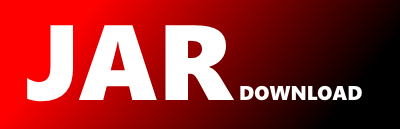
it.tidalwave.geo.viewer.GeoViewManager Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.viewer;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.Collection;
import java.awt.Point;
import it.tidalwave.geo.Coordinate;
import it.tidalwave.geo.Sector;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public interface GeoViewManager
{
/*******************************************************************************************************************
*
* Adjusts the geographic view view so all the specified {@link Coordinate}s are visible.
*
* @param coordinates the collection of {@code Coordinate}s
* @param defaultZoomLevel the default zoom level to use if only a single {@link Coordinate} is specified
* @throws IllegalArgumentException if the collection is empty
*
******************************************************************************************************************/
public void fitView (@Nonnull Collection coordinates, @Nonnull ZoomLevel defaultZoomLevel);
/*******************************************************************************************************************
*
* Adjusts the geographic view view so all the specified {@link Sector}s is entirely visible.
*
* @param sector the {@code Sector}
* @param defaultZoomLevel the default zoom level to use if the {@code Sector} collapses to a single
* {@code Coordinate}
*
******************************************************************************************************************/
public void fitView (@Nonnull Sector sector, @Nonnull ZoomLevel defaultZoomLevel);
/*******************************************************************************************************************
*
* Centers the geographic view on the given {@link Coordinate} and sets the specified {@link ZoomLevel}. The zoom
* level can be {@code null}, in this case the previous value is preserved.
*
* @param coordinates the center position
* @param zoomLevel the zoom level (can be null)
*
******************************************************************************************************************/
public void centerOn (@Nonnull Coordinate coordinates, @Nullable ZoomLevel zoomLevel);
/*******************************************************************************************************************
*
* Moves the center point of the geographic view by the specified amount.
*
* @param longitudeDelta the pan amount in the longitude axis
* @param latitudeDelta the pan amount in the latitude axis
*
******************************************************************************************************************/
public void pan (double longitudeDelta, double latitudeDelta);
/*******************************************************************************************************************
*
* Set the zoom level.
*
* @return zoom the zoom level
*
******************************************************************************************************************/
public void setZoom (int zoom); // FIXME: redo using ZoomLevel in place of the int
/*******************************************************************************************************************
*
* Returns the current zoom level.
*
* @return the zoom level
*
**************************************************************************/
public int getZoom(); // FIXME: redo using ZoomLevel in place of the int
/*******************************************************************************************************************
*
* Takes a {@link Coordinate} and returns the {@link Point} where they are rendered (referring to the origin of the
* renderer component).
*
* @param coordinate the {@code Coordinate}
* @return the {@code Point}
*
******************************************************************************************************************/
@Nonnull
public Point coordinateToPoint (@Nonnull Coordinate coordinate);
/*******************************************************************************************************************
*
* Takes a {@link Point} referring to the origin of the renderer component and returns the {@link Coordinate} that
* are rendered at its position.
*
* @param point the {@code Point}
* @return the {@code Coordinate}
*
******************************************************************************************************************/
@Nonnull
public Coordinate pointToCoordinate (@Nonnull Point location);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy