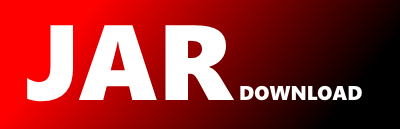
it.tidalwave.metadata.persistence.MetadataProperty Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.persistence;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import it.tidalwave.metadata.persistence.spi.BeanAccessor;
import it.tidalwave.metadata.persistence.spi.UnknownPropertyException;
/*******************************************************************************
*
* This class describes a single property of a metadata item.
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public class MetadataProperty extends MetadataComposite
{
private final Map> cache = new HashMap>();
/***************************************************************************
*
* Creates a new instance given a parent and a name.
*
* @param parent the parent
* @param propertyName the name
*
**************************************************************************/
public MetadataProperty (@Nonnull final MetadataComposite parent,
@Nonnull final String propertyName)
{
super(parent, propertyName);
}
/***************************************************************************
*
* Returns the property name.
*
* @return the name of the property
*
**************************************************************************/
@CheckForNull
public String getPropertyName()
{
return propertyName;
}
/***************************************************************************
*
* Returns the property type.
*
* @return the type of the property
*
**************************************************************************/
@Nonnull
public Class getType()
throws UnknownPropertyException
{
final BeanAccessor> beanAccessor = persistenceSpi.findAccessorByClass(getItemClass());
return (Class)beanAccessor.getDefaultPropertyType(propertyName);
}
/***************************************************************************
*
* Finds or creates a new PropertyAndValue
instance for the given
* value. This method guarantees that always the same instance is returned
* for a given value; this saves time in case the method
* {@link PropertyAndValue#getDataObjectCount()} is called multiple times
* since it won't be recomputed for multiple instances, and furthermore it
* makes it possible for the Persistence Facility to constantly update the
* count when new data is added or removed.
*
* @param value the value
*
**************************************************************************/
@Nonnull
public synchronized PropertyAndValue findOrCreatePropertyAndValue (@CheckForNull final T value)
{
// Use a String for the key so you can handle null and
// don't worry about value's equals() and hashCode()
final String key = (value == null) ? "null" : value.toString();
PropertyAndValue pav = cache.get(key);
if (pav == null)
{
pav = new PropertyAndValue(this, value);
cache.put(key, pav);
}
return pav;
}
/***************************************************************************
*
* Returns the list of all the possible values for this property. This
* method dinamically queries the persistent facility and always produces
* an updated list.
*
* @return the values for this property
*
**************************************************************************/
@Nonnull
public List getValues()
{
return persistenceSpi.findValues(this);
}
/***************************************************************************
*
* {@inheritDoc}
*
**************************************************************************/
@Override
@Nonnull
public final String toString()
{
return String.format("MetadataProperty[%s]", propertyName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy