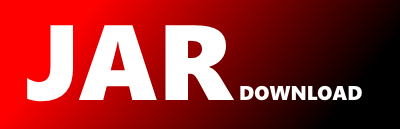
it.tidalwave.metadata.persistence.MetadataPersistence Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.persistence;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.NoSuchElementException;
import java.util.Set;
import org.openide.loaders.DataObject;
import org.openide.util.Lookup;
import it.tidalwave.metadata.MetadataItemHolder;
import it.tidalwave.metadata.Metadata.FindOption;
import it.tidalwave.metadata.Metadata.StoreOption;
import it.tidalwave.metadata.NoSuchMetadataTypeException;
/*******************************************************************************
*
* This service provides persistence facilities for metadata.
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public interface MetadataPersistence
{
/***************************************************************************
*
* An option specifying a set of names for property sets. Only the sets matching
* the given names will be used. ANY_PROPERTY_SET
matches any
* property set.
*
**************************************************************************/
public static class PropertySetFilter implements StoreOption
{
@Nonnull
private final List propertySetNames;
public PropertySetFilter (@Nonnull final String ... propertySetNames)
{
this.propertySetNames = Arrays.asList(propertySetNames);
}
public PropertySetFilter (@Nonnull final Collection propertySetNames)
{
this.propertySetNames = new ArrayList(propertySetNames);
}
@Nonnull
public Set getPropertySetNames()
{
return new HashSet(propertySetNames);
}
@Override
@Nonnull
public String toString()
{
return String.format("PropertySetFilter[%s]", propertySetNames);
}
public static final PropertySetFilter ANY_PROPERTY_SET = new PropertySetFilter()
{
@Override
@Nonnull
public Set getPropertySetNames()
{
final Set result = new HashSet();
for (final MetadataPropertySet propertySet : MetadataPersistence.Locator.findPersistence().getPropertySets())
{
result.add(propertySet.getName());
}
return result;
}
};
}
/***************************************************************************
*
* Make the specified item entering the "managed" state. This means that
* first the item is updated with the contents of the persistence layer, if
* any; later, every change to item will be automatically reflected to the
* persistent layer.
*
* @param dataObject the bound object
* @param item the item to manage
*
**************************************************************************/
public void manage (@Nonnull DataObject dataObject,
@Nonnull MetadataItemHolder item,
@Nonnull StoreOption... options)
throws MetadataPersistenceException, NoSuchMetadataTypeException;
/***************************************************************************
*
* Refresh the given item. The item properties will be updated with the
* values currently present in the persistence facility.
*
* @param dataObject the bound object
* @param item the item to manage
*
**************************************************************************/
public boolean refresh (@Nonnull DataObject dataObject,
@Nonnull MetadataItemHolder item,
@Nonnull FindOption... options)
throws MetadataPersistenceException, NoSuchMetadataTypeException;
/***************************************************************************
*
* Makes the give item persistent. The item properties will be updated
* (and eventually created) in the persistence facility.
*
* @param dataObject the bound object
* @param item the item to manage
*
**************************************************************************/
public void persist (@Nonnull DataObject dataObject,
@Nonnull MetadataItemHolder item,
@Nonnull StoreOption... options)
throws MetadataPersistenceException, NoSuchMetadataTypeException;
/***************************************************************************
*
* Returns a list of all the property sets currently present in the
* persistence facility.
*
* @return the list of property sets
*
**************************************************************************/
@Nonnull
public List getPropertySets();
/***************************************************************************
*
* Finds a {@link MetadataPropertySet} given its bean class.
*
* @param beanClass the bean class
* @return the property set
* @throws NoSuchElementException if nothing is found
*
**************************************************************************/
@Nonnull
public MetadataPropertySet findPropertySetByClass (@Nonnull Class> beanClass)
throws NoSuchElementException;
/***************************************************************************
*
* Removes all the properties bound to the given {@link DataObject} and
* satisfying all the options.
*
* @param dataObject the data object
* @param options the options
*
**************************************************************************/
public void removeProperties (@Nonnull DataObject dataObject,
@Nonnull StoreOption ... options);
/***************************************************************************
*
* The Service Locator for MetadataPersistence
.
*
**************************************************************************/
public static final class Locator
{
private Locator()
{
}
@Nonnull
public static MetadataPersistence findPersistence()
{
final MetadataPersistence persistence = Lookup.getDefault().lookup(MetadataPersistence.class);
if (persistence == null)
{
throw new RuntimeException("MetadataPersistence not found");
}
return persistence;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy