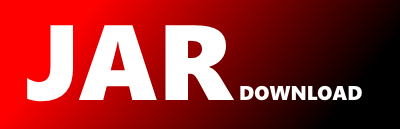
it.tidalwave.metadata.persistence.spi.BeanAccessor Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.persistence.spi;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.util.List;
/*******************************************************************************
*
* This class defines how the Persistence Facility "sees" a bean of metadata.
* By means of BeanAccessor
it is possible to define how data values
* are stored (see {@link convert(String,Object)} and
* {@link convertReverse(String,Object)}) and how property names are encoded
* (for instance, it could be better for TIFF data to store TIFF codes rather
* than Java bean property names, that could be refactored in time).
*
There must be an instance of BeanAccessor
for each type of
* metadata item (e.g. one for TIFF, one for EXIF, one for MakerNote, etc...)
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public interface BeanAccessor
{
/***************************************************************************
*
* Returns the name of the set of properties managed by this class as it is
* seen by the Persistence Facility.
*
* @return the name of the property set
*
**************************************************************************/
@Nonnull
public String getPropertySetName();
/***************************************************************************
*
* Returns a list of names of persistent properties as they are seen by the
* Persistence Facility. This list does not necesarily include all the
* existing properties of the bean; some are decided to be "transient" and
* ignored.
*
* @param bean the bean to inspect
* @return the list of names of persistent properties
*
**************************************************************************/
@Nonnull
public List getPersistentPropertyNames (@Nonnull Bean bean);
/***************************************************************************
*
* Returns true if the given property is persistent.
*
* @param propertyName the property name
* @return true if the property is persistent
*
**************************************************************************/
public boolean isPersistent (@Nonnull String propertyName);
/***************************************************************************
*
* Returns the default type of the given property. Note that some values
* for that property could have a different type - for instance, it's
* typical in the TIFF world to have a field officially labeled as INTEGER
* and yet have values typed SHORT or LONG. Usually it's better to test
* the type of a value by calling the getClass
method on it;
* this method is useful to retrieve the default type when you have a null
* value to manage.
*
* @param propertyName the property name
* @throws UnknownPropertyException if the property type cannot be found
* @return the default property type
*
**************************************************************************/
@Nonnull
public Class> getDefaultPropertyType (@Nonnull String propertyName)
throws UnknownPropertyException;
/***************************************************************************
*
* Reads the given property from the given bean, returning a representation
* suitable for the Persistence Facility.
*
* FIXME: swap arguments for similarity with setProperty
*
* @param bean the bean
* @param propertyName the property name
* @return the property value
*
**************************************************************************/
@CheckForNull
public Object getProperty (@Nonnull String propertyName, @Nonnull Bean bean)
throws UnknownPropertyException;
/***************************************************************************
*
* Writes the given property for the given bean, passing a value whose
* representation comes from the Persistence Facility.
*
* @param bean the bean
* @param propertyName the property name
* @param value the value
*
**************************************************************************/
@Nonnull
public void setProperty (@Nonnull Bean bean,
@Nonnull String propertyName,
@CheckForNull Object value)
throws UnknownPropertyException;
/***************************************************************************
*
* Converts a value into a representation suitable for the Persistence
* Facility.
*
* @param propertyName the property name
* @param value the value
* @return the value representation for the persistence
*
**************************************************************************/
@CheckForNull
public Object convertValueToPersistence (@Nonnull String propertyName,
@CheckForNull Object value)
throws UnknownPropertyException;
/***************************************************************************
*
* Converts a value from the representation given by the Persistence
* Facility.
*
* @param propertyName the property name
* @param value the value from the persistence
* @return the value
*
**************************************************************************/
@CheckForNull
public Object convertValueFromPersistence (@Nonnull String propertyName,
@CheckForNull Object value)
throws UnknownPropertyException;
/***************************************************************************
*
* Converts a propertyName into the code used by the Persistence Facility.
*
* @param propertyName the property name
* @return the Persistence representation
*
**************************************************************************/
@Nonnull
public String convertPropertyNameToPersistence (@Nonnull String propertyName)
throws UnknownPropertyException;
/***************************************************************************
*
* Converts a propertyName from the code used by the Persistence Facility.
*
* @param propertyName the Persistence representation
* @return the property name
*
**************************************************************************/
@Nonnull
public String convertPropertyNameFromPersistence (@Nonnull String propertyName)
throws UnknownPropertyException;
}