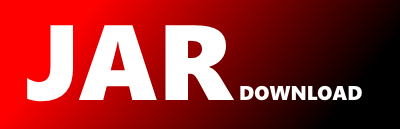
it.tidalwave.geo.CoordinateSet Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.Immutable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.io.Serializable;
import org.openide.util.Lookup;
import it.tidalwave.util.As;
import it.tidalwave.geo.impl.AsSupport;
/***********************************************************************************************************************
*
* This class models an immutable set of unordered {@link Coordinate}s.
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@Immutable
public final class CoordinateSet extends AsSupport implements Serializable, Lookup.Provider, As, Iterable
{
private static final long serialVersionUID = 58902340982359L;
private final Collection coordinates;
private final Sector sector;
/*******************************************************************************************************************
*
* Creates an empty set of coordinates.
*
******************************************************************************************************************/
public CoordinateSet()
{
coordinates = Collections.emptyList();
sector = new Sector();
}
/*******************************************************************************************************************
*
* Creates a set of coordinates with the given contents.
*
******************************************************************************************************************/
public CoordinateSet (@Nonnull final Coordinate ... coordinates)
{
this(Arrays.asList(coordinates));
}
/*******************************************************************************************************************
*
* Creates a set of coordinates with the given contents.
*
******************************************************************************************************************/
public CoordinateSet (@Nonnull final Collection coordinates)
{
this.coordinates = Collections.unmodifiableCollection(coordinates);
sector = new Sector(coordinates);
}
/*******************************************************************************************************************
*
* Creates a new set of coordinates adding the given coordinate.
*
* @param coordinate the coordinate to add
* @return a new set
*
******************************************************************************************************************/
@Nonnull
public CoordinateSet with (@Nonnull final Coordinate coordinate)
{
final List all = new ArrayList(this.coordinates);
all.add(coordinate);
return new CoordinateSet(all);
}
/*******************************************************************************************************************
*
* Creates a new set of coordinates adding the given coordinates.
*
* @param coordinate the coordinate to add
* @return a new set
*
******************************************************************************************************************/
@Nonnull
public CoordinateSet with (@Nonnull final Collection coordinates)
{
final List all = new ArrayList(this.coordinates);
all.addAll(coordinates);
return new CoordinateSet(all);
}
/*******************************************************************************************************************
*
* Creates a new set of coordinates adding the given coordinates.
*
* @param coordinate the coordinate to add
* @return a new set
*
******************************************************************************************************************/
@Nonnull
public CoordinateSet with (@Nonnull final Coordinate ... coordinates)
{
return with(Arrays.asList(coordinates));
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
@Nonnull
public Iterator iterator()
{
return Collections.unmodifiableCollection(coordinates).iterator();
}
/*******************************************************************************************************************
*
* Returns the size of the set.
*
* @return the size of the set
*
******************************************************************************************************************/
@Nonnegative
public int getSize()
{
return coordinates.size();
}
/*******************************************************************************************************************
*
* Returns the smaller {@link Sector} containing all the coordinates.
*
* @return the {@code Sector}
*
******************************************************************************************************************/
@Nonnull
public Sector getSector()
{
return sector;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public boolean equals (@CheckForNull final Object object)
{
if (object == null)
{
return false;
}
if (getClass() != object.getClass())
{
return false;
}
final CoordinateSet other = (CoordinateSet)object;
return this.coordinates.equals(other.coordinates) && this.sector.equals(other.sector);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public int hashCode()
{
int hash = 5;
hash = 97 * hash + coordinates.hashCode();
hash = 97 * hash + sector.hashCode();
return hash;
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
@Nonnull
public String toString()
{
return sector.isVoid() ? "CoordinateSet[empty]"
: String.format("CoordinateSet[%s / %s]", coordinates, sector);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy