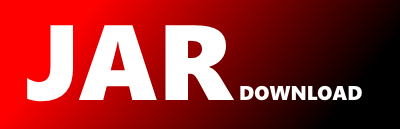
it.tidalwave.geo.viewer.spi.GeoViewProviderSupport Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo.viewer.spi;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import javax.inject.Inject;
import java.util.HashMap;
import java.util.Map;
import javax.swing.JPopupMenu;
import org.openide.util.Lookup;
import it.tidalwave.util.logging.Logger;
import it.tidalwave.geo.viewer.ZoomLevel;
/***********************************************************************************************************************
*
* A support class useful as a base for concrete {@link GeoViewProvider} implementations.
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
public abstract class GeoViewProviderSupport implements GeoViewProvider, GeoViewProvider.LifeCycle, Lookup.Provider
{
private static final String CLASS = GeoViewProviderSupport.class.getName();
private static final Logger logger = Logger.getLogger(CLASS);
protected boolean ready;
/** The mapping from abstract to concrete zoom level. */
private final Map zoomLevelMap = new HashMap();
@Nonnull
private final String name;
private final int initialZoomLevel;
protected CoordinateTracker coordinatesTracker;
@Inject
private Lookup lookup;
protected GeoDataProviderDescriptor geoDataProviderDescriptor;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
protected GeoViewProviderSupport (final @Nonnull String name,
final int initialZoomLevel)
{
this.name = name;
this.initialZoomLevel = initialZoomLevel;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public Lookup getLookup()
{
return lookup;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void initResources()
{
logger.info("initResources() - this provider is now ready");
ready = true;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public /*final becauxe of mockito */ boolean isReady()
{
return ready;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void setCoordinatesTracker (final @Nonnull CoordinateTracker coordinatesTracker)
{
this.coordinatesTracker = coordinatesTracker;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public int getZoomLevel (final @Nonnull ZoomLevel zoomLevel)
{
final Integer i = zoomLevelMap.get(zoomLevel);
return (i != null) ? i : getInitialZoomLevel();
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public final String getName()
{
return name;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public final int getInitialZoomLevel()
{
return initialZoomLevel;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @CheckForNull
public JPopupMenu getOptionsMenu()
{
return null;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void activating()
{
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void activated()
{
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void deactivated()
{
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void deactivating()
{
}
/*******************************************************************************************************************
*
* Registers a mapping from an abstract zoom level to a concrete zoom level.
*
* @param zoomLevel the abstract zoom level
* @param level the concrete zoom level
*
******************************************************************************************************************/
protected void registerZoomLevel (final @Nonnull ZoomLevel zoomLevel, final int level)
{
zoomLevelMap.put(zoomLevel, level);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public void setGeoDataProviderDescriptor (final @Nonnull GeoDataProviderDescriptor geoDataProviderDescriptor)
{
this.geoDataProviderDescriptor = geoDataProviderDescriptor;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public GeoDataProviderDescriptor getGeoDataProviderDescriptor()
{
return geoDataProviderDescriptor;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy