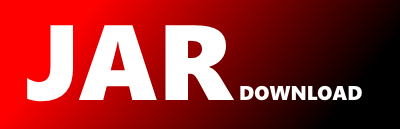
it.tidalwave.geo.Range Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* forceTen - open source geography
* Copyright (C) 2007-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://forceten.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/forceten-src
*
**********************************************************************************************************************/
package it.tidalwave.geo;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.Immutable;
import java.io.Serializable;
import org.openide.util.Lookup;
import it.tidalwave.util.As;
import it.tidalwave.util.AsException;
import it.tidalwave.geo.impl.AsSupport;
import it.tidalwave.geo.impl.WGS84;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@Immutable
public class Range extends AsSupport implements Serializable, Lookup.Provider, As
{
private static final long serialVersionUID = 70483759982359L;
@Nonnull
private final Coordinate coordinate;
@Nonnegative
private final double radius;
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private Range (final @Nonnull Coordinate coordinate, final @Nonnegative double radius)
{
this.coordinate = coordinate;
this.radius = radius;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public static Range on (final double latitude, final double longitude)
{
return Range.on(new Coordinate(latitude, longitude));
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public static Range on (final @Nonnull Coordinate coordinate)
{
return new Range(coordinate, 0);
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public static Range on (final @Nonnull Lookup.Provider lookupProvider)
{
final Coordinate coordinate = lookupProvider.getLookup().lookup(Coordinate.class);
if (coordinate == null)
{
throw new IllegalArgumentException("" + lookupProvider);
}
return new Range(coordinate, 0);
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public static Range on (final @Nonnull As as)
{
try
{
return new Range(as.as(Coordinate.class), 0);
}
catch (AsException e)
{
throw new IllegalArgumentException("" + as);
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Range withRadius (final @Nonnegative double radius)
{
return new Range(coordinate, radius);
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnull
public Coordinate getCoordinate()
{
return coordinate;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
@Nonnegative
public double getRadius()
{
return radius;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public boolean contains (final @Nonnull Coordinate coordinate)
{
return WGS84.distance(this.coordinate, coordinate) <= radius;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public boolean contains (final @Nonnull Iterable coordinates)
{
for (final Coordinate coordinate : coordinates)
{
if (!contains(coordinate))
{
return false;
}
}
return true;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public boolean contains (final @Nonnull Range range)
{
return WGS84.distance(coordinate, range.coordinate) <= radius - range.radius;
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
public boolean contains (final @Nonnull Sector sector)
{
return contains(sector.getNECorner()) && contains(sector.getNWCorner()) &&
contains(sector.getSECorner()) && contains(sector.getSWCorner());
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public boolean equals (@CheckForNull final Object object)
{
if (object == null)
{
return false;
}
if (getClass() != object.getClass())
{
return false;
}
final Range other = (Range)object;
return (Math.abs(this.radius - other.radius) < Coordinate.EPSILON) &&
this.coordinate.equals(other.coordinate);
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override
public int hashCode()
{
return 7 + 79 * ((int)Double.doubleToLongBits(radius) + 79 * coordinate.hashCode());
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public String toString()
{
return String.format("Range[%s %s, %f km]",
CoordinateFormat.formatLatitude(coordinate.getLatitude()),
CoordinateFormat.formatLatitude(coordinate.getLongitude()),
radius / 1000.0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy