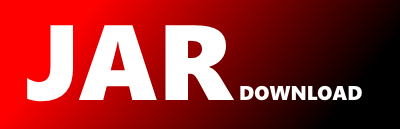
it.tidalwave.forceten.lookandfeel.impl.LookAndFeelModule Maven / Gradle / Ivy
/*******************************************************************************
*
* blueMarine - open source photo workflow
* =======================================
*
* Copyright (C) 2003-2009 by Fabrizio Giudici
* Project home page: http://bluemarine.tidalwave.it
*
*******************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*******************************************************************************
*
* $Id: LookAndFeelModule.java,v d7f0b33228ba 2009/12/19 11:59:30 fabrizio $
*
******************************************************************************/
package it.tidalwave.forceten.lookandfeel.impl;
import java.util.logging.Logger;
import java.awt.Color;
import java.awt.Font;
import javax.swing.JPopupMenu;
import javax.swing.UIManager;
import javax.swing.text.Style;
import javax.swing.text.StyleConstants;
import javax.swing.text.html.HTMLEditorKit;
import javax.swing.text.html.StyleSheet;
import org.jdesktop.swingx.JXDatePicker;
import org.openide.util.Utilities;
import org.openide.modules.ModuleInstall;
import it.tidalwave.forceten.lookandfeel.LookAndFeel;
// This was originally for fixing BM407, but now also serves to apply the proper gap to header panels.
// FIXME
//class PachedLayoutStyle extends LayoutStyle
// {
// private final LayoutStyle style;
//
// public PachedLayoutStyle (final LayoutStyle style)
// {
// this.style = style;
// }
//
// @Override
// public int getPreferredGap (final JComponent component1, final JComponent component2, final int type, final int position, final Container parent)
// {
// if (isHeaderPanel(component1) || isHeaderPanel(component2))
// {
// return 0;
// }
//
// return super.getPreferredGap(component1, component2, type, position, parent);
// }
//
// @Override
// public int getContainerGap (final JComponent component, final int position, final Container parent)
// {
// return super.getContainerGap(component, position, parent);
// }
//
// private boolean isHeaderPanel (final JComponent component)
// {
// return false;
//// final Boolean b = (Boolean)component.getClientProperty(HeaderPanelUI.HEADER_PANEL_CP);
//// return (b != null) ? b : false;
// }
// }
/*******************************************************************************
*
* @author Fabrizio Giudici
* @version $Id: LookAndFeelModule.java,v d7f0b33228ba 2009/12/19 11:59:30 fabrizio $
*
******************************************************************************/
public class LookAndFeelModule extends ModuleInstall
{
private static final String CLASS = LookAndFeelModule.class.getName();
private static final Logger logger = Logger.getLogger(CLASS);
private static final float FONT_HEADER_SCALE = 16.0F / 13.0F;
private static final float FONT_HEADER_SMALL_SCALE = 14.0F / 13.0F;
/***************************************************************************
*
* Installs the L&F settings at startup.
*
**************************************************************************/
@Override
public void restored()
{
super.restored();
// dumpDefaults();
logger.info("Installing look and feel...");
if (Utilities.getOperatingSystem() == Utilities.OS_MAC)
{
installQuaqua();
// installNimrod(); // only for testing
// Workaround for a bug in SwingX 2007_04_15, as there's a bad mapping for DatePickerUI on Mac OS X
// See https://swingx.dev.java.net/issues/show_bug.cgi?id=488
UIManager.put(JXDatePicker.uiClassID, "org.jdesktop.swingx.plaf.basic.BasicDatePickerUI");
}
else
{
// This is because NASA World Wind 0.2.0 use a heavyweight component that hides lightweight popups
JPopupMenu.setDefaultLightWeightPopupEnabled(false);
installNimrod();
// installNimbus();
}
// UIManager.put("ScrollBarUI", BlueMarineScrollBarUI.class.getName());
// FIXME LayoutStyle.setSharedInstance(new PachedLayoutStyle(LayoutStyle.getSharedInstance()));
// int borderSize = 2;
// Border border = BorderFactory.createEmptyBorder(0, borderSize, 0, borderSize);
// UIManager.put("ToolBar.rolloverBorder", border);
// UIManager.put("ToolBar.nonrolloverBorder", border);
if (!Boolean.getBoolean("it.tidalwave.bluemarine.showtabs"))
{
installEmptyComponentTabs();
}
installFonts();
installBlueMarineTheme();
installBlackTheme();
}
/***************************************************************************
*
* Installs the Quaqua L&F.
*
**************************************************************************/
private void installQuaqua()
{
if (!Boolean.getBoolean("it.tidalwave.bluemarine.disableQuaua"))
{
final String lafClassName = "ch.randelshofer.quaqua.QuaquaLookAndFeel";
// final Object save = UIManager.get("LayoutStyle.instance");
// System.setProperty("Quaqua.opaque", "true");
// System.setProperty("Quaqua.Table.style", "striped");
// System.setProperty("Quaqua.Tree.style", "striped");
// UIManager.put("OptionPaneUI", "it.tidalwave.bluemarine.lookandfeel.quaqua.BMQuaquaOptionPaneUI");
installLookAndFeel(lafClassName);
// UIManager.put("ScrollBarUI", BMQuaquaScrollBarUI.class.getName());
// UIManager.put("OptionPaneUI", BMQuaquaOptionPaneUI.class.getName());
// UIManager.put("TreeUI", BMQuaquaTreeUI.class.getName());
// UIManager.put("Nb.Toolbar.ui", BMQuaquaToolBarUI.class.getName());
// FIXME: the one below does not work
// UIManager.put("ToolBarSeparatorUI", BMQuaquaToolBarSeparatorUI.class.getName());
final Object icon = javax.swing.LookAndFeel.makeIcon(getClass(), "Icon-64.png");
UIManager.put("OptionPane.errorIcon", icon);
UIManager.put("OptionPane.informationIcon", icon);
UIManager.put("OptionPane.questionIcon", icon);
UIManager.put("OptionPane.warningIcon", icon);
}
}
/***************************************************************************
*
* Installs the Nimbus L&F.
*
**************************************************************************/
private void installNimbus()
{
installLookAndFeel("org.jdesktop.swingx.plaf.nimbus.NimbusLookAndFeel");
UIManager.put("Nb.Toolbar.ui", "javax.swing.plaf.basic.BasicToolBarUI");
// UIManager.put("ScrollBarUI", BlueMarineScrollBarUI.class.getName());
installDefaultDarkUISettings();
}
/***************************************************************************
*
* Installs the Nimrod L&F.
*
**************************************************************************/
private void installNimrod()
{
System.setProperty("nimrodlf.p1", "#808080");
System.setProperty("nimrodlf.p2", "#a0a0a0");
System.setProperty("nimrodlf.p3", "#c0c0c0");
System.setProperty("nimrodlf.s1", "#525252");
System.setProperty("nimrodlf.s2", "#5C5C5C");
System.setProperty("nimrodlf.s3", "#666666");
System.setProperty("nimrodlf.w", "#000000");
System.setProperty("nimrodlf.b", "#FFFFFF");
System.setProperty("nimrodlf.menuOpacity", "195");
System.setProperty("nimrodlf.frameOpacity", "180");
installLookAndFeel("com.nilo.plaf.nimrod.NimRODLookAndFeel");
UIManager.put("Nb.Toolbar.ui", "javax.swing.plaf.basic.BasicToolBarUI");
// UIManager.put("ScrollBarUI", BlueMarineScrollBarUI.class.getName());
installDefaultDarkUISettings();
}
/***************************************************************************
*
* Installs a L&F specified by the given class name.
*
* @param lafClassName the L&F class name
*
**************************************************************************/
private void installLookAndFeel (final String lafClassName)
{
try
{
UIManager.setLookAndFeel(lafClassName);
}
catch (Exception e)
{
logger.throwing(CLASS, "installLookAndFeel()", e);
}
}
/***************************************************************************
*
* Installs the UI components that remove the tabs from NetBeans.
*
**************************************************************************/
private void installEmptyComponentTabs()
{
// UIManager.put("EditorTabDisplayerUI", NoTabsTabDisplayerUI.class.getName());
// UIManager.put("ViewTabDisplayerUI", NoTabsTabDisplayerUI.class.getName());
// UIManager.put("TabbedContainer.editor.contentBorder", BorderFactory.createEmptyBorder());
// UIManager.put("TabbedContainer.view.contentBorder", BorderFactory.createEmptyBorder());
}
/***************************************************************************
*
* Installs the UIManager defaults for the blueMarine color theme.
*
**************************************************************************/
private void installBlueMarineTheme()
{
// UIManager.put(LookAndFeel.UI_HEADER_PANEL, new HeaderPanelUI());
// UIManager.put(LookAndFeel.UI_DARK_VIEW_PANEL, new DarkViewPanelUI());
// Light theme - not used any longer, if you want to re-enable it, you have to complete the settings.
UIManager.put(LookAndFeel.COLOR_DESKTOP_BACKGROUND, new Color(100, 100, 100));
UIManager.put(LookAndFeel.COLOR_EXPLORER_BACKGROUND, new Color(210, 210, 210));
UIManager.put(LookAndFeel.COLOR_EXPLORER_FOREGROUND, new Color(40, 40, 40));
UIManager.put(LookAndFeel.COLOR_HEADER_FOREGROUND, new Color(0, 0, 0));
UIManager.put(LookAndFeel.COLOR_HEADER_BACKGROUND, new Color(185, 185, 185));
UIManager.put(LookAndFeel.COLOR_HEADER_DARK_FOREGROUND, new Color(140, 140, 140));
UIManager.put(LookAndFeel.COLOR_HEADER_DARK_FOREGROUND2, new Color(200, 200, 200));
UIManager.put(LookAndFeel.COLOR_HEADER_DARK_BACKGROUND, new Color(25, 25, 25));
// //
// // The default renderer would drastically choose BLACK or WHITE if there's not enough contrast
// // between the background and the foreround. See a comment in http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=5069799
// // We disable it, so we take control of the colors.
// //
// System.setProperty("nb.useSwingHtmlRendering", "true");
// UIManager.put(LookAndFeel.COLOR_FRAME_FOREGROUND, new Color(28, 28, 28));
// UIManager.put(LookAndFeel.COLOR_DESKTOP_BACKGROUND, new Color(100, 100, 100));
// UIManager.put(LookAndFeel.COLOR_EXPLORER_BACKGROUND, new Color(86, 86, 86));
// UIManager.put(LookAndFeel.COLOR_EXPLORER_FOREGROUND, new Color(210, 210, 210));
// UIManager.put(LookAndFeel.COLOR_EXPLORER_SELECTION_FOREGROUND, new Color(15, 15, 15));
// UIManager.put(LookAndFeel.COLOR_EXPLORER_SELECTION_BACKGROUND, new Color(208, 208, 208));
// UIManager.put(LookAndFeel.COLOR_HEADER_FOREGROUND, new Color(178, 178, 178));
// UIManager.put(LookAndFeel.COLOR_HEADER_BACKGROUND, new Color(65, 65, 65));
// UIManager.put(LookAndFeel.COLOR_HEADER_DARK_FOREGROUND, new Color(140, 140, 140));
// UIManager.put(LookAndFeel.COLOR_HEADER_DARK_FOREGROUND2, new Color(200, 200, 200));
// UIManager.put(LookAndFeel.COLOR_HEADER_DARK_BACKGROUND, new Color(25, 25, 25));
//// UIManager.put(LookAndFeel.BORDER_EXPLORER, new ExplorerPanelBorder());
//// UIManager.put("it.tidalwave.openide.explorer.scrollbar.darkenerColor", new Color(0, 0, 0, 128));
// UIManager.put("ScrollBar.thumb", new Color(90, 90, 90));
// UIManager.put("ScrollBar.track", new Color(64, 64, 64));
//// UIManager.put("nbProgressBar.Foreground", Color.RED); useless
}
/***************************************************************************
*
*
**************************************************************************/
private void installDefaultDarkUISettings()
throws NumberFormatException
{
UIManager.put("List.background", Color.decode("#777777"));
UIManager.put("Tree.background", Color.decode("#777777"));
UIManager.put("Tree.textBackground", Color.decode("#777777"));
UIManager.put("Table.background", Color.decode("#777777"));
UIManager.put("TextField.background", Color.decode("#777777"));
UIManager.put("EditorPane.background", Color.decode("#666666"));
UIManager.put("EditorPane.foreground", Color.decode("#ffffff"));
UIManager.put("TextPane.background", Color.decode("#666666"));
UIManager.put("TextPane.foreground", Color.decode("#ffffff"));
//
// This is for JTextPane and JEditorPane.
//
final HTMLEditorKit editorKit = new HTMLEditorKit();
// By default HTMLEditorKit comes with a shared StyleSheet.
// Changing it will affect all HTMLEditorKits.
final StyleSheet defaultStyleSheet = editorKit.getStyleSheet();
final Style bodyStyle = defaultStyleSheet.getStyle("body");
StyleConstants.setForeground(bodyStyle, Color.WHITE);
StyleConstants.setFontFamily(bodyStyle, "sansserif");
final Style aStyle = defaultStyleSheet.getStyle("a");
StyleConstants.setForeground(aStyle, Color.WHITE);
StyleConstants.setBold(aStyle, true);
StyleConstants.setUnderline(aStyle, false);
StyleConstants.setItalic(aStyle, true);
}
/***************************************************************************
*
*
**************************************************************************/
private void installFonts()
{
Font font = UIManager.getFont("Tree.font"); // reference font
if (Utilities.getOperatingSystem() == Utilities.OS_MAC)
{
// to try: Geeza pro, Lucida grande, Tahoma, Trebuchet MS
// These don't have bold: Geneva, Gulim, Open Symbol
font = new Font("Lucida grande", Font.PLAIN, font.getSize());
}
else if (Utilities.getOperatingSystem() == Utilities.OS_LINUX)
{
font = new Font("Arial", Font.PLAIN, font.getSize());
}
if (font != null)
{
float scale = 12.0f / 13.0f;
Font newFont = font.deriveFont(scale * font.getSize2D());
UIManager.put(LookAndFeel.FONT_EXPLORER, newFont);
UIManager.put(LookAndFeel.FONT_SMALL, newFont);
UIManager.put(LookAndFeel.FONT_HEADER, font.deriveFont(FONT_HEADER_SCALE * font.getSize2D()));
UIManager.put(LookAndFeel.FONT_HEADER_SMALL, font.deriveFont(FONT_HEADER_SMALL_SCALE * font.getSize2D()));
}
}
/***************************************************************************
*
*
**************************************************************************/
private void installBlackTheme()
{
// SwingUtilities.invokeLater(new Runnable()
// {
// public void run()
// {
// final Color color = UIManager.getColor(LookAndFeel.COLOR_FRAME_FOREGROUND);
// System.err.println("XXXCOL " + color);
// final Frame mainWindow = WindowManager.getDefault().getMainWindow();
//
// if (mainWindow.isVisible())
// {
// setBackgroundRecursively(mainWindow, color);
// }
//
// else
// {
// mainWindow.addComponentListener(new ComponentAdapter()
// {
// @Override
// public void componentShown (final ComponentEvent event)
// {
// setBackgroundRecursively(mainWindow, color);
// mainWindow.removeComponentListener(this);
// }
// });
// }
// }
// });
}
/***************************************************************************
*
*
**************************************************************************/
// private void setBackgroundRecursively (final Component component, final Color color)
// {
// final String className = component.getClass().getName();
//
// if (className.startsWith("org.openide.awt.MenuBar"))
// {
// return;
// }
//
//// if (component instanceof TopComponent) // refactor using className, --but include subclasses!--, drop import and module dependency
//// {
//// return;
//// }
//
// logger.fine("Setting background on: " + component);
// component.setBackground(color);
//
// if (component instanceof Container)
// {
// for (final Component child : ((Container)component).getComponents())
// {
// setBackgroundRecursively(child, color);
// }
// }
// }
/***************************************************************************
*
*
**************************************************************************/
// private void dumpDefaults()
// {
// logger.info("UIDefaults: ");
//
// for (final Object propName : new ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy