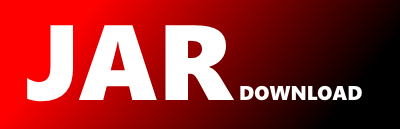
it.tidalwave.bluebill.taxonomy.elmo.impl.ElmoTaxonomyManager Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueBill Mobile - open source birdwatching
* ==========================================
*
* Copyright (C) 2009, 2010 by Tidalwave s.a.s. (http://www.tidalwave.it)
* http://bluebill.tidalwave.it/mobile/
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* $Id: ElmoTaxonomyManager.java,v 31ee4e8a62bc 2010/07/17 14:44:24 fabrizio $
*
**********************************************************************************************************************/
package it.tidalwave.bluebill.taxonomy.elmo.impl;
import it.tidalwave.util.NotFoundException;
import javax.annotation.Nonnull;
import javax.xml.namespace.QName;
import org.openide.util.Lookup;
import org.openrdf.elmo.ElmoManager;
import org.openrdf.elmo.ElmoQuery;
import org.openrdf.concepts.skos.core.ConceptScheme;
import org.openrdf.repository.Repository;
import it.tidalwave.openrdf.elmo.ElmoManagerProxy;
import it.tidalwave.openrdf.elmo.ElmoUtils;
import it.tidalwave.bluebill.taxonomy.Taxonomy;
import it.tidalwave.bluebill.taxonomy.TaxonomyManager;
import it.tidalwave.bluebill.taxonomy.elmo.ElmoTaxonomyVocabulary;
import it.tidalwave.openrdf.elmo.impl.ElmoEntityFactory;
import it.tidalwave.semantic.EntityFactory;
import it.tidalwave.semantic.Wrapper;
import it.tidalwave.util.logging.Logger;
import org.openide.util.lookup.ServiceProvider;
import org.openrdf.elmo.Entity;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id: ElmoTaxonomyManager.java,v 31ee4e8a62bc 2010/07/17 14:44:24 fabrizio $
*
**********************************************************************************************************************/
@ServiceProvider(service=TaxonomyManager.class)
public class ElmoTaxonomyManager implements TaxonomyManager
{
private static final String CLASS = ElmoTaxonomyManager.class.getName();
private static final Logger logger = Logger.getLogger(CLASS);
private static final String QUERY_TAXONOMY_BY_NAME = String.format(
"SELECT ?taxonomy WHERE " +
" { " +
" ?taxonomy a <%s> ;" +
" ?name ." +
// " ?name ." +
" }", ElmoTaxonomyVocabulary.URI_TAXONOMY);
private Lookup lookup;
private final ElmoManager em = new ElmoManagerProxy();
private final EntityFactory taxonomyFactory =
new ElmoEntityFactory(InternalElmoTaxonomy.class,
ElmoTaxonomy.class,
ConceptScheme.class);
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnull
@Override
public Taxonomy createTaxonomy (@Nonnull final String displayName,
@Nonnull final String id,
@Nonnull final Object repository)
{
final QName qName = new QName(id);
final InternalElmoTaxonomy taxonomy = taxonomyFactory.create(qName);
taxonomy.setBindings((Repository)repository);
taxonomy.init(displayName);
return taxonomy;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public Taxonomy findTaxonomyByName (@Nonnull final String name,
@Nonnull final Object repository)
throws NotFoundException
{
logger.fine("findTaxonomyByName(%s, %s)", name, repository);
// FIXME: handle the repository!
final ElmoQuery query = em.createQuery(QUERY_TAXONOMY_BY_NAME).setParameter("name", name);
final Entity entity = (Entity)query.getSingleResult();
final InternalElmoTaxonomy taxonomy = taxonomyFactory.find(entity.getQName());
// final ElmoTaxonomy taxonomy = (ElmoTaxonomy)query.getSingleResult();
taxonomy.setBindings((Repository)repository);
return Wrapper.wrap(taxonomy);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnull
@Override
public synchronized Lookup getLookup()
{
if (lookup == null)
{
lookup = ElmoUtils.createLookup(this);
}
return lookup;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy