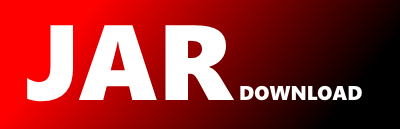
it.tidalwave.geo.geocoding.GeoCoderEntity Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.geocoding;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.ThreadSafe;
import java.util.Collections;
import java.util.Map;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.TimeZone;
import java.util.TreeMap;
import java.io.Serializable;
import java.awt.Image;
import org.openide.util.Lookup;
import it.tidalwave.util.As;
import it.tidalwave.role.Displayable;
import it.tidalwave.util.Finder;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.geo.Coordinate;
import it.tidalwave.geo.Sector;
import it.tidalwave.util.AsException;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@ThreadSafe
public interface GeoCoderEntity extends Comparable, Serializable, As, Lookup.Provider
{
public static final Class GeoCoderEntity = GeoCoderEntity.class;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public enum Type
{
PLANET,
CONTINENT,
COUNTRY,
ADMIN_DIVISION_1,
ADMIN_DIVISION_2,
ADMIN_DIVISION_3,
ADMIN_DIVISION_4,
INHABITED_PLACE,
OTHER,
UNKNOWN
};
/*******************************************************************************************************************
*
* This class provides the capability of recovering the emblem of a {@link GeoCoderEntity}. An emblem is the
* national flag for countries or a blason for local entities. Usually the underlying provider is
* capable of returning a default icon, if possible.
*
* The {@code EmblemFactory} can be used as follows:
*
*
* import static it.tidalwave.geo.geocoding.GeoCoderEntity.EmblemFactory;
*
* GeoCoderEntity entity = ...
* Image emblem = entity.as(EmblemFactory).findEmblem(32); // or entity.getLookup().lookup(EmblemFactory.class) ...
*
*
* Note that it's guaranteed that a non-null {@code}EmblemFactory} is always returned from the lookup.
*
******************************************************************************************************************/
public static interface EmblemFactory extends Serializable
{
public static final Class EmblemFactory = EmblemFactory.class;
/***************************************************************************************************************
*
* Finds the emblem of the current entity. It is possible to specify the minimum size of the required emblem and
* this class will make its best to find one of the proper size; but it's not guaranteed. Note that a larger
* emblem than requested can be returned.
*
* Note that this operation could download resources on demand and so it might take some time to complete.
*
* @param minimumSize the needed size
* @return the emblem
*
**************************************************************************************************************/
@Nonnull
public Image findEmblem (@Nonnegative int minimumSize)
throws NotFoundException;
}
/*******************************************************************************************************************
*
* This class is a generic container of information associated to each {@link GeoCoderEntity}, such as the
* population count or the time zone. It's guaranteed that any {@code GeoCoderEntity} has got its own
* {@link FactSheet}, but no guarantees are made about its contents; some geocoding services provide more, other
* less, other could even provide nothing.
*
* Typical usage is:
*
*
* import it.tidalwave.geo.geocoding.GeoCoderEntity;
* import it.tidalwave.geo.geocoding.GeoCoderEntity.FactSheet;
* import static it.tidalwave.geo.geocoding.GeoCoderEntity.FactSheet.*;
*
* GeoCoderEntity auxerre = ...;
* FactSheet factSheet = auxerre.as(FactSheet); // or auxerre.getLookup().lookup(FactSheet.class);
* long population = factSheet.get(POPULATION);
* TimeZone timeZone = factSheet.get(TIMEZONE);
*
*
******************************************************************************************************************/
@Immutable
public final static class FactSheet implements Serializable
{
private static final long serialVersionUID = 435465463456473L;
public static final Class FactSheet = FactSheet.class;
@Immutable
public final static class Key implements Serializable, Comparable>
{
private static final long serialVersionUID = 565475490834534L;
@Nonnull
private final String name;
protected Key (final @Nonnull String name)
{
this.name = name;
}
@Override
public int compareTo (final @Nonnull Key other)
{
return this.name.compareTo(other.name);
}
@Override
public boolean equals (final @CheckForNull Object object)
{
if (object == null)
{
return false;
}
if (getClass() != object.getClass())
{
return false;
}
final Key other = (Key)object;
return this.name.equals(other.name);
}
@Override
public int hashCode()
{
return this.name.hashCode();
}
@Override @Nonnull
public String toString()
{
return name;
}
}
public final static Key POPULATION = new Key("population");
public final static Key ELEVATION = new Key("elevation");
public final static Key TIMEZONE = new Key("timezone");
public final static Key BOUNDS = new Key("bounds");
private final SortedMap, Object> itemMap = new TreeMap, Object>();
public FactSheet (final @Nonnull Map, Object> itemMap)
{
this.itemMap.putAll(itemMap);
}
/***************************************************************************************************************
*
* Returns a piece of information from this {@code FactSheet}.
*
* @param key the key
* @return the value
* @throws NotFoundException if that piece of information is not present
*
**************************************************************************************************************/
@Nonnull
public T get (final @Nonnull Key key)
throws NotFoundException
{
return NotFoundException.throwWhenNull((T)itemMap.get(key), "Not found: " + key);
}
/***************************************************************************************************************
*
* Returns whether a piece of information is present in this {@code FactSheet}.
*
* @param key the key
* @return {@code true} if that piece of information is present
*
**************************************************************************************************************/
public boolean contains (final @Nonnull Key> item)
{
return itemMap.containsKey(item);
}
/***************************************************************************************************************
*
* Returns which pieces of information are present in this {@code FactSheet}.
*
* @return the keys of the available pieces of information
*
**************************************************************************************************************/
@Nonnull
public SortedSet> getKeys()
{
return Collections.unmodifiableSortedSet((SortedSet>)itemMap.keySet());
}
@Override @Nonnull
public String toString()
{
return String.format("FactSheet@%x[%s]", System.identityHashCode(this), itemMap);
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public GeoCoder getGeoCoder();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public String getId();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Coordinate getCoordinate();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public String getCode();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public String getTypeAsString();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Type getType();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Finder findChildren();
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public GeoCoderEntity getParent()
throws NotFoundException;
/*******************************************************************************************************************
*
* FIXME: this should not be public
*
******************************************************************************************************************/
public boolean isParentSet();
// These methods are replicated here to provide specific javadocs
/*******************************************************************************************************************
*
* Returns a different personality of this object.
*
* Some supported personalities are:
*
*
* - {@link Displayable} which provides the display name of this object
* - {@link EmblemFactory} which provides an emblem for this object
* - {@link FactSheet} which provides an fact sheet for this object
*
*
* For instance:
*
*
* import static it.tidalwave.netbeans.util.Displayable.Displayable;
* import static it.tidalwave.geo.geocoding.GeoCoderEntity.EmblemFactory;
* import static it.tidalwave.geo.geocoding.GeoCoderEntity.FactSheet.*;
*
* GeoCoderEntity geoCoderEntity = ...;
* String displayName = geoCoderEntity.as(Displayable).getDisplayName(Locale.FRENCH);
* Image emblem = geoCoderEntity.as(EmblemFactory).findEmblem(100);
* long population = geoCoderEntity.as(FactSheet).get(POPULATION);
*
*
* @param personalityType the personality type
* @return the personality
* @throws AsException if the personality does not exist
*
******************************************************************************************************************/
@Override @Nonnull
public T as (@Nonnull Class personalityType);
/*******************************************************************************************************************
*
* Returns a different personality of this object.
*
* Some supported personalities are:
*
*
* - {@link Displayable} which provides the display name of this object
* - {@link EmblemFactory} which provides an emblem for this object
* - {@link FactSheet} which provides an fact sheet for this object
*
*
* For instance:
*
*
* import static it.tidalwave.netbeans.util.Displayable.Displayable;
* import static it.tidalwave.geo.geocoding.GeoCoderEntity.EmblemFactory;
* import static it.tidalwave.geo.geocoding.GeoCoderEntity.FactSheet.*;
*
* GeoCoderEntity geoCoderEntity = ...;
* String displayName = geoCoderEntity.as(Displayable).getDisplayName(Locale.FRENCH);
* Image emblem = geoCoderEntity.as(EmblemFactory).findEmblem(100);
* long population = geoCoderEntity.as(FactSheet).get(POPULATION);
*
*
* @param personalityType the personality type
* @param notFoundBehaviour what to do when the personality is not found
* @return the personality
*
******************************************************************************************************************/
@Override @Nonnull
public T as (@Nonnull Class personalityType, @Nonnull NotFoundBehaviour notFoundBehaviour);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy