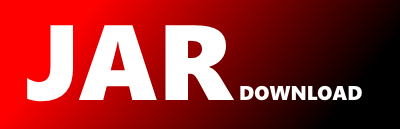
it.tidalwave.geo.location.elmo.impl.Support Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.location.elmo.impl;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.util.Date;
import javax.xml.datatype.XMLGregorianCalendar;
import org.openide.util.Lookup;
import org.openide.util.Parameters;
import org.openrdf.elmo.Entity;
import org.openrdf.elmo.sesame.SesameManager;
import org.openrdf.model.Literal;
import it.tidalwave.util.As;
import it.tidalwave.role.Displayable;
import it.tidalwave.role.MutableDisplayable;
import it.tidalwave.semantic.OwlThing;
import it.tidalwave.semantic.SkosConcept;
import it.tidalwave.semantic.Wrapper;
import it.tidalwave.openrdf.elmo.ElmoAsSupport;
import it.tidalwave.openrdf.elmo.ElmoManagerThreadLocal;
import it.tidalwave.openrdf.elmo.ElmoOwlThing;
import it.tidalwave.openrdf.elmo.ElmoSkosConcept;
import it.tidalwave.openrdf.elmo.ElmoUtils;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public class Support implements As
{
@CheckForNull
private Lookup lookup;
protected final static Class Entity = Entity.class;
private final SkosConcept skosConcept = new ElmoSkosConcept(this);
private final OwlThing owlThing = new ElmoOwlThing(this);
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
@Nonnull
public T as (@Nonnull final Class clazz)
{
return as(clazz, As.Defaults.throwAsException(clazz));
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
@Nonnull
public T as (@Nonnull final Class clazz, @Nonnull final As.NotFoundBehaviour notFoundBehaviour)
{
// final T as = getLookup().lookup(clazz);
// FIXME: should be injected by the factory
if (clazz.equals(SkosConcept.class) || clazz.equals(MutableDisplayable.class) || clazz.equals(Displayable.class))
{
return (T)skosConcept;
}
if (clazz.equals(OwlThing.class))
{
return (T)owlThing;
}
// END FIXME
return ElmoAsSupport.as(this, clazz, notFoundBehaviour);
// return (as != null) ? as : ElmoAsSupport.as(this, clazz, notFoundBehaviour);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Nonnull
public synchronized Lookup getLookup()
{
if (lookup == null)
{
lookup = ElmoUtils.createLookup(this, this, skosConcept, owlThing);
}
return lookup;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
protected static XMLGregorianCalendar toXMLGregorianCalendar (final @Nonnull Date date)
{
final Literal literal = ((SesameManager)(ElmoManagerThreadLocal.get())).getLiteralManager().getLiteral(date);
assert literal != null : "null literal";
return literal.calendarValue();
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
protected static void merge (final @Nonnull As object) // FIXME: move to an injected capability - Persistence?
{
Parameters.notNull("object", object);
final Entity entity = Wrapper.unwrap(object.as(Entity.class));
ElmoManagerThreadLocal.get().merge(entity);
}
/*******************************************************************************************************************
*
* Useful for self-document purportedly empty catch blocks (and avoid a FindBugs warning).
*
******************************************************************************************************************/
protected static void doNothing()
{
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy