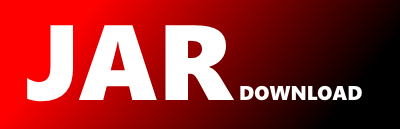
it.tidalwave.geo.viewer.spi.openstreetmap.OpenStreetMapTileProviderDescriptor Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.viewer.spi.openstreetmap;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import java.util.Arrays;
import java.util.Collections;
import java.util.Locale;
import java.util.Map;
import java.util.SortedSet;
import java.util.TreeSet;
import java.awt.Dimension;
import java.net.MalformedURLException;
import java.net.URL;
import org.openide.util.lookup.ServiceProvider;
import it.tidalwave.role.Displayable;
import it.tidalwave.geo.viewer.spi.TileProviderDescriptor;
import it.tidalwave.role.LocalizedDisplayable;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@ServiceProvider(service=TileProviderDescriptor.class)
public class OpenStreetMapTileProviderDescriptor extends TileProviderDescriptor
{
private final static int MIN_ZOOM_LEVEL = 3;
private final static int MAX_ZOOM_LEVEL = 17;
private final static int TOP_ZOOM_LEVEL = 17;
private final static int INITIAL_ZOOM_LEVEL = 13;
private final static int TILE_SIZE = 256;
private final static LocalizedDisplayable DISPLAYABLE = new LocalizedDisplayable()
{
@Override @Nonnull
public String getDisplayName()
{
return getDisplayName(Locale.ENGLISH);
}
@Override @Nonnull
public String getDisplayName (final @Nonnull Locale locale)
{
return "OpenStreetMap";
}
@Override @Nonnull
public Map getDisplayNames()
{
return Collections.singletonMap(Locale.ENGLISH, getDisplayName());
}
@Override @Nonnull
public SortedSet getLocales()
{
return new TreeSet(Arrays.asList(Locale.ENGLISH));
}
};
@Override @Nonnull
public Dimension getTileSize()
{
return new Dimension(TILE_SIZE, TILE_SIZE);
}
@Override @Nonnegative
public int getLevelCount()
{
return 16;
}
@Override @Nonnegative
public int getMinZoomLevel()
{
return MIN_ZOOM_LEVEL;
}
@Override @Nonnegative
public int getMaxZoomLevel()
{
return MAX_ZOOM_LEVEL;
}
@Override @Nonnegative
public int getTopZoomLevel()
{
return TOP_ZOOM_LEVEL;
}
@Override @Nonnegative
public int getInitialZoomLevel()
{
return INITIAL_ZOOM_LEVEL;
}
@Override @Nonnull
public HorizontalLayout getHorizontalLayout()
{
return HorizontalLayout.LEFT_TO_RIGHT;
}
@Override @Nonnull
public VerticalLayout getVerticalLayout()
{
return VerticalLayout.BOTTOM_TO_TOP;
}
@Override @Nonnull
public String getDataCacheName()
{
return "OSM";
}
@Override @Nonnull
public URL getTileUrl (final @Nonnegative int level, final int column, final int row)
throws MalformedURLException
{
return new URL(String.format("http://tile.openstreetmap.org/%d/%d/%d.png", level, column, row));
}
@Override @Nonnull
protected Displayable getDisplayable()
{
return DISPLAYABLE;
}
// registerZoomLevel(ZoomLevel.STREET, 9);
// registerZoomLevel(ZoomLevel.TOWN, 9);
// registerZoomLevel(ZoomLevel.PROVINCE, 9);
// registerZoomLevel(ZoomLevel.REGION, 10);
// registerZoomLevel(ZoomLevel.COUNTRY, 12);
// registerZoomLevel(ZoomLevel.CONTINENT, 13);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy