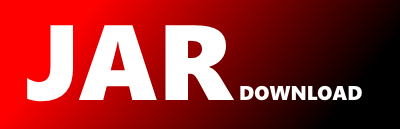
it.tidalwave.metadata.persistence.MetadataComposite Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.persistence;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.util.List;
import it.tidalwave.metadata.persistence.spi.MetadataPersistenceSpi;
/*******************************************************************************
*
* This class provides basic support for {@link MetadataProperty} and
* @link MetadataPropertySet}. Should not be used directly.
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public class MetadataComposite
{
public static final String CHILD_PROPERTIES_PROPERTY = "childProperties";
@Nonnull
protected static final MetadataPersistenceSpi persistenceSpi = MetadataPersistenceSpi.Locator.findPersistenceSpi();
@Nonnull
protected final Class> itemClass;
@CheckForNull
protected final String propertyName;
@CheckForNull
protected final MetadataComposite parent;
/***************************************************************************
*
**************************************************************************/
protected MetadataComposite (@Nonnull final MetadataComposite parent,
@Nonnull final String propertyName)
{
if (parent == null)
{
throw new IllegalArgumentException("parent is mandatory");
}
if (propertyName == null)
{
throw new IllegalArgumentException("propertyName is mandatory");
}
assert (parent.getItemClass() != null) : "parent with no itemClass";
this.propertyName = propertyName;
this.parent = parent;
this.itemClass = parent.getItemClass();
}
/***************************************************************************
*
**************************************************************************/
protected MetadataComposite (@Nonnull final Class> itemClass)
{
if (itemClass == null)
{
throw new IllegalArgumentException("itemClass is mandatory");
}
this.itemClass = itemClass;
this.propertyName = null;
this.parent = null;
}
/***************************************************************************
*
* Returns the class which contains the property represented by this object.
*
* @return the class
*
**************************************************************************/
@Nonnull
public final Class> getItemClass()
{
return itemClass;
}
/***************************************************************************
*
* Returns the parent of this metadata property.
*
* @return the parent
*
**************************************************************************/
@CheckForNull
public MetadataComposite getParent()
{
return parent;
}
/***************************************************************************
*
* Return all the child properties contained in this property.
*
* @return the child properties
*
**************************************************************************/
@Nonnull
public synchronized List getChildProperties()
{
return persistenceSpi.findProperties(this);
}
/***************************************************************************
*
* {@inheritDoc}
*
**************************************************************************/
@Override
public final boolean equals (@CheckForNull final Object object)
{
if (object == null)
{
return false;
}
if (getClass() != object.getClass())
{
return false;
}
final MetadataComposite other = (MetadataComposite)object;
if (this.propertyName != other.propertyName && (this.propertyName == null || !this.propertyName.equals(other.propertyName)))
{
return false;
}
if (!this.itemClass.equals(other.itemClass))
{
return false;
}
if (this.parent != other.parent && (this.parent == null || !this.parent.equals(other.parent)))
{
return false;
}
return true;
}
/***************************************************************************
*
* {@inheritDoc}
*
**************************************************************************/
@Override
public final int hashCode()
{
int hash = 7;
hash = 97 * hash + (this.propertyName != null ? this.propertyName.hashCode() : 0);
hash = 97 * hash + this.itemClass.hashCode();
hash = 97 * hash + (this.parent != null ? this.parent.hashCode() : 0);
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy