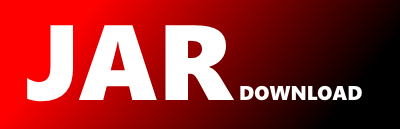
it.tidalwave.xml.jaxp.JaxpXPath Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueBill Core - open source birding
* Copyright (C) 2009-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluebill.tidalwave.it
* SCM: https://java.net/hg/bluebill~core-src
*
**********************************************************************************************************************/
package it.tidalwave.xml.jaxp;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.annotation.CheckForNull;
import javax.xml.XMLConstants;
import javax.xml.xpath.XPathConstants;
import javax.xml.xpath.XPathExpression;
import javax.xml.xpath.XPathExpressionException;
import javax.xml.xpath.XPathFactory;
import org.w3c.dom.NodeList;
import org.jaxen.FunctionContext;
import org.jaxen.JaxenException;
import org.jaxen.NamespaceContext;
import org.jaxen.Navigator;
import org.jaxen.VariableContext;
import org.jaxen.XPath;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public class JaxpXPath implements XPath
{
private final static javax.xml.xpath.XPath JAXP_XPATH;
private final XPathExpression xPathExpression;
private final static Map PREFIX_TO_NAMESPACE_MAP = new HashMap();
private final static Map NAMESPACE_TO_PREFIX_MAP = new HashMap();
static
{
final XPathFactory xPathFactory = XPathFactory.newInstance();
JAXP_XPATH = xPathFactory.newXPath();
JAXP_XPATH.setNamespaceContext(new javax.xml.namespace.NamespaceContext()
{
@Nonnull
public String getNamespaceURI (final @Nonnull String prefix)
{
final String nameSpace = PREFIX_TO_NAMESPACE_MAP.get(prefix);
return (nameSpace != null) ? nameSpace : XMLConstants.NULL_NS_URI;
}
@CheckForNull
public String getPrefix (final @Nonnull String nameSpace)
{
return NAMESPACE_TO_PREFIX_MAP.get(nameSpace);
}
@Nonnull
public Iterator getPrefixes (final @Nonnull String nameSpace)
{
final String prefix = getPrefix(nameSpace);
return ((prefix == null) ? Collections.emptyList() : Collections.singletonList(prefix)).iterator();
}
});
PREFIX_TO_NAMESPACE_MAP.put("html", "http://www.w3.org/1999/xhtml");
NAMESPACE_TO_PREFIX_MAP.put("http://www.w3.org/1999/xhtml", "html");
}
public JaxpXPath (final @Nonnull String expression)
throws JaxenException
{
try
{
xPathExpression = JAXP_XPATH.compile(expression);
}
catch (XPathExpressionException e)
{
throw new JaxenException(e);
}
}
public Object evaluate (final @Nonnull Object o)
throws JaxenException
{
try
{
return xPathExpression.evaluate(o);
}
catch (XPathExpressionException e)
{
throw new JaxenException(e);
}
}
@Deprecated
public String valueOf (final @Nonnull Object o)
throws JaxenException
{
return stringValueOf(o);
}
public String stringValueOf (final @Nonnull Object o)
throws JaxenException
{
try
{
return (String)xPathExpression.evaluate(o, XPathConstants.STRING);
}
catch (XPathExpressionException e)
{
throw new JaxenException(e);
}
}
public boolean booleanValueOf (final @Nonnull Object o)
throws JaxenException
{
try
{
return (Boolean)xPathExpression.evaluate(o, XPathConstants.BOOLEAN);
}
catch (XPathExpressionException e)
{
throw new JaxenException(e);
}
}
public Number numberValueOf (final @Nonnull Object o)
throws JaxenException
{
try
{
return (Number)xPathExpression.evaluate(o, XPathConstants.NUMBER);
}
catch (XPathExpressionException e)
{
throw new JaxenException(e);
}
}
public List selectNodes (final @Nonnull Object o)
throws JaxenException
{
try
{
final NodeList nodeList = (NodeList)xPathExpression.evaluate(o, XPathConstants.NODESET);
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy