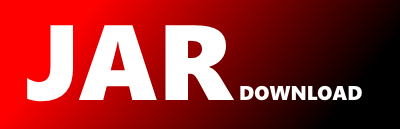
it.tidalwave.geo.geocoding.GeoCoder Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.geocoding;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.ThreadSafe;
import org.openide.util.Lookup;
import it.tidalwave.util.Finder;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.geo.Coordinate;
import it.tidalwave.geo.geocoding.GeoCoderEntity.Type;
/***********************************************************************************************************************
*
* A {@code GeoCoder} provides basic functions for navigate and query a repository of geographic information, laid out
* in a hierarchycal fashion. Typical operations are to retrieve the children of a given node, or the parent of a
* given node, or the near by entities, as well as coordinate-oriented operations (geocoding and reverse geocoding).
* The service is designed as an abstract layer that can be implemented by many providers, both online remote services
* (such as GeoNames or Google or Yahoo!) and local repositories, as well as a mix of the two.
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@ThreadSafe
public interface GeoCoder extends Lookup.Provider
{
public final static Class GeoCoder = GeoCoder.class;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public String getName();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public String getId();
// TODO: introduce a GeoCoderSPI, reduce the public methods on GeoCoder (e.g. most of methods taking an id should
// be methods of GeoCoderEntity.
/*******************************************************************************************************************
*
* Returns the root of the geographical hierarchy (typically the earth).
*
* @return the root of the hierarchy
*
******************************************************************************************************************/
@Nonnull
public GeoCoderEntity getRoot();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public GeoCoderEntity findGeoEntityById (@Nonnull String id)
throws NotFoundException;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Finder findChildren (@Nonnull String id);
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public Finder findNeighbours (@Nonnull String id);
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Finder findSiblings (@Nonnull String id);
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Finder findNearbyPostalCodes (@Nonnull Coordinate coordinate, @Nonnegative float radius);
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Finder findNearestEntity (@Nonnull Coordinate coordinate);
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Finder findEntities (@Nonnull Coordinate coordinate, @Nonnegative float radius);
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public String findCountryCode (@Nonnull Coordinate coordinate);
//FIXME: drop it - use findGeoSubDivision() and then look up country code
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
// @Nonnull
// public GeoCoderEntity findSubdivision (@Nonnull Coordinate coordinates);
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Type typeFromString (@Nonnull String typeAsString);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy