdf.elmo.Entity;
import org.openrdf.concepts.skos.core.Concept;
import org.openrdf.concepts.skos.core.ConceptScheme;
import it.tidalwave.openrdf.elmo.RDFUtils;
import it.tidalwave.bluebill.taxonomy.Finder;
import it.tidalwave.bluebill.taxonomy.Taxonomy;
import it.tidalwave.bluebill.taxonomy.TaxonomyConcept;
import it.tidalwave.bluebill.taxonomy.elmo.ElmoTaxonomyVocabulary;
import it.tidalwave.openrdf.elmo.ElmoManagerThreadLocal;
import it.tidalwave.util.logging.Logger;
import java.util.Collections;
import java.util.Iterator;
import java.util.Set;
import javax.annotation.CheckForNull;
import org.openrdf.elmo.ResourceManager;
import org.openrdf.elmo.sesame.SesameManager;
import org.openrdf.model.Resource;
import org.openrdf.model.URI;
import org.openrdf.model.impl.URIImpl;
import org.openrdf.repository.RepositoryException;
import org.openrdf.repository.RepositoryResult;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id: ElmoTaxonomyConcept.java,v 9827802063b2 2010/07/17 22:18:31 fabrizio $
*
**********************************************************************************************************************/
@rdf(ElmoTaxonomyVocabulary.URI_TAXONOMY_CONCEPT)
public class ElmoTaxonomyConcept extends Support implements InternalElmoTaxonomyConcept
{
private static final String CLASS = ElmoTaxonomyConcept.class.getName();
private static final Logger logger = Logger.getLogger(CLASS);
@Nonnull
private Repository repository;
@Nonnull
private Taxonomy taxonomy;
// @rdf(ElmoTaxonomyVocabulary.URI_TAXONOMY_SYNONYM)
@CheckForNull
private AnonymousTaxon synonym;
/*******************************************************************************************************************
*
*
*
******************************************************************************************************************/
public ElmoTaxonomyConcept (@Nonnull final Entity entity)
{
super(entity);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnull
@Override
public TaxonomyConcept createSubConcept (@Nonnull final String displayName,
@Nonnull final String id)
{
logger.finest("createSubConcept(%s, %s)", displayName, id);
final TaxonomyConcept taxonomyConcept = taxonomy.createConcept(displayName, id);
final Concept skosChild = taxonomyConcept.getLookup().lookup(Concept.class);
final Concept skosParent = getLookup().lookup(Concept.class);
skosParent.getSkosNarrowers().add(skosChild);
// as(Concept.class).getSkosNarrowers().add(taxonomyConcept.as(Concept.class));
return taxonomyConcept;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnull
@Override
public Finder findSubConcepts()
{
try
{
logger.finest("findSubConcepts() - %s", getDisplayName());
final List result = new ArrayList();
for (final Concept skosConcept : as(Concept.class).getSkosNarrowers())
{
final QName id = skosConcept.getQName();
final InternalElmoTaxonomyConcept concept = ((ElmoTaxonomy)taxonomy).conceptFactory.find(id);
// final ElmoTaxonomyConcept concept = (ElmoTaxonomyConcept)skosConcept;
concept.setBindings(repository, taxonomy);
result.add(concept);
}
return new YYYFinder(result);
}
catch (NotFoundException e)
{
throw new RuntimeException(e);
}
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void setAnonymousSynonym (@Nonnull final AnonymousTaxon synonym)
{
this.synonym = synonym;
final SesameManager em = (SesameManager)ElmoManagerThreadLocal.get();
final ResourceManager rm = em.getResourceManager();
try // FIXME: workaround because the @rdf annotation of this.synonym doesn't work
{
em.getConnection().add(rm.createResource(getLookup().lookup(Entity.class).getQName()),
new URIImpl(ElmoTaxonomyVocabulary.URI_TAXONOMY_SYNONYM),
rm.createResource(synonym.getLookup().lookup(Entity.class).getQName())); // FIXME
}
catch (RepositoryException e)
{
throw new RuntimeException(e);
}
// final Concept asConcept = synonym.getLookup().lookup(Concept.class); // FIXME: as() doesn't work
// asConcept.getSkosNotes().add("debugging-info: " + getLookup().lookup(Entity.class).getQName().getLocalPart()); // For debugging only FIXME: use as()
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public AnonymousTaxon getAnonymousSynonym()
throws NotFoundException
{
try // FIXME: workaround because the @rdf annotation of this.synonym doesn't work
{
final SesameManager em = (SesameManager)ElmoManagerThreadLocal.get();
final ResourceManager rm = em.getResourceManager();
final RepositoryResult triples = em.getConnection().getStatements(rm.createResource(getLookup().lookup(Entity.class).getQName()),
new URIImpl(ElmoTaxonomyVocabulary.URI_TAXONOMY_SYNONYM),
null);
if (!triples.hasNext())
{
throw new NotFoundException("No synonyms");
}
final Statement triple = triples.next();
final URI uri = (URI)triple.getObject();
return taxonomy.findOrCreateAnonymousTaxon(uri.stringValue());
}
catch (RepositoryException e)
{
throw new RuntimeException(e);
}
// if (synonym == null)
// {
// throw new NotFoundException("No synonyms.");
// }
//
// return synonym;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public Object getSynonyms()
{
try
{
final Set synonyms = (Set)getAnonymousSynonym().getSynonyms();
for (final Iterator i = synonyms.iterator(); i.hasNext(); )
{
// if (this.equals(i.next())) FIXME
if (as(Entity.class).getQName().equals(i.next().as(Entity.class).getQName()))
{
i.remove();
break;
}
}
return synonyms;
}
catch (NotFoundException e)
{
return Collections.emptySet();
}
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void init (@Nonnull final String displayName,
@Nonnull final Taxonomy taxonomy)
{
logger.finest("init(%s, %s) - @%x", displayName, taxonomy, System.identityHashCode(this));
RDFUtils.setDisplayName(entity, displayName);
final Concept skosConcept = as(Concept.class);
skosConcept.getSkosInSchemes().add(taxonomy.as(ConceptScheme.class));
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void setBindings (@Nonnull final Repository repository,
@Nonnull final Taxonomy taxonomy)
{
logger.finest("setBindings(%s, %s) - @%x", repository, taxonomy, System.identityHashCode(this));
this.taxonomy = taxonomy;
this.repository = repository;
}
public Map getDisplayNames()
{
throw new UnsupportedOperationException("Not supported yet.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy