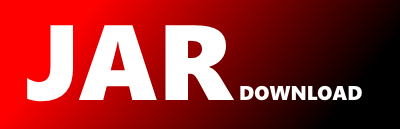
it.tidalwave.metadata.persistence.query.Criterion Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.persistence.query;
import it.tidalwave.metadata.persistence.MetadataComposite;
import it.tidalwave.metadata.persistence.spi.UnknownPropertyException;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import java.util.List;
import org.openide.loaders.DataObject;
import it.tidalwave.metadata.persistence.MetadataProperty;
import it.tidalwave.metadata.persistence.MetadataPropertySet;
import it.tidalwave.metadata.persistence.PropertyAndValue;
import it.tidalwave.metadata.persistence.spi.MetadataPersistenceSpi;
/*****************************s**************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public class Criterion
{
/***************************************************************************
*
*
**************************************************************************/
public static enum Operator
{
AND, OR, NOT, EQUALS, GT, GTE, LT, LTE
}
@CheckForNull
private final Criterion a;
@CheckForNull
private final Criterion b;
@CheckForNull
private final String propertySetName;
@CheckForNull
private final String code;
@Nonnull
private final Operator op;
@CheckForNull
private final Object object;
/***************************************************************************
*
*
**************************************************************************/
private Criterion (@Nonnull final Operator op, @Nonnull final Criterion a)
{
this(a, op, null);
}
/***************************************************************************
*
*
**************************************************************************/
private Criterion (@Nonnull final Criterion a,
@Nonnull final Operator op,
@CheckForNull final Criterion b)
{
this.a = a;
this.b = b;
this.op = op;
this.propertySetName = null;
this.code = null;
this.object = null;
}
/***************************************************************************
*
*
**************************************************************************/
private Criterion (@Nonnull final String propertySetName,
@Nonnull final String code,
@Nonnull final Operator op,
@Nonnull final Object object)
{
this.a = null;
this.b = null;
this.op = op;
this.propertySetName = propertySetName;
this.code = code;
this.object = object;
}
/***************************************************************************
*
* Finds all the {@link DataObject}s that satisfy this criterion.
*
* @return the objects
*
**************************************************************************/
@Nonnull
public List findDataObjects()
throws UnknownPropertyException
{
return MetadataPersistenceSpi.Locator.findPersistenceSpi().findDataObjects(this);
}
/***************************************************************************
*
* Returns the count of all {@link DataObject}s bound to this criterion.
*
* @return the count
*
**************************************************************************/
@Nonnegative
public int findDataObjectCount()
throws UnknownPropertyException
{
return MetadataPersistenceSpi.Locator.findPersistenceSpi().findDataObjectCount(this);
}
/***************************************************************************
*
*
**************************************************************************/
@CheckForNull
public Criterion getA()
{
return a;
}
/***************************************************************************
*
*
**************************************************************************/
@CheckForNull
public Criterion getB()
{
return b;
}
/***************************************************************************
*
*
**************************************************************************/
@CheckForNull
public String getCode()
{
return code;
}
/***************************************************************************
*
*
**************************************************************************/
@CheckForNull
public String getPropertySetName()
{
return propertySetName;
}
/***************************************************************************
*
*
**************************************************************************/
@CheckForNull
public Object getObject()
{
return object;
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public Operator getOp()
{
return op;
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public Criterion and (@Nonnull final Criterion a)
{
return op(this, Operator.AND, a);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public Criterion or (@Nonnull final Criterion a)
{
return op(this, Operator.OR, a);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion matches (@Nonnull final PropertyAndValue propertyAndValue)
{
final MetadataProperty property = propertyAndValue.getProperty();
final MetadataPersistenceSpi persistenceSpi = MetadataPersistenceSpi.Locator.findPersistenceSpi();
final String propertySetName = persistenceSpi.findPropertySetNameByBeanClass(property.getItemClass());
final String propertyName = property.getPropertyName();
return equals(propertySetName, propertyName, propertyAndValue.getValue());
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion equals (@CheckForNull final String propertySetName,
@CheckForNull final String propertyName,
@CheckForNull final Object value)
{
return new Criterion(propertySetName, propertyName, Operator.EQUALS, value);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion lt (@Nonnull final String propertySetName,
@Nonnull final String propertyName,
@Nonnull final Object value)
{
return new Criterion(propertySetName, propertyName, Operator.LT, value);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion lte (@Nonnull final String propertySetName,
@Nonnull final String propertyName,
@Nonnull final Object value)
{
return new Criterion(propertySetName, propertyName, Operator.LTE, value);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion gt (@Nonnull final String propertySetName,
@Nonnull final String propertyName,
@Nonnull final Object value)
{
return new Criterion(propertySetName, propertyName, Operator.GT, value);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion gte (@Nonnull final String propertySetName,
@Nonnull final String propertyName,
@Nonnull final Object value)
{
return new Criterion(propertySetName, propertyName, Operator.GTE, value);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion between (@Nonnull final String propertySetName,
@Nonnull final String propertyName,
@Nonnull final Object low,
@Nonnull final Object high)
{
return gte(propertySetName, propertyName, low).and(lte(propertySetName, propertyName, high));
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion between (@Nonnull final MetadataProperty> property,
@Nonnull final Object low,
@Nonnull final Object high)
{
final MetadataComposite propertySet = property.getParent();
final MetadataPersistenceSpi persistenceSpi = MetadataPersistenceSpi.Locator.findPersistenceSpi();
final String propertySetName = persistenceSpi.findPropertySetNameByBeanClass(propertySet.getItemClass());
return Criterion.between(propertySetName, property.getPropertyName(), low, high);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public static Criterion not (@Nonnull final Criterion a)
{
return new Criterion(Operator.NOT, a);
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
private static Criterion op (@Nonnull final Criterion a,
@Nonnull final Operator op,
@Nonnull final Criterion b)
{
return new Criterion(a, op, b);
}
/***************************************************************************
*
*
**************************************************************************/
@Override
@Nonnull
public String toString()
{
if (op == Operator.EQUALS)
{
return String.format("%s.%s=%s", propertySetName, code, object);
}
else
{
return String.format("(%s %s %s)", a, op, b);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy