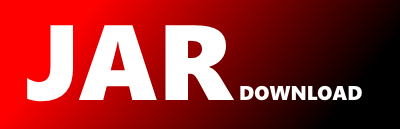
it.tidalwave.geo.geocoding.impl.DefaultGeoCoderEntity Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.geocoding.impl;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import javax.xml.namespace.QName;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.List;
import java.io.Serializable;
import org.openide.util.Lookup;
import org.openide.util.Parameters;
import org.openide.util.lookup.Lookups;
import it.tidalwave.util.As;
import it.tidalwave.util.As.NotFoundBehaviour;
import it.tidalwave.util.Finder;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.role.spi.DefaultDisplayable;
import it.tidalwave.netbeans.util.Locator;
import it.tidalwave.netbeans.capabilitiesprovider.LookupFactory;
import it.tidalwave.geo.Coordinate;
import it.tidalwave.geo.geocoding.GeoCoder;
import it.tidalwave.geo.geocoding.GeoCoderEntity;
import it.tidalwave.geo.geocoding.GeoCoderManager;
import static it.tidalwave.role.Displayable.Displayable;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public class DefaultGeoCoderEntity implements GeoCoderEntity
{
private static final long serialVersionUID = 906839684954745845L;
@CheckForNull @edu.umd.cs.findbugs.annotations.SuppressWarnings("SE_TRANSIENT_FIELD_NOT_RESTORED")
protected transient GeoCoder geoCoder;
private final String geoCoderName;
private Finder children;
private boolean childrenLoaded = false;
@CheckForNull
/* package */ GeoCoderEntity parent;
@Nonnull
private final String id;
@Nonnull
private final String code;
@Nonnull
private final String typeAsString;
@Nonnull
private final Coordinate coordinate;
private boolean parentSearched = false;
@CheckForNull @edu.umd.cs.findbugs.annotations.SuppressWarnings("SE_TRANSIENT_FIELD_NOT_RESTORED")
/* package */ transient Lookup lookup;
private final List capabilities = new ArrayList();
private final DefaultDisplayable displayable;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public DefaultGeoCoderEntity (final @Nonnull GeoCoder geoCoder,
final @CheckForNull GeoCoderEntity parent,
final @Nonnull String id,
final @Nonnull String displayName,
final @Nonnull Coordinate coordinate,
final @Nonnull String code,
final @Nonnull String typeAsString,
final @Nonnull Serializable[] capabilities)
{
Parameters.notNull("id", id);
this.geoCoder = geoCoder;
this.parent = parent;
this.id = id;
this.displayable = new DefaultDisplayable(displayName, "DefaultGeoCoderEntity");
this.coordinate = coordinate;
this.code = code;
this.typeAsString = typeAsString;
this.geoCoderName = geoCoder.getName();
this.capabilities.addAll(Arrays.asList(capabilities));
this.capabilities.addAll(Arrays.asList(coordinate, displayable, new QName(geoCoder.getId() + id + "/")));
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public synchronized GeoCoder getGeoCoder()
{
if (geoCoder == null)
{
try
{
geoCoder = Locator.find(GeoCoderManager.class).findGeoCoderByName(geoCoderName);
}
catch (NotFoundException e)
{
throw new IllegalStateException(e);
}
}
return geoCoder;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String getId()
{
return id;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public Coordinate getCoordinate()
{
return coordinate;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String getCode()
{
return code;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String getTypeAsString()
{
return typeAsString;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public Type getType()
{
return getGeoCoder().typeFromString(typeAsString);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public synchronized Finder findChildren()
{
if (!childrenLoaded)
{
children = getGeoCoder().findChildren(id);
childrenLoaded = true;
}
return children;
}
/*******************************************************************************************************************
*
* FIXME: this setter is the only not immutable part of this class. Drop it.
* I think it's only needed for lazy exploring of parents, but we could move
* the logic to getParent().
*
******************************************************************************************************************/
public void setParent (final @Nonnull GeoCoderEntity parent)
{
this.parent = parent;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public GeoCoderEntity getParent()
throws NotFoundException
{
if ((parent == null) && !parentSearched)
{
getGeoCoder().findGeoEntityById(id); // this will set my parent as a side-effect
parentSearched = true; // needed since e.g. Europe has a null parent
}
return NotFoundException.throwWhenNull(parent, "No parent for " + this);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public boolean isParentSet()
{
return parentSearched;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String toString()
{
return String.format("DefaultGeoCoderEntity@%x[%s, %s]",
System.identityHashCode(this), id, displayable.getDisplayName());
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public boolean equals (final @CheckForNull Object object)
{
if (object == null)
{
return false;
}
if (getClass() != object.getClass())
{
return false;
}
final DefaultGeoCoderEntity other = (DefaultGeoCoderEntity)object;
return this.id.equals(other.id);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public int hashCode()
{
return id.hashCode();
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public int compareTo (final @Nonnull GeoCoderEntity geoCoderEntity)
{
return displayable.getDisplayName().compareTo(geoCoderEntity.as(Displayable).getDisplayName());
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public T as (final @Nonnull Class clazz)
{
return as(clazz, As.Defaults.throwAsException(clazz));
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public T as (final @Nonnull Class clazz,
final @Nonnull NotFoundBehaviour notFoundBehaviour)
{
final T as = getLookup().lookup(clazz);
if (as != null)
{
return as;
}
else
{
return notFoundBehaviour.run(null);
}
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public synchronized Lookup getLookup()
{
if (lookup == null) // also after serialization
{
lookup = LookupFactory.createLookup(this, Lookups.fixed(capabilities.toArray()));
}
return lookup;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy