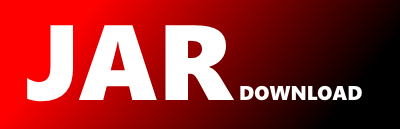
it.tidalwave.metadata.persistence.spi.IntrospectionBeanAccessor Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.persistence.spi;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.beans.IntrospectionException;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.util.Locale;
import org.jdesktop.beansbinding.Property;
import it.tidalwave.beans.FastBeanProperty;
/*******************************************************************************
*
* This class provides support for a {@link BeanAccessor} based on JavaBean
* introspection.
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public abstract class IntrospectionBeanAccessor- extends BeanAccessorSupport
-
{
/***************************************************************************
*
* Creates this object passing the class of the represented item and the name
* of the property set.
*
* @param itemClass the class of the represented item
* @param propertySetName the name of the property set
*
**************************************************************************/
public IntrospectionBeanAccessor (@Nonnull final Class extends Item> itemClass,
@Nonnull final String propertySetName)
{
super(itemClass, propertySetName);
}
/***************************************************************************
*
* {@inheritDoc}.
*
**************************************************************************/
@Nonnull
public Class> getDefaultPropertyType (@Nonnull final String propertyName)
throws UnknownPropertyException
{
final String xxx = propertyName.substring(0, 1).toUpperCase(Locale.getDefault()) + propertyName.substring(1);
try
{
final String getterName = "get" + xxx;
return getItemClass().getMethod(getterName).getReturnType();
}
catch (NoSuchMethodException e)
{
try
{
final String getterName = "is" + xxx;
return getItemClass().getMethod(getterName).getReturnType();
}
catch (NoSuchMethodException e2)
{
throw new UnknownPropertyException(getItemClass(), propertyName);
}
}
}
/***************************************************************************
*
* {@inheritDoc}.
*
**************************************************************************/
@CheckForNull
public Object getProperty (@Nonnull final String propertyName, @Nonnull final Item bean)
throws UnknownPropertyException
{
final Property property = FastBeanProperty.create(propertyName);
return convertValueToPersistence(propertyName, property.getValue(bean));
}
/***************************************************************************
*
* {@inheritDoc}.
*
**************************************************************************/
public void setProperty (@Nonnull final Item bean,
@Nonnull final String propertyName,
@CheckForNull final Object value)
throws UnknownPropertyException
{
final Property property = FastBeanProperty.create(propertyName);
property.setValue(bean, convertValueFromPersistence(propertyName, value));
}
/***************************************************************************
*
* {@inheritDoc}.
*
**************************************************************************/
@Override
@Nonnull
public String toString()
{
return String.format("IntrospectionBeanAccessor[%s]", getPropertySetName());
}
/***************************************************************************
*
**************************************************************************/
@Nonnull
// FIXME the parameter is unneeded, use itemClass
// FIXME compute the thing only the first time is called, then cache it
protected PropertyDescriptor[] findPropertyDescriptors (@Nonnull final Object item)
throws IntrospectionException
{
return Introspector.getBeanInfo(item.getClass()).getPropertyDescriptors();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy