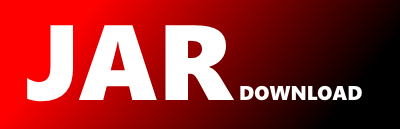
it.tidalwave.geo.geocoding.geonamesprovider.EarthGeoCoderEntity Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.geocoding.geonamesprovider;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Locale;
import java.util.SortedSet;
import java.util.Map;
import java.util.TreeSet;
import javax.xml.namespace.QName;
import org.openide.util.Lookup;
import org.openide.util.lookup.Lookups;
import it.tidalwave.geo.Coordinate;
import it.tidalwave.geo.geocoding.GeoCoder;
import it.tidalwave.geo.geocoding.GeoCoderEntity;
import it.tidalwave.geo.geocoding.GeoCoderManager;
import it.tidalwave.util.As;
import it.tidalwave.util.Finder;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.util.spi.SimpleFinderSupport;
import it.tidalwave.role.LocalizedDisplayable;
import it.tidalwave.netbeans.util.Locator;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
/* package */ class EarthGeoCoderEntity implements GeoCoderEntity
{
private static final long serialVersionUID = 2343453654745775845L;
private static final String ID = "6295630";
private final static Coordinate ZERO = new Coordinate(0, 0);
private static final String[] CONTINENT_CODES =
{
"6255146", "6255147", "6255148", "6255149", "6255151", "6255150", "6255152"
};
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private final static LocalizedDisplayable DISPLAYABLE = new LocalizedDisplayable() // FIXME: use DefaultDisplayable
{
@Override @Nonnull
public String getDisplayName()
{
return "Earth";
}
@Override @Nonnull
public String getDisplayName (final @Nonnull Locale locale)
{
return getDisplayName(); // FIXME
}
@Override @Nonnull
public SortedSet getLocales()
{
return new TreeSet(); // FIXME
}
@Override @Nonnull
public Map getDisplayNames()
{
throw new UnsupportedOperationException("Not supported yet.");
}
};
@CheckForNull @edu.umd.cs.findbugs.annotations.SuppressWarnings("SE_TRANSIENT_FIELD_NOT_RESTORED")
private transient GeoCoder geoCoder;
private Finder children;
private boolean childrenLoaded = false;
private final QName qName = new QName(GeoNamesProvider.URI_BASE + ID + "/");
@CheckForNull @edu.umd.cs.findbugs.annotations.SuppressWarnings("SE_TRANSIENT_FIELD_NOT_RESTORED")
private transient Lookup lookup;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public EarthGeoCoderEntity (final @Nonnull GeoCoder geoCoder)
{
this.geoCoder = geoCoder;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public synchronized GeoCoder getGeoCoder()
{
if (geoCoder == null)
{
try
{
geoCoder = Locator.find(GeoCoderManager.class).findGeoCoderByName("GeoNames");
}
catch (NotFoundException e)
{
throw new IllegalStateException(e);
}
}
return geoCoder;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String getId()
{
return ID;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public Coordinate getCoordinate()
{
return ZERO;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String getCode()
{
return "";
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String getTypeAsString()
{
return "PLANET";
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public Type getType()
{
return Type.PLANET;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public synchronized Finder findChildren()
{
if (!childrenLoaded)
{
childrenLoaded = true;
children = new SimpleFinderSupport("findChildren()")
{
@Override @Nonnull
protected List computeResults()
{
List result = new ArrayList();
for (final String continentCode : CONTINENT_CODES)
{
try
{
result.add(getGeoCoder().findGeoEntityById(continentCode));
}
catch (NotFoundException e)
{
}
}
Collections.sort(result);
return result;
}
};
}
return children;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public GeoCoderEntity getParent()
throws NotFoundException
{
throw new NotFoundException("Earth has got no parent");
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public boolean isParentSet()
{
return true;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public boolean equals (final @CheckForNull Object object)
{
if (object == null)
{
return false;
}
if (getClass() != object.getClass())
{
return false;
}
final EarthGeoCoderEntity other = (EarthGeoCoderEntity)object;
return getId().equals(other.getId());
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public int hashCode()
{
return ID.hashCode();
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public int compareTo (final @Nonnull GeoCoderEntity other)
{
// FIXME
return (this == other) ? 0 : +1;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String toString()
{
return String.format("EarthGeoCoderEntity@%x[]", System.identityHashCode(this));
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public T as (final @Nonnull Class clazz)
{
return as(clazz, As.Defaults.throwAsException(clazz));
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public T as (final @Nonnull Class clazz,
final @Nonnull NotFoundBehaviour notFoundBehaviour)
{
final T as = getLookup().lookup(clazz);
if (as != null)
{
return as;
}
else
{
return notFoundBehaviour.run(null);
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Override @Nonnull
public synchronized Lookup getLookup()
{
if (lookup == null) // also after serialization
{
lookup = Lookups.fixed(qName, DISPLAYABLE, ZERO, new it.tidalwave.geo.geocoding.geonamesprovider.EmblemFactory(ID));
}
return lookup;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy