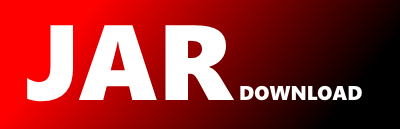
it.tidalwave.geo.viewer.impl.spi.DefaultResourceCache Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.viewer.impl.spi;
import javax.annotation.Nonnull;
import javax.annotation.PostConstruct;
import javax.inject.Inject;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.awt.image.BufferedImage;
import javax.imageio.ImageIO;
import java.net.URI;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.IOUtils;
import org.openide.util.Parameters;
import it.tidalwave.util.logging.Logger;
import it.tidalwave.geo.viewer.role.CacheLocationProvider;
import it.tidalwave.geo.viewer.spi.ResourceCache;
import it.tidalwave.util.NotFoundException;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public class DefaultResourceCache implements ResourceCache // extends TileCache
{
private static final String CLASS = DefaultResourceCache.class.getName();
private static final Logger logger = Logger.getLogger(CLASS);
private File cacheFolder;
@Inject
private CacheLocationProvider cacheSettings;
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public BufferedImage findImage (final URI uri)
throws IOException, NotFoundException
{
logger.fine("get(%s)", uri);
File file = getFile(uri);
if (file.exists() && file.canRead())
{
try
{
logger.finest(">>>> locally cached at %s", file);
return ImageIO.read(file);
}
catch (IOException e)
{
logger.throwing(CLASS, "put()", e);
}
}
throw new NotFoundException(uri.toString());
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public synchronized void storeImage (final @Nonnull URI uri, final @Nonnull byte[] buffer)
{
Parameters.notNull("uri", uri);
Parameters.notNull("buffer", buffer);
logger.fine("storeImage(%s, %d)", uri, buffer.length);
FileOutputStream fos = null;
try
{
final File file = getFile(uri);
logger.finest(">>>> locally caching at %s", file);
fos = new FileOutputStream(file);
fos.write(buffer);
}
catch (IOException e)
{
logger.throwing(CLASS, "storeImage()", e);
}
finally
{
IOUtils.closeQuietly(fos);
}
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public URI findCachedResourceUri (final @Nonnull URI uri)
throws NotFoundException
{
logger.fine("findCachedResourceUri(%s)", uri);
try
{
final File file = getFile(uri);
logger.finer(">>>> cached file %s does %sexist", file, file.exists() ? "" : "not ");
if (!file.exists())
{
throw new NotFoundException(uri.toString());
}
final URI url = file.toURI();
logger.finer(">>>> returning %s", url);
return url;
}
catch (IOException e)
{
throw new NotFoundException(uri.toString() + " - " + e);
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private File getFile (final URI uri)
throws IOException
{
String prefix = "";
// FIXME - use regex matchers and make them registrable
if (uri.toString().startsWith("http://tile.openstreetmap.org"))
{
prefix = "OSM/";
}
else if (uri.toString().contains("ortho.tiles.virtualearth.net/tiles/a"))
{
prefix = "MVE/a/";
}
else if (uri.toString().contains("ortho.tiles.virtualearth.net/tiles/h"))
{
prefix = "MVE/h/";
}
else if (uri.toString().contains("ortho.tiles.virtualearth.net/tiles/r"))
{
prefix = "MVE/r/";
}
// END FIXME
final String fileName = NameMangler.mangle(uri.toString());
final File file = new File(cacheFolder, prefix + fileName);
FileUtils.forceMkdir(file.getParentFile());
return file;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@PostConstruct
@SuppressWarnings("PMD.UnusedPrivateMethod") @edu.umd.cs.findbugs.annotations.SuppressWarnings("UPM_UNCALLED_PRIVATE_METHOD")
private void initialize()
{
this.cacheFolder = cacheSettings.getCacheFolder();
logger.info(">>>> cacheFolder: " + cacheFolder);
try
{
FileUtils.forceMkdir(cacheFolder);
}
catch (IOException e)
{
logger.severe("Cannot create cache folder: %s", cacheFolder);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy